PySpark
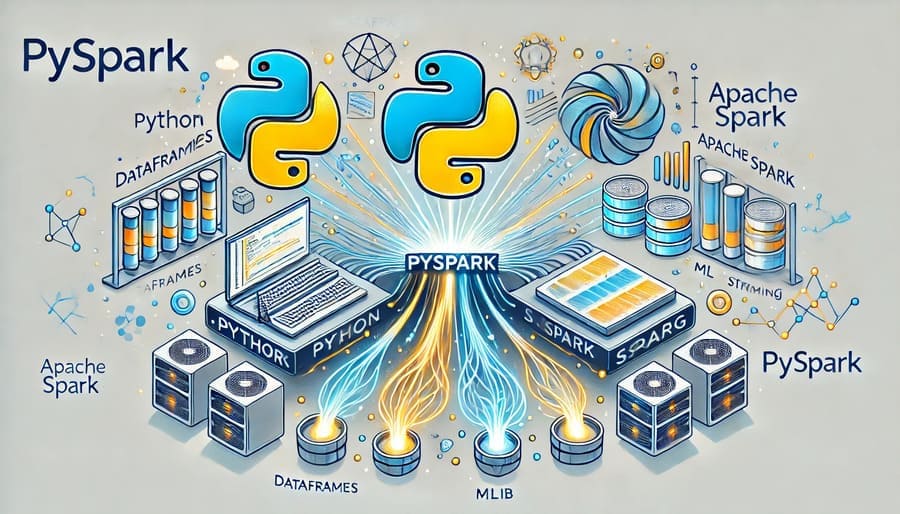
In today’s data-driven world, processing massive datasets efficiently has become a critical challenge for organizations of all sizes. Enter PySpark—a powerful Python API for Apache Spark that bridges the gap between scalable distributed computing and Python’s accessibility. This comprehensive guide explores PySpark’s capabilities, advantages, and practical applications for data engineers and data scientists.
PySpark is the Python API for Apache Spark, a unified analytics engine designed for large-scale data processing. Created to make Spark accessible to Python developers, PySpark combines Spark’s distributed computing power with Python’s simplicity and rich ecosystem of data science libraries.
# Simple PySpark example
from pyspark.sql import SparkSession
# Initialize Spark session
spark = SparkSession.builder \
.appName("PySpark Introduction") \
.getOrCreate()
# Read data from CSV
df = spark.read.csv("s3://my-bucket/large-dataset.csv", header=True, inferSchema=True)
# Perform transformations
result = df.filter(df.age > 25) \
.groupBy("department") \
.agg({"salary": "avg", "age": "max"}) \
.orderBy("department")
# Show results
result.show()
PySpark’s architecture consists of several key components that work together to enable distributed data processing:
The entry point to any PySpark functionality, SparkSession provides a unified interface to all Spark operations and data.
from pyspark.sql import SparkSession
# Create a SparkSession with specific configurations
spark = SparkSession.builder \
.appName("MyApplication") \
.config("spark.executor.memory", "4g") \
.config("spark.executor.cores", "4") \
.config("spark.driver.memory", "2g") \
.master("yarn") \
.getOrCreate()
RDDs are PySpark’s fundamental data structure—immutable, distributed collections of objects that can be processed in parallel.
# Create an RDD from a Python list
data = [1, 2, 3, 4, 5]
rdd = spark.sparkContext.parallelize(data)
# Apply transformations
squared = rdd.map(lambda x: x * x)
# Collect results
result = squared.collect()
print(result) # [1, 4, 9, 16, 25]
Building on RDDs, DataFrames provide a more structured, tabular data abstraction similar to pandas DataFrames but distributed across a cluster.
# Create a DataFrame from a CSV file
df = spark.read.csv("customer_data.csv", header=True, inferSchema=True)
# Display schema
df.printSchema()
# Basic operations
df.select("name", "age").show(5)
df.filter(df.age > 30).show(5)
df.groupBy("department").count().show()
PySpark includes Spark SQL, allowing you to execute SQL queries against your data.
# Register a DataFrame as a temporary view
df.createOrReplaceTempView("employees")
# Run SQL query
result = spark.sql("""
SELECT department, AVG(salary) as avg_salary
FROM employees
WHERE age > 30
GROUP BY department
HAVING AVG(salary) > 60000
ORDER BY avg_salary DESC
""")
result.show()
PySpark’s MLlib provides scalable machine learning algorithms optimized for distributed computing.
from pyspark.ml.feature import VectorAssembler
from pyspark.ml.classification import RandomForestClassifier
from pyspark.ml.evaluation import MulticlassClassificationEvaluator
# Prepare features
assembler = VectorAssembler(
inputCols=["age", "income", "education_years", "credit_score"],
outputCol="features"
)
training_data = assembler.transform(df)
# Split data
train_data, test_data = training_data.randomSplit([0.7, 0.3], seed=42)
# Train model
rf = RandomForestClassifier(
labelCol="risk_category",
featuresCol="features",
numTrees=100
)
model = rf.fit(train_data)
# Make predictions
predictions = model.transform(test_data)
# Evaluate model
evaluator = MulticlassClassificationEvaluator(
labelCol="risk_category",
predictionCol="prediction",
metricName="accuracy"
)
accuracy = evaluator.evaluate(predictions)
print(f"Model Accuracy: {accuracy}")
PySpark offers Structured Streaming for processing real-time data streams with the same DataFrame API used for batch processing.
# Create streaming DataFrame from a data source
streaming_df = spark \
.readStream \
.format("kafka") \
.option("kafka.bootstrap.servers", "broker1:9092,broker2:9092") \
.option("subscribe", "sensor_data") \
.load()
# Process the stream
processed_stream = streaming_df \
.selectExpr("CAST(key AS STRING)", "CAST(value AS STRING)") \
.select(from_json("value", schema).alias("data")) \
.select("data.*") \
.filter("temperature > 80") \
.groupBy(window("timestamp", "10 minutes"), "sensor_id") \
.agg({"temperature": "avg"})
# Write the output to a sink
query = processed_stream \
.writeStream \
.outputMode("complete") \
.format("console") \
.start()
query.awaitTermination()
One of PySpark’s greatest strengths is its ability to scale from a single machine to hundreds of nodes with minimal code changes.
# Local mode for development
spark_local = SparkSession.builder \
.master("local[*]") \
.appName("Local Development") \
.getOrCreate()
# Same code runs on a cluster
spark_cluster = SparkSession.builder \
.master("yarn") \
.appName("Production Application") \
.config("spark.executor.instances", "20") \
.getOrCreate()
PySpark integrates with Python’s rich data science libraries, enabling a powerful combination of distributed computing and specialized analytical tools.
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from pyspark.ml.feature import PCA
from pyspark.ml.clustering import KMeans
# Process data with PySpark
pca = PCA(k=2, inputCol="features", outputCol="pca_features")
kmeans = KMeans(k=5, featuresCol="pca_features")
# Convert to pandas for visualization
pandas_df = result.toPandas()
# Create a scatter plot with matplotlib
plt.figure(figsize=(10, 8))
for cluster in range(5):
cluster_data = pandas_df[pandas_df['prediction'] == cluster]
plt.scatter(
cluster_data['pca_features'].apply(lambda x: x[0]),
cluster_data['pca_features'].apply(lambda x: x[1]),
label=f'Cluster {cluster}'
)
plt.title('Customer Segmentation')
plt.xlabel('PCA Component 1')
plt.ylabel('PCA Component 2')
plt.legend()
plt.savefig('customer_segments.png')
PySpark inherits Spark’s robust fault tolerance mechanisms, ensuring reliable processing even when individual nodes fail.
# Configure checkpointing for fault tolerance
spark.sparkContext.setCheckpointDir("hdfs://namenode:8020/spark-checkpoints")
# Use checkpointing for complex operations
complex_rdd = initial_rdd.map(step1).filter(filter_condition).checkpoint()
result = complex_rdd.groupByKey().mapValues(complex_aggregation)
PySpark leverages Spark’s optimization engine for efficient distributed computation.
# Enable adaptive query execution
spark.conf.set("spark.sql.adaptive.enabled", "true")
# Partition data for better parallelism
df = df.repartition(100, "customer_id")
# Cache frequently used DataFrames
df.cache()
# Use broadcast joins for small-large table joins
from pyspark.sql.functions import broadcast
result = df1.join(broadcast(small_df), "join_key")
PySpark excels at Extract, Transform, Load (ETL) operations on massive datasets:
# Extract data from multiple sources
customer_df = spark.read.parquet("s3://data-lake/customers/")
orders_df = spark.read.jdbc(
url="jdbc:postgresql://warehouse.company.com:5432/orders",
table="orders",
properties={"user": "etl_user", "password": "secret", "driver": "org.postgresql.Driver"}
)
web_events = spark.read.json("s3://data-lake/web-events/")
# Transform data
from pyspark.sql.functions import col, datediff, current_date, when, expr
# Clean and standardize
customers_clean = customer_df.dropDuplicates(["customer_id"]) \
.filter(col("email").isNotNull()) \
.withColumn("customer_segment",
when(col("total_spend") > 1000, "high_value")
.when(col("total_spend") > 500, "medium_value")
.otherwise("low_value"))
# Join datasets
customer_orders = customers_clean.join(
orders_df,
"customer_id",
"left"
)
# Aggregate
customer_metrics = customer_orders.groupBy("customer_id", "customer_segment") \
.agg(
count("order_id").alias("order_count"),
sum("order_total").alias("lifetime_value"),
avg("order_total").alias("avg_order_value"),
datediff(current_date(), max("order_date")).alias("days_since_last_order")
)
# Load data to destination
customer_metrics.write \
.mode("overwrite") \
.partitionBy("customer_segment") \
.parquet("s3://analytics-warehouse/customer_metrics/")
PySpark’s MLlib enables training complex machine learning models on massive datasets:
from pyspark.ml import Pipeline
from pyspark.ml.feature import StringIndexer, OneHotEncoder, VectorAssembler
from pyspark.ml.classification import GBTClassifier
from pyspark.ml.tuning import CrossValidator, ParamGridBuilder
from pyspark.ml.evaluation import BinaryClassificationEvaluator
# Prepare data
categorical_cols = ["education", "marital_status", "occupation"]
numeric_cols = ["age", "hours_per_week", "capital_gain", "capital_loss"]
# Feature engineering pipeline
stages = []
# Handle categorical features
for col in categorical_cols:
# Convert string to category index
string_indexer = StringIndexer(inputCol=col, outputCol=f"{col}_index")
# Convert category index to one-hot encoding
encoder = OneHotEncoder(inputCols=[f"{col}_index"], outputCols=[f"{col}_encoded"])
stages += [string_indexer, encoder]
# Combine all features into vector
assembler_inputs = [f"{col}_encoded" for col in categorical_cols] + numeric_cols
assembler = VectorAssembler(inputCols=assembler_inputs, outputCol="features")
stages += [assembler]
# Add classifier to the pipeline
gbt = GBTClassifier(labelCol="income_label", featuresCol="features", maxIter=10)
stages += [gbt]
# Create and fit the pipeline
pipeline = Pipeline(stages=stages)
# Split data
train, test = df.randomSplit([0.7, 0.3], seed=42)
# Parameter grid for cross-validation
paramGrid = ParamGridBuilder() \
.addGrid(gbt.maxDepth, [5, 10, 15]) \
.addGrid(gbt.maxIter, [10, 20]) \
.addGrid(gbt.stepSize, [0.01, 0.1]) \
.build()
# Evaluator
evaluator = BinaryClassificationEvaluator(
labelCol="income_label",
metricName="areaUnderROC"
)
# Cross-validation
cv = CrossValidator(
estimator=pipeline,
estimatorParamMaps=paramGrid,
evaluator=evaluator,
numFolds=3
)
# Train with cross-validation
cv_model = cv.fit(train)
# Make predictions
predictions = cv_model.transform(test)
# Evaluate performance
auc = evaluator.evaluate(predictions)
print(f"Area under ROC curve: {auc}")
# Save model for deployment
cv_model.write().overwrite().save("s3://models/income_predictor")
PySpark Structured Streaming enables complex real-time analytics:
from pyspark.sql.functions import from_json, window, col, count, avg
from pyspark.sql.types import StructType, StructField, StringType, TimestampType, DoubleType
# Define schema for incoming JSON data
schema = StructType([
StructField("device_id", StringType(), True),
StructField("timestamp", TimestampType(), True),
StructField("temperature", DoubleType(), True),
StructField("humidity", DoubleType(), True),
StructField("pressure", DoubleType(), True),
StructField("location", StringType(), True)
])
# Read from Kafka stream
streaming_df = spark \
.readStream \
.format("kafka") \
.option("kafka.bootstrap.servers", "kafka:9092") \
.option("subscribe", "iot_sensors") \
.option("startingOffsets", "latest") \
.load()
# Parse JSON data
parsed_df = streaming_df \
.select(from_json(col("value").cast("string"), schema).alias("data")) \
.select("data.*")
# Detect anomalies in real-time
anomalies = parsed_df \
.withWatermark("timestamp", "1 minute") \
.filter("temperature > 100 OR temperature < 0") \
.select("device_id", "timestamp", "temperature", "location")
# Calculate moving averages by location
avg_metrics = parsed_df \
.withWatermark("timestamp", "10 minutes") \
.groupBy(
window(col("timestamp"), "5 minutes", "1 minute"),
col("location")
) \
.agg(
count("*").alias("event_count"),
avg("temperature").alias("avg_temp"),
avg("humidity").alias("avg_humidity")
)
# Write anomalies to alert system
anomaly_query = anomalies \
.writeStream \
.outputMode("append") \
.format("kafka") \
.option("kafka.bootstrap.servers", "kafka:9092") \
.option("topic", "temperature_alerts") \
.option("checkpointLocation", "hdfs://checkpoint/anomalies") \
.start()
# Write aggregates to dashboard
dashboard_query = avg_metrics \
.writeStream \
.outputMode("complete") \
.format("memory") \
.queryName("metrics_for_dashboard") \
.start()
# Keep the streaming application running
spark.streams.awaitAnyTermination()
Create custom functions to extend PySpark’s capabilities:
from pyspark.sql.functions import udf
from pyspark.sql.types import FloatType, ArrayType, StringType
import re
# Python function to clean text
def clean_text(text):
if text is None:
return ""
# Remove special characters, lowercase
cleaned = re.sub(r'[^\w\s]', '', text.lower())
# Remove extra whitespace
cleaned = re.sub(r'\s+', ' ', cleaned).strip()
return cleaned
# Register as UDF
clean_text_udf = udf(clean_text, StringType())
# Apply to DataFrame
cleaned_df = df.withColumn("clean_description", clean_text_udf(col("product_description")))
# More complex UDF with multiple inputs
def calculate_risk_score(income, debt, credit_score):
if credit_score > 700:
base_score = 80
elif credit_score > 650:
base_score = 60
else:
base_score = 40
# Calculate debt-to-income ratio
if income > 0:
dti = (debt / income) * 100
if dti < 20:
return base_score + 15
elif dti < 40:
return base_score
else:
return max(20, base_score - 20)
else:
return 0
# Register complex UDF
risk_score_udf = udf(calculate_risk_score, FloatType())
# Apply to DataFrame
risk_df = customer_df.withColumn(
"risk_score",
risk_score_udf(col("annual_income"), col("total_debt"), col("credit_score"))
)
Tune PySpark applications for maximum performance:
# Memory tuning
spark.conf.set("spark.memory.fraction", "0.8")
spark.conf.set("spark.memory.storageFraction", "0.4")
# Serialization
spark.conf.set("spark.serializer", "org.apache.spark.serializer.KryoSerializer")
spark.conf.set("spark.kryo.registrationRequired", "false")
# SQL optimization
spark.conf.set("spark.sql.adaptive.enabled", "true")
spark.conf.set("spark.sql.adaptive.skewJoin.enabled", "true")
spark.conf.set("spark.sql.adaptive.coalescePartitions.enabled", "true")
# Handle skewed data
from pyspark.sql.functions import spark_partition_id
# Check partition skew
df.groupBy(spark_partition_id()).count().show()
# Repartition data based on distribution
df_balanced = df.repartitionByRange(200, "customer_id")
# Use salting technique for skewed joins
from pyspark.sql.functions import monotonically_increasing_id, rand
def salted_join(skewed_df, normal_df, join_key, num_salts=10):
# Add a salt column to both DataFrames
skewed_with_salt = skewed_df.withColumn("salt", (rand() * num_salts).cast("int"))
# Explode the normal DataFrame with salts
normal_exploded = normal_df.withColumn("temp_key", monotonically_increasing_id())
salts = spark.range(0, num_salts).toDF("salt")
normal_with_salts = normal_exploded.crossJoin(salts)
# Join on key and salt
joined = skewed_with_salt.join(
normal_with_salts,
(skewed_with_salt[join_key] == normal_with_salts[join_key]) &
(skewed_with_salt["salt"] == normal_with_salts["salt"])
)
# Select original columns
columns_to_select = [c for c in joined.columns if c != "salt" and c != "temp_key"]
return joined.select(*columns_to_select)
# Use the salted join for skewed keys
balanced_join = salted_join(customers, transactions, "customer_id", 20)
Combine PySpark with deep learning frameworks for distributed training:
# Using Deep Learning with PySpark
from pyspark.sql.functions import pandas_udf
from pyspark.sql.types import ArrayType, FloatType
import tensorflow as tf
import numpy as np
# Define model-building function
def build_model(input_dim):
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(input_dim,)),
tf.keras.layers.Dense(32, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
return model
# Define Pandas UDF for distributed prediction
@pandas_udf(ArrayType(FloatType()))
def predict_batch(features_pandas):
# Convert pandas series to numpy arrays
features_np = np.array(features_pandas.tolist())
# Load the pre-trained model
loaded_model = tf.keras.models.load_model("s3://models/tf_binary_classifier")
# Make predictions
predictions = loaded_model.predict(features_np)
# Return as list of floats
return pd.Series(predictions.flatten().tolist())
# Apply the model to PySpark DataFrame
predictions_df = feature_df.withColumn("prediction_score", predict_batch(col("features")))
Establish an efficient development workflow:
# Local development setup
import findspark
findspark.init()
import pyspark
from pyspark.sql import SparkSession
# Create a local session with limited resources
spark = SparkSession.builder \
.master("local[2]") \
.appName("Local Development") \
.config("spark.executor.memory", "2g") \
.config("spark.driver.memory", "2g") \
.config("spark.ui.port", "4050") \
.getOrCreate()
# Work with sample data
sample_df = spark.read.parquet("sample_data.parquet")
# Profile your application
with SparkProfiler("profile_results.json"):
result = complex_transformation(sample_df)
# Unit testing with PyTest
def test_transformation():
# Create test data
test_data = [("A", 1), ("B", 2), ("A", 3)]
schema = ["key", "value"]
test_df = spark.createDataFrame(test_data, schema)
# Apply transformation
result = group_and_sum(test_df)
# Convert to pandas for assertions
pd_result = result.toPandas()
assert len(pd_result) == 2
assert pd_result[pd_result.key == "A"].sum_value.iloc[0] == 4
Prepare PySpark applications for production:
# Package as Python application
# requirements.txt
# pyspark==3.3.0
# boto3==1.24.59
# delta-spark==2.1.0
# main.py
from pyspark.sql import SparkSession
import argparse
def create_spark_session(app_name, configs=None):
"""Create a configured Spark session."""
builder = SparkSession.builder.appName(app_name)
# Apply additional configurations
if configs:
for key, value in configs.items():
builder = builder.config(key, value)
return builder.getOrCreate()
def run_etl(date, source_path, target_path):
"""Main ETL logic."""
spark = create_spark_session("Production ETL")
# Log start
spark.sparkContext.setJobDescription(f"ETL processing for {date}")
try:
# ETL logic here
# ...
# Write outputs
# ...
return "SUCCESS"
except Exception as e:
spark.sparkContext.setJobGroup("error_handling", f"Handling error: {str(e)}")
# Error handling logic
# ...
return f"FAILED: {str(e)}"
finally:
spark.stop()
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument("--date", required=True, help="Processing date (YYYY-MM-DD)")
parser.add_argument("--source", required=True, help="Source data path")
parser.add_argument("--target", required=True, help="Target data path")
args = parser.parse_args()
result = run_etl(args.date, args.source, args.target)
print(f"Job completed with status: {result}")
# Running in production
# spark-submit --master yarn \
# --deploy-mode cluster \
# --conf spark.executor.memory=4g \
# --conf spark.executor.cores=2 \
# --conf spark.dynamicAllocation.enabled=true \
# --conf spark.dynamicAllocation.minExecutors=5 \
# --conf spark.dynamicAllocation.maxExecutors=20 \
# --py-files dependencies.zip \
# main.py --date 2023-05-15 --source s3://data-lake/raw/ --target s3://warehouse/processed/
Implement robust monitoring for PySpark applications:
# Add logging
import logging
from pyspark.sql import SparkSession
# Configure logging
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
logger = logging.getLogger("etl_application")
# Create session with listener for metrics
spark = SparkSession.builder \
.appName("Monitored Application") \
.config("spark.extraListeners", "com.example.SparkMetricsListener") \
.getOrCreate()
logger.info("Starting ETL process")
try:
# Process data with checkpoints
logger.info("Loading source data")
df = spark.read.parquet("s3://data/source/")
# Log record count
count = df.count()
logger.info(f"Loaded {count} records")
# Add checkpoints for complex operations
logger.info("Starting transformation phase")
df.write.mode("overwrite").saveAsTable("checkpoint_1")
# Continue processing
transformed = spark.table("checkpoint_1") \
.transform(complex_operation_1) \
.transform(complex_operation_2)
# Log statistics for data quality
null_counts = {col: transformed.filter(col(col).isNull()).count()
for col in transformed.columns}
logger.info(f"Null value counts: {null_counts}")
# Save results
logger.info("Writing results")
transformed.write.mode("overwrite").parquet("s3://data/target/")
logger.info("ETL process completed successfully")
except Exception as e:
logger.error(f"ETL process failed: {str(e)}", exc_info=True)
raise
As data volumes continue to grow and analytics requirements become more complex, PySpark is evolving to meet these challenges:
PySpark is becoming more tightly integrated with deep learning frameworks, enabling end-to-end AI pipelines from data preparation to model deployment.
With the growth of cloud computing, PySpark is enhancing its integration with cloud-native services for storage, security, and resource management.
Ongoing optimizations in Apache Spark’s execution engine continue to improve PySpark’s performance, particularly for real-time and streaming applications.
Efforts to simplify PySpark’s API and improve error messages make the framework more accessible to data scientists without deep distributed computing knowledge.
PySpark has become an essential tool in the modern data engineer’s toolkit, offering the perfect balance between Python’s accessibility and Apache Spark’s distributed computing power. From ETL pipelines and machine learning to real-time analytics, PySpark enables organizations to process massive datasets efficiently while leveraging Python’s rich ecosystem.
Whether you’re a data engineer building robust data pipelines, a data scientist training models on large datasets, or an analyst exploring massive data lakes, PySpark provides the tools to handle data at any scale with the familiarity of Python syntax.
As big data continues to grow in importance across industries, mastering PySpark opens up opportunities to solve the most challenging data problems with elegant, scalable solutions. By combining Python’s simplicity with Spark’s distributed computing capabilities, PySpark truly offers the best of both worlds for modern data processing.
#PySpark #ApacheSpark #BigData #DataEngineering #DistributedComputing #DataScience #PythonProgramming #MachineLearning #ETL #DataProcessing #DataAnalytics #SparkSQL #StreamProcessing #ParallelComputing #DataPipelines #ScalableComputing #BigDataAnalytics #CloudComputing #RealTimeAnalytics #DataTransformation