SQL
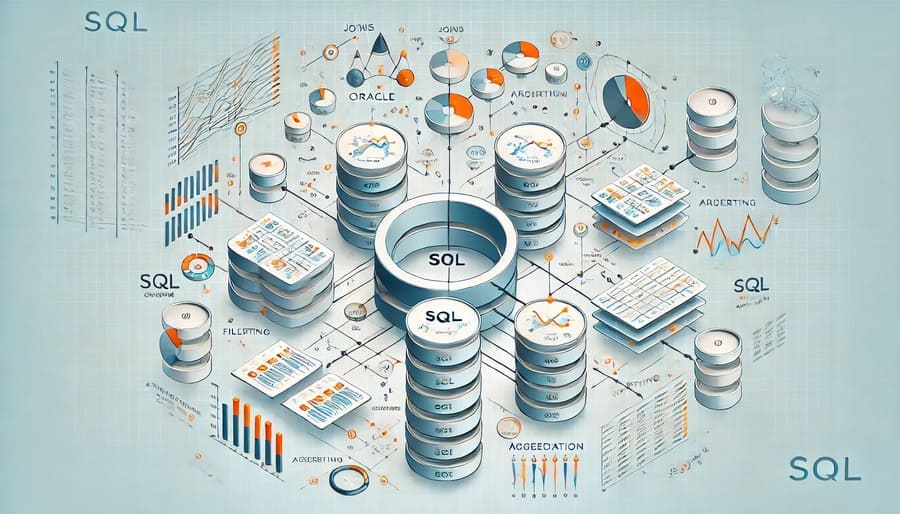
In the vast digital landscape where data reigns supreme, one language has consistently served as the foundation for how we store, access, and manipulate structured information. SQL—Structured Query Language—has stood the test of time as the standard language for working with relational databases. Whether you’re a seasoned database administrator, a data analyst, a developer, or just beginning your journey in the data world, understanding SQL is an invaluable skill that unlocks the ability to harness the power of organized data.
SQL emerged in the 1970s at IBM, based on Edgar F. Codd’s groundbreaking work on relational database theory. What began as a research project has evolved into the lingua franca of data management, enabling professionals across industries to speak a common language when it comes to working with structured data.
-- A simple SQL query that retrieves customer information
SELECT
customer_id,
first_name,
last_name,
city,
state
FROM
customers
WHERE
state = 'California'
ORDER BY
last_name, first_name;
This simple query demonstrates the readable, almost English-like syntax that makes SQL approachable yet powerful. With just a few lines, we can instruct a database containing millions of records to find exactly the information we need.
SQL encompasses several language components that work together to provide comprehensive data management capabilities:
DDL commands allow you to create and modify database objects like tables, indexes, and views:
-- Creating a table to store product information
CREATE TABLE products (
product_id INT PRIMARY KEY,
product_name VARCHAR(100) NOT NULL,
category VARCHAR(50),
price DECIMAL(10, 2) NOT NULL,
stock_quantity INT DEFAULT 0,
last_updated TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Adding a new column to track product ratings
ALTER TABLE products
ADD COLUMN average_rating DECIMAL(3, 2);
-- Creating an index to speed up searches by category
CREATE INDEX idx_category ON products(category);
DDL provides the architectural framework for your data, defining its structure, constraints, and relationships.
DML commands enable you to interact with the data itself, adding, modifying, and removing records:
-- Inserting a new product record
INSERT INTO products (product_id, product_name, category, price, stock_quantity)
VALUES (1001, 'Ergonomic Office Chair', 'Furniture', 249.99, 15);
-- Updating stock quantities after a sale
UPDATE products
SET stock_quantity = stock_quantity - 5,
last_updated = CURRENT_TIMESTAMP
WHERE product_id = 1001;
-- Removing discontinued products
DELETE FROM products
WHERE stock_quantity = 0 AND last_updated < DATE_SUB(CURRENT_DATE, INTERVAL 6 MONTH);
These operations form the backbone of data management, allowing applications to maintain accurate, up-to-date information.
The SELECT statement and its many clauses form the heart of SQL’s querying capabilities:
-- Find the total sales by product category for the past month
SELECT
p.category,
SUM(o.quantity * p.price) AS total_sales,
COUNT(DISTINCT o.customer_id) AS unique_customers
FROM
orders o
JOIN
order_items oi ON o.order_id = oi.order_id
JOIN
products p ON oi.product_id = p.product_id
WHERE
o.order_date >= DATE_SUB(CURRENT_DATE, INTERVAL 1 MONTH)
GROUP BY
p.category
HAVING
total_sales > 10000
ORDER BY
total_sales DESC;
DQL allows analysts and applications to extract meaningful insights from raw data, transforming information into actionable knowledge.
DCL commands manage access permissions, crucial for security in multi-user database environments:
-- Grant read-only access to the analytics team
GRANT SELECT ON orders TO role_analytics;
-- Allow the inventory manager to update product stock
GRANT UPDATE (stock_quantity, last_updated) ON products TO role_inventory;
-- Revoke permissions when an employee changes roles
REVOKE ALL PRIVILEGES ON customers FROM user_former_support;
These permission controls ensure that users can access only the data they need for their specific roles, maintaining security and compliance.
One of SQL’s most powerful features is its ability to combine data from multiple tables through various types of joins:
-- Inner join: Find customers and their most recent orders
SELECT
c.customer_id,
c.first_name,
c.last_name,
o.order_id,
o.order_date,
o.total_amount
FROM
customers c
INNER JOIN
orders o ON c.customer_id = o.customer_id
WHERE
o.order_date = (
SELECT MAX(order_date)
FROM orders
WHERE customer_id = c.customer_id
);
-- Left join: Include all customers, even those without orders
SELECT
c.customer_id,
c.first_name,
c.last_name,
COUNT(o.order_id) AS order_count
FROM
customers c
LEFT JOIN
orders o ON c.customer_id = o.customer_id
GROUP BY
c.customer_id, c.first_name, c.last_name;
-- Self join: Find employees and their managers
SELECT
e.employee_id,
e.first_name || ' ' || e.last_name AS employee_name,
m.first_name || ' ' || m.last_name AS manager_name
FROM
employees e
LEFT JOIN
employees m ON e.manager_id = m.employee_id;
Joins allow databases to implement relational data models, connecting information across different tables while maintaining data integrity and reducing redundancy.
Beyond the basics, SQL offers sophisticated features for complex data manipulation:
Window functions perform calculations across sets of rows related to the current row:
-- Calculate running totals and moving averages
SELECT
date,
amount,
SUM(amount) OVER (ORDER BY date) AS running_total,
AVG(amount) OVER (ORDER BY date ROWS BETWEEN 6 PRECEDING AND CURRENT ROW) AS 7day_avg
FROM
daily_sales;
-- Rank products by price within each category
SELECT
product_name,
category,
price,
RANK() OVER (PARTITION BY category ORDER BY price DESC) AS price_rank
FROM
products;
These functions are invaluable for time-series analysis, ranking calculations, and other complex analytical needs.
CTEs create temporary result sets that simplify complex queries:
-- Analyze the customer purchase funnel
WITH visitor_counts AS (
SELECT COUNT(DISTINCT visitor_id) AS total_visitors
FROM website_visits
WHERE visit_date = CURRENT_DATE - 1
),
add_to_cart AS (
SELECT COUNT(DISTINCT visitor_id) AS cart_visitors
FROM cart_actions
WHERE action_date = CURRENT_DATE - 1
),
purchases AS (
SELECT COUNT(DISTINCT customer_id) AS purchasing_customers
FROM orders
WHERE order_date = CURRENT_DATE - 1
)
SELECT
v.total_visitors,
c.cart_visitors,
p.purchasing_customers,
ROUND(c.cart_visitors / v.total_visitors * 100, 2) AS browse_to_cart_rate,
ROUND(p.purchasing_customers / c.cart_visitors * 100, 2) AS cart_to_purchase_rate,
ROUND(p.purchasing_customers / v.total_visitors * 100, 2) AS overall_conversion_rate
FROM
visitor_counts v, add_to_cart c, purchases p;
CTEs break down complex logic into manageable, readable segments, making queries easier to understand and maintain.
Recursive CTEs handle hierarchical or graph-structured data elegantly:
-- Find all reports in an organizational hierarchy
WITH RECURSIVE employee_hierarchy AS (
-- Base case: CEO (no manager)
SELECT
employee_id,
first_name,
last_name,
manager_id,
0 AS level
FROM
employees
WHERE
manager_id IS NULL
UNION ALL
-- Recursive case: employees with managers
SELECT
e.employee_id,
e.first_name,
e.last_name,
e.manager_id,
eh.level + 1
FROM
employees e
JOIN
employee_hierarchy eh ON e.manager_id = eh.employee_id
)
SELECT
employee_id,
first_name,
last_name,
level,
REPEAT(' ', level) || first_name || ' ' || last_name AS hierarchy_display
FROM
employee_hierarchy
ORDER BY
level, first_name;
Recursive queries elegantly solve problems that would otherwise require multiple queries or application-level logic.
SQL’s endurance is a testament to its flexibility and power. Today, SQL has expanded far beyond traditional relational database systems:
Modern big data platforms have embraced SQL as an interface:
-- Apache Spark SQL analyzing web logs stored in a data lake
SELECT
client_ip,
http_method,
path,
status_code,
COUNT(*) AS request_count
FROM
parquet.`s3://logs/webserver/2023/04/01/`
WHERE
status_code >= 400
GROUP BY
client_ip, http_method, path, status_code
HAVING
COUNT(*) > 100
ORDER BY
request_count DESC
LIMIT 20;
Platforms like Apache Spark, Presto, and Google BigQuery have extended SQL to work with petabytes of data across distributed systems.
Streaming SQL adapts traditional concepts to continuous data flows:
-- Kafka SQL analyzing real-time payment events
CREATE STREAM payment_stream (
payment_id VARCHAR KEY,
customer_id VARCHAR,
amount DOUBLE,
payment_time TIMESTAMP
) WITH (
kafka_topic = 'payments',
value_format = 'JSON'
);
-- Detect potentially fraudulent transactions
CREATE TABLE potential_fraud AS
SELECT
customer_id,
COUNT(*) AS payment_count,
SUM(amount) AS total_amount
FROM
payment_stream
WINDOW
TUMBLING (SIZE 5 MINUTES)
GROUP BY
customer_id
HAVING
COUNT(*) > 5 OR SUM(amount) > 10000;
Systems like Kafka SQL and Flink SQL bring SQL’s declarative approach to streaming data processing.
Even non-relational databases have adopted SQL-like query languages:
-- Querying document data in MongoDB using SQL
SELECT
c.first_name,
c.last_name,
c.email,
o.order_date,
o.items
FROM
customers c
JOIN
orders o ON c._id = o.customer_id
WHERE
o.status = "shipped"
AND o.order_date > "2023-01-01";
Many NoSQL databases now support SQL or SQL-like query interfaces, recognizing the language’s intuitive power.
SQL’s utility spans countless real-world scenarios:
SQL powers business intelligence tools that transform raw data into actionable insights:
-- Sales performance dashboard query
SELECT
DATE_TRUNC('month', order_date) AS month,
product_category,
sales_region,
SUM(order_total) AS revenue,
SUM(order_total) - SUM(product_cost) AS gross_profit,
COUNT(DISTINCT order_id) AS order_count,
COUNT(DISTINCT customer_id) AS customer_count,
SUM(order_total) / COUNT(DISTINCT customer_id) AS avg_customer_spend
FROM
fact_orders
JOIN
dim_products ON fact_orders.product_id = dim_products.product_id
JOIN
dim_regions ON fact_orders.region_id = dim_regions.region_id
WHERE
order_date BETWEEN '2023-01-01' AND '2023-12-31'
GROUP BY
DATE_TRUNC('month', order_date),
product_category,
sales_region
ORDER BY
month, revenue DESC;
SQL’s aggregation capabilities make it ideal for summarizing and analyzing business metrics.
Before analysis can begin, data often needs cleaning and standardization:
-- Standardize inconsistent company names
UPDATE customer_companies
SET company_name = CASE
WHEN company_name LIKE '%Microsoft%' THEN 'Microsoft Corporation'
WHEN company_name LIKE '%Google%' THEN 'Google LLC'
WHEN company_name LIKE '%Amazon%' THEN 'Amazon.com Inc.'
ELSE company_name
END
WHERE company_name IN (
SELECT company_name
FROM customer_companies
GROUP BY company_name
HAVING COUNT(*) > 1
);
-- Fill in missing values based on related records
UPDATE products p
SET category = (
SELECT category
FROM products
WHERE product_name LIKE p.product_name || '%'
AND category IS NOT NULL
LIMIT 1
)
WHERE category IS NULL;
SQL provides powerful tools for identifying and correcting data quality issues.
Most web applications rely on SQL databases to store and retrieve user data:
-- User authentication and session management
SELECT
user_id,
username,
email,
password_hash,
last_login,
account_status
FROM
users
WHERE
username = ? AND password_hash = HASH(?);
-- Insert user activity log
INSERT INTO user_activities (
user_id,
activity_type,
ip_address,
user_agent,
activity_time
) VALUES (
?,
'login',
?,
?,
CURRENT_TIMESTAMP
);
From e-commerce platforms to social media sites, SQL databases form the persistent storage layer for most web applications.
Writing effective SQL involves more than just syntax knowledge:
Well-designed queries and indexes dramatically improve performance:
-- Create targeted indexes for common query patterns
CREATE INDEX idx_orders_customer_date ON orders(customer_id, order_date);
-- Use EXPLAIN to analyze query execution plans
EXPLAIN ANALYZE
SELECT
c.customer_id,
c.email,
COUNT(o.order_id) AS order_count,
SUM(o.total_amount) AS lifetime_value
FROM
customers c
JOIN
orders o ON c.customer_id = o.customer_id
WHERE
c.signup_date > '2023-01-01'
GROUP BY
c.customer_id, c.email
HAVING
COUNT(o.order_id) > 5;
Understanding execution plans and indexing strategies ensures databases perform well even as they grow.
Protecting data requires careful attention to security principles:
-- Use parameterized queries to prevent SQL injection
-- Instead of:
-- "SELECT * FROM users WHERE username = '" + username + "' AND password = '" + password + "'"
-- Use prepared statements:
PREPARE user_lookup AS
SELECT * FROM users WHERE username = $1 AND password_hash = $2;
EXECUTE user_lookup('johndoe', '5f4dcc3b5aa765d61d8327deb882cf99');
-- Implement row-level security
CREATE POLICY account_isolation ON customer_accounts
USING (account_owner = current_user);
ALTER TABLE customer_accounts ENABLE ROW LEVEL SECURITY;
Practices like parameterized queries, proper authentication, and fine-grained access control protect against common security threats.
Clear, consistent SQL improves maintainability:
-- Use CTEs for improved readability
WITH monthly_sales AS (
SELECT
DATE_TRUNC('month', order_date) AS month,
SUM(total_amount) AS revenue
FROM
orders
WHERE
order_date >= DATE_TRUNC('year', CURRENT_DATE)
GROUP BY
DATE_TRUNC('month', order_date)
),
previous_year_sales AS (
SELECT
DATE_TRUNC('month', DATE_ADD('year', 1, order_date)) AS month,
SUM(total_amount) AS prev_year_revenue
FROM
orders
WHERE
order_date >= DATE_TRUNC('year', DATE_ADD('year', -1, CURRENT_DATE))
AND order_date < DATE_TRUNC('year', CURRENT_DATE)
GROUP BY
DATE_TRUNC('month', DATE_ADD('year', 1, order_date))
)
SELECT
ms.month,
ms.revenue,
pys.prev_year_revenue,
(ms.revenue - pys.prev_year_revenue) / pys.prev_year_revenue * 100 AS growth_percentage
FROM
monthly_sales ms
LEFT JOIN
previous_year_sales pys ON ms.month = pys.month
ORDER BY
ms.month;
Techniques like consistent formatting, descriptive naming, and breaking complex queries into logical parts make SQL code easier to understand and maintain.
The journey to SQL proficiency follows a natural progression:
- Start with the fundamentals: Learn basic SELECT queries, filtering, and sorting
- Master joins and relationships: Understand how to combine data from multiple tables
- Build aggregation skills: Use GROUP BY and aggregate functions for data summarization
- Explore advanced features: Dive into window functions, CTEs, and recursive queries
- Understand performance: Learn indexing, query optimization, and execution plans
- Apply to real projects: Build expertise through practical application to real-world problems
The beauty of SQL lies in its layered approach—you can start being productive with just the basics, while continuing to develop more advanced skills over time.
In an era of rapid technological change, SQL has demonstrated remarkable staying power. The language has not only survived decades of evolution in computing but has expanded its reach into new domains like big data, stream processing, and even machine learning integration.
SQL’s endurance can be attributed to several key factors:
- Declarative nature: SQL describes what data you want, not how to get it, allowing database engines to optimize execution
- Standardization: ANSI SQL provides a common foundation across different database systems
- Balance of power and accessibility: SQL is accessible to beginners yet powerful enough for complex data operations
- Adaptability: The language has evolved to address new data challenges while maintaining backward compatibility
As we move further into the age of data-driven decision making, SQL’s role as the universal language for working with structured data seems more secure than ever. Whether you’re analyzing customer behavior, building web applications, or exploring big data, SQL provides the tools to transform raw information into valuable insights.
For aspiring data professionals, the message is clear: investing time in mastering SQL yields returns across countless domains and technologies. It remains one of the most valuable skills in the modern technical landscape.
#SQL #DatabaseManagement #RelationalDatabases #DataAnalysis #QueryLanguage #DataScience #DatabaseDevelopment #RDBMS #SQLTutorial #DataEngineering #BusinessIntelligence #DatabaseAdministration #DataModeling #SQLServer #MySQL #PostgreSQL #OracleDatabase #DataQuery #SQLOptimization #DataManipulation