NumPy
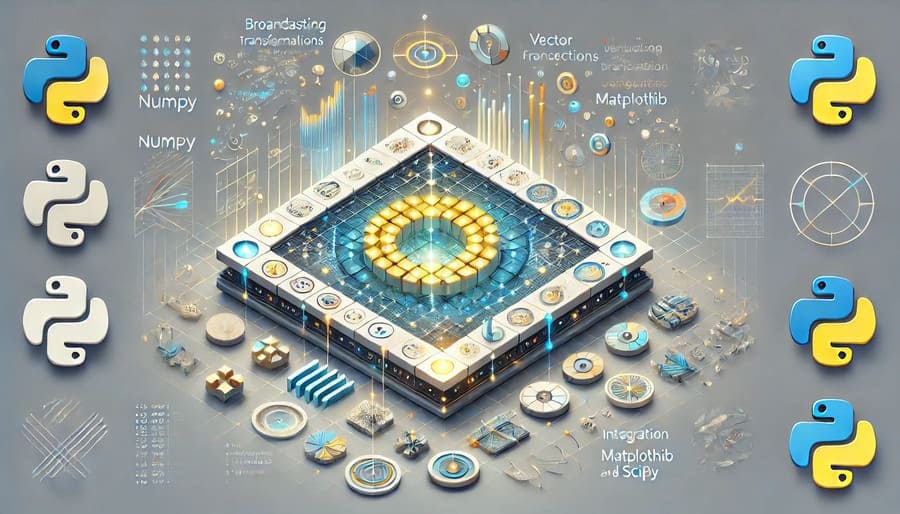
In the vast ecosystem of Python libraries, NumPy stands as one of the most fundamental and revolutionary tools for scientific computing. Short for “Numerical Python,” NumPy has transformed how researchers, data scientists, and engineers work with numerical data, making Python a viable alternative to specialized languages like MATLAB and R. This comprehensive guide explores NumPy’s capabilities, advantages, and practical applications that have made it the cornerstone of Python’s scientific computing stack.
NumPy is an open-source Python library that provides support for large, multi-dimensional arrays and matrices, along with a collection of high-level mathematical functions to operate on these arrays. Created by Travis Oliphant in 2005, NumPy evolved from the earlier Numeric and Numarray libraries, unifying them into a powerful tool that would become the foundation for Python’s scientific computing ecosystem.
import numpy as np
# Create a 2D array
arr = np.array([[1, 2, 3], [4, 5, 6]])
print(arr)
# Output:
# [[1 2 3]
# [4 5 6]]
# Array properties
print(f"Shape: {arr.shape}") # Output: Shape: (2, 3)
print(f"Dimensions: {arr.ndim}") # Output: Dimensions: 2
print(f"Data type: {arr.dtype}") # Output: Data type: int64
At the heart of NumPy is the ndarray
(n-dimensional array) — a homogeneous, fixed-size multidimensional array that offers significant advantages over Python’s built-in data structures:
# Creating arrays
zeros = np.zeros((3, 4)) # 3x4 array of zeros
ones = np.ones((2, 3, 4)) # 2x3x4 array of ones
range_array = np.arange(0, 10, 2) # [0, 2, 4, 6, 8]
linspace = np.linspace(0, 1, 5) # 5 evenly spaced values from 0 to 1
# Array from existing data
list_array = np.array([1, 2, 3, 4])
nested_array = np.array([[1, 2], [3, 4], [5, 6]])
Unlike Python lists, NumPy arrays store data in contiguous memory blocks, which enables:
- Memory efficiency: Arrays use less memory than equivalent Python lists
- Faster access: Contiguous memory allows for quick data retrieval
- Vectorization: Operations apply to entire arrays without explicit loops
- Optimized C implementation: Core operations are implemented in C for speed
import time
import numpy as np
# Compare performance: Python list vs. NumPy array
size = 10000000
# Python list operation
python_list = list(range(size))
start = time.time()
python_result = [x * 2 for x in python_list]
python_time = time.time() - start
# NumPy operation
numpy_array = np.arange(size)
start = time.time()
numpy_result = numpy_array * 2
numpy_time = time.time() - start
print(f"Python list time: {python_time:.6f} seconds")
print(f"NumPy array time: {numpy_time:.6f} seconds")
print(f"NumPy is {python_time/numpy_time:.1f}x faster")
Broadcasting is one of NumPy’s most powerful features, allowing operations between arrays of different dimensions:
# Adding a scalar to an array
arr = np.array([1, 2, 3, 4])
result = arr + 10 # [11, 12, 13, 14]
# Broadcasting with different shapes
x = np.array([[1, 2, 3], [4, 5, 6]]) # 2x3 array
y = np.array([10, 20, 30]) # 1D array
result = x + y # Result: [[11, 22, 33], [14, 25, 36]]
NumPy provides a rich set of functions for reshaping, combining, and splitting arrays:
# Reshaping arrays
arr = np.arange(12)
reshaped = arr.reshape(3, 4) # Convert to 3x4 array
# Combining arrays
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
stacked_v = np.vstack((a, b)) # Vertical stack: [[1, 2, 3], [4, 5, 6]]
stacked_h = np.hstack((a, b)) # Horizontal stack: [1, 2, 3, 4, 5, 6]
concatenated = np.concatenate((a, b)) # [1, 2, 3, 4, 5, 6]
# Splitting arrays
arr = np.arange(12).reshape(3, 4)
split_h = np.hsplit(arr, 2) # Split into 2 arrays horizontally
split_v = np.vsplit(arr, 3) # Split into 3 arrays vertically
NumPy excels at mathematical operations, from basic arithmetic to complex linear algebra:
# Basic arithmetic
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
addition = a + b # [5, 7, 9]
multiplication = a * b # [4, 10, 18]
power = a ** 2 # [1, 4, 9]
# Statistical operations
arr = np.array([1, 2, 3, 4, 5])
mean = np.mean(arr) # 3.0
median = np.median(arr) # 3.0
std_dev = np.std(arr) # 1.4142...
# Linear algebra
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
matrix_product = np.matmul(a, b) # or a @ b in Python 3.5+
# [[19, 22],
# [43, 50]]
# Eigenvalues and eigenvectors
eigenvalues, eigenvectors = np.linalg.eig(a)
NumPy offers flexible ways to access and modify array elements:
# Basic indexing
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
element = arr[1, 2] # Value at row 1, column 2: 6
# Slicing
row = arr[1, :] # Second row: [4, 5, 6]
column = arr[:, 1] # Second column: [2, 5, 8]
sub_matrix = arr[0:2, 1:3] # 2x2 sub-matrix: [[2, 3], [5, 6]]
# Boolean indexing
mask = arr > 5
filtered = arr[mask] # [6, 7, 8, 9]
# Fancy indexing
selected_rows = arr[[0, 2]] # First and third rows
selected_elements = arr[[0, 1, 2], [1, 2, 0]] # Elements at (0,1), (1,2), (2,0)
NumPy’s random module provides comprehensive tools for generating random data:
# Generate random numbers
uniform = np.random.random(5) # 5 random floats between 0 and 1
normal = np.random.normal(0, 1, 5) # 5 samples from normal distribution
integers = np.random.randint(1, 10, 5) # 5 random integers between 1 and 9
# Set seed for reproducibility
np.random.seed(42)
random_data = np.random.random(3) # Always the same "random" values
# Generate random matrices
random_matrix = np.random.random((3, 4)) # 3x4 matrix of random values
NumPy forms the foundation of the Python data science stack:
# Feature scaling example for machine learning
data = np.array([
[73, 67, 43],
[91, 88, 64],
[87, 134, 58],
[102, 43, 37],
[69, 96, 70]
])
# Standardize each feature (column)
mean = np.mean(data, axis=0)
std = np.std(data, axis=0)
standardized_data = (data - mean) / std
print("Standardized data:")
print(standardized_data)
NumPy’s array structure is perfect for representing and manipulating images:
import numpy as np
from PIL import Image
# Load an image into a NumPy array
img = np.array(Image.open('example.jpg'))
print(f"Image shape: {img.shape}") # (height, width, channels)
# Simple image operations
grayscale = np.mean(img, axis=2).astype(np.uint8) # Convert to grayscale
flipped = np.fliplr(img) # Flip horizontally
rotated = np.rot90(img) # Rotate 90 degrees
# Save processed image
Image.fromarray(grayscale).save('grayscale.jpg')
NumPy enables complex scientific simulations and computations:
# Simple wave propagation simulation
import numpy as np
import matplotlib.pyplot as plt
# Create a grid
x = np.linspace(-10, 10, 500)
y = np.linspace(-10, 10, 500)
X, Y = np.meshgrid(x, y)
# Calculate wave function at time t
def wave(X, Y, t):
r = np.sqrt(X**2 + Y**2)
return np.sin(r - t) / (r + 1)
# Generate wave at time t=0
Z = wave(X, Y, 0)
# Visualize the wave
plt.figure(figsize=(10, 8))
plt.imshow(Z, cmap='viridis')
plt.colorbar(label='Amplitude')
plt.title('2D Wave Simulation')
plt.savefig('wave_simulation.png')
NumPy is extensively used in quantitative finance:
# Monte Carlo simulation for stock price prediction
def monte_carlo_stock_price(S0, mu, sigma, T, N, simulations):
"""
S0: Initial stock price
mu: Expected return
sigma: Volatility
T: Time period (in years)
N: Number of time steps
simulations: Number of simulation paths
"""
dt = T/N
paths = np.zeros((simulations, N+1))
paths[:, 0] = S0
for t in range(1, N+1):
rand = np.random.standard_normal(simulations)
paths[:, t] = paths[:, t-1] * np.exp((mu - 0.5 * sigma**2) * dt +
sigma * np.sqrt(dt) * rand)
return paths
# Simulate stock paths
S0 = 100 # Initial stock price
mu = 0.09 # Annual expected return
sigma = 0.2 # Annual volatility
T = 1 # Time period (1 year)
N = 252 # Trading days in a year
simulations = 1000 # Number of simulation paths
stock_paths = monte_carlo_stock_price(S0, mu, sigma, T, N, simulations)
# Calculate statistics
final_prices = stock_paths[:, -1]
mean_price = np.mean(final_prices)
price_95ci = np.percentile(final_prices, [2.5, 97.5])
print(f"Expected stock price after {T} year: ${mean_price:.2f}")
print(f"95% confidence interval: ${price_95ci[0]:.2f} to ${price_95ci[1]:.2f}")
NumPy’s universal functions operate element-wise on arrays with optimized performance:
# Built-in ufuncs
a = np.arange(1, 6)
exp_values = np.exp(a) # Exponential: [e^1, e^2, e^3, e^4, e^5]
log_values = np.log(a) # Natural logarithm
sin_values = np.sin(a) # Sine function
# Creating custom ufuncs
def custom_sigmoid(x):
return 1 / (1 + np.exp(-x))
sigmoid_values = custom_sigmoid(a)
NumPy can handle heterogeneous data with structured arrays:
# Define a structured array for customer data
customer_dtype = np.dtype([
('name', 'U30'),
('age', 'i4'),
('purchase_amount', 'f8'),
('member', 'bool')
])
customers = np.array([
('John Doe', 34, 199.95, True),
('Jane Smith', 28, 349.50, False),
('Bob Johnson', 42, 149.99, True)
], dtype=customer_dtype)
# Access by field
names = customers['name']
ages = customers['age']
# Filter by conditions
premium_customers = customers[customers['purchase_amount'] > 200]
NumPy provides tools to manage memory and create array views without copying data:
# Create a large array
large_array = np.random.random((10000, 10000))
print(f"Memory usage: {large_array.nbytes / 1e6} MB")
# Create a view (no data is copied)
view = large_array.view()
# Slice creates a view, not a copy
slice_view = large_array[:100, :100]
# Explicit copy when needed
array_copy = large_array.copy()
# Check if array owns its data
print(f"View owns data: {view.flags.owndata}")
print(f"Copy owns data: {array_copy.flags.owndata}")
For dealing with missing or invalid data, NumPy offers masked arrays:
from numpy import ma
# Create a masked array
data = np.array([1, 2, -999, 4, -999, 6])
masked_data = ma.masked_array(data, mask=data==-999)
# Operations automatically skip masked values
mean = ma.mean(masked_data) # Mean of [1, 2, 4, 6]
print(f"Mean (ignoring missing values): {mean}")
NumPy’s true power comes from its integration with the broader Python scientific ecosystem:
# NumPy with Pandas
import pandas as pd
numpy_data = np.random.randint(0, 100, (5, 3))
df = pd.DataFrame(numpy_data, columns=['A', 'B', 'C'])
# NumPy with Matplotlib
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title('Sine Wave')
# NumPy with SciPy
from scipy import stats
data = np.random.normal(0, 1, 1000)
kde = stats.gaussian_kde(data)
x_values = np.linspace(-3, 3, 100)
plt.plot(x_values, kde(x_values))
plt.title('Kernel Density Estimation')
For even more performance, NumPy arrays can be used with GPU-accelerated libraries:
# Example with CuPy (NVIDIA GPU acceleration)
# pip install cupy
import cupy as cp
# Create GPU array
gpu_array = cp.array([1, 2, 3, 4, 5])
# Perform calculation on GPU
result = cp.sin(gpu_array) + cp.cos(gpu_array)
# Transfer back to CPU if needed
cpu_result = cp.asnumpy(result)
To get the most out of NumPy’s performance capabilities:
# Vectorize operations instead of using loops
# Bad (slow):
result = np.zeros(1000)
for i in range(1000):
result[i] = i**2
# Good (fast):
result = np.arange(1000)**2
# Pre-allocate arrays
# Bad:
result = np.array([])
for i in range(1000):
result = np.append(result, i**2) # Creates a new array each time
# Good:
result = np.zeros(1000)
for i in range(1000):
result[i] = i**2
# Best:
result = np.arange(1000)**2
Working with large datasets requires careful memory management:
# Use appropriate dtypes to save memory
small_integers = np.arange(1000, dtype=np.int8) # Uses 1 byte per element
large_integers = np.arange(1000, dtype=np.int64) # Uses 8 bytes per element
print(f"Small integers: {small_integers.nbytes} bytes")
print(f"Large integers: {large_integers.nbytes} bytes")
# Free memory when done with large arrays
del large_array
import gc
gc.collect() # Force garbage collection
Tools for troubleshooting NumPy operations:
# Set error handling
np.seterr(all='raise') # Raise exceptions on numerical errors
# Debugging with print statements
print(f"Array shape: {arr.shape}, dtype: {arr.dtype}")
print(f"Min: {np.min(arr)}, Max: {np.max(arr)}")
print(f"Contains NaN: {np.isnan(arr).any()}")
print(f"Contains Inf: {np.isinf(arr).any()}")
NumPy has become indispensable in scientific computing, data analysis, machine learning, and many other fields due to its:
- Performance: Vectorized operations and optimized C implementations
- Versatility: Support for a wide range of numerical operations
- Ecosystem integration: Seamless connection with other scientific Python libraries
- Simplicity: Clean, intuitive API for complex numerical operations
Whether you’re analyzing experimental data, building machine learning models, simulating physical systems, or processing images, NumPy provides the foundation for efficient numerical computation in Python. Its influence extends far beyond its direct usage, as it establishes patterns and data structures that the entire scientific Python ecosystem builds upon.
By mastering NumPy, you unlock not just a single library but gain the key to the entire world of scientific and data-driven computing in Python.
#NumPy #PythonDataScience #ScientificComputing #DataAnalysis #NumericalComputing #ArrayManipulation #PythonProgramming #MachineLearning #DataVisualization #LinearAlgebra #ImageProcessing #FinancialModeling #SimulationAnalysis #PythonLibrary #VectorizedComputing #DataStructures #PerformanceOptimization #DataScience #ComputationalMathematics #PythonForScience