Julia
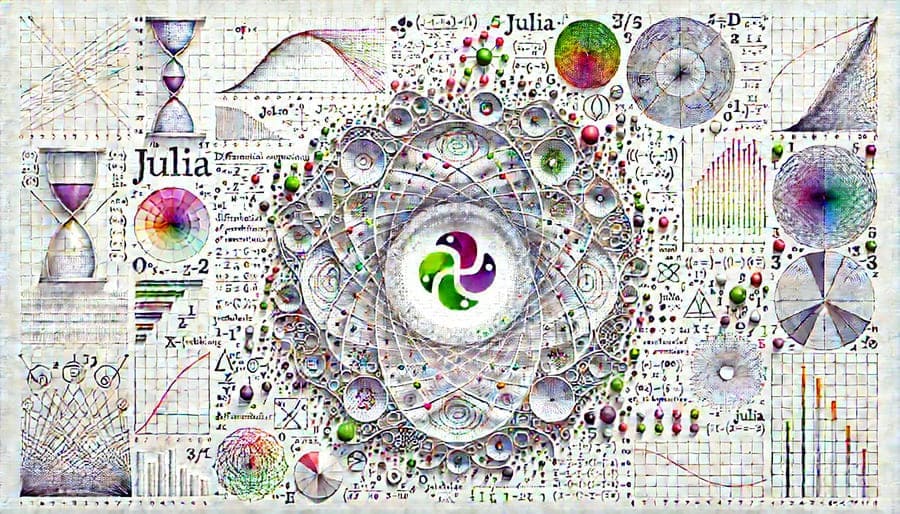
In the world of scientific computing and data analysis, the programming language you choose can significantly impact both development speed and computational efficiency. Enter Julia—a relatively young language that has rapidly gained traction for its remarkable ability to offer both high-level abstractions and blazing-fast performance. This comprehensive guide explores why Julia is revolutionizing numerical computing and why it might be the perfect tool for your next data-intensive project.
Julia was born in 2012 out of frustration with a common workflow in scientific computing: prototyping in a high-level language like Python or R, then rewriting performance-critical sections in C or Fortran. This “two-language problem” creates a significant productivity bottleneck and potential for errors during translation.
The creators of Julia—Jeff Bezanson, Stefan Karpinski, Viral Shah, and Alan Edelman—envisioned a language that could eliminate this inefficiency by providing both ease of use and C-like performance in a single package. Their ambitious goal is eloquently stated in what has become known as “The Julia Manifesto”:
# We want a language that's:
# 1. Open source
# 2. Fast as C
# 3. Expressive as Python
# 4. Dynamic and interactive
# 5. Specialized for scientific and numerical computing
Julia’s performance secret lies in its just-in-time compilation using LLVM, which allows it to generate optimized machine code at runtime:
# Simple function definition
function add_numbers(a, b)
return a + b
end
# Julia compiles specialized versions for each type combination
result1 = add_numbers(1, 2) # Compiles version for integers
result2 = add_numbers(1.0, 2.0) # Compiles version for floats
result3 = add_numbers([1, 2], [3, 4]) # Compiles version for arrays
While many languages use object-oriented programming with single dispatch (methods belong to objects), Julia uses multiple dispatch, where method behavior depends on all argument types:
# Define a generic function for area calculation
function area(shape)
error("Area not defined for shape of type $(typeof(shape))")
end
# Implement for different shapes using multiple dispatch
struct Circle
radius::Float64
end
struct Rectangle
width::Float64
height::Float64
end
area(c::Circle) = π * c.radius^2
area(r::Rectangle) = r.width * r.height
# Usage
circle = Circle(2.0)
rectangle = Rectangle(3.0, 4.0)
println("Circle area: $(area(circle))")
println("Rectangle area: $(area(rectangle))")
This paradigm allows for remarkably clean and extensible code that can be augmented without modifying existing definitions.
Julia’s syntax is exceptionally close to mathematical notation, making algorithms more readable:
# Julia allows Unicode symbols for variables and functions
α = 0.5
β = 1.0
γ = α + β
# Matrix operations look like mathematical notation
A = [1 2; 3 4]
b = [5, 6]
x = A \ b # Solves the system Ax = b
# Summation looks natural
function riemann_sum(f, a, b, n)
Δx = (b - a) / n
sum(f(a + i*Δx) * Δx for i in 0:n-1)
end
# Calculate integral of x² from 0 to 1
integral = riemann_sum(x -> x^2, 0, 1, 1000)
println("∫₀¹ x² dx ≈ $integral")
Julia was designed from the ground up with parallelism in mind, offering simple primitives for distributed and multi-threaded computation:
# Multi-threading
using Base.Threads
# Parallel array operations
function parallel_sum(arr)
result = Atomic{Float64}(0.0)
@threads for i in eachindex(arr)
atomic_add!(result, arr[i])
end
return result[]
end
# Distributed computing
using Distributed
addprocs(4) # Add 4 worker processes
@distributed (+) for i in 1:1000
1 / i^2 # Calculate part of the Basel problem series
end
Julia can easily call code written in C, Python, R, and other languages without special wrappers:
# Calling Python from Julia
using PyCall
np = pyimport("numpy")
plt = pyimport("matplotlib.pyplot")
x = np.linspace(0, 2π, 1000)
y = np.sin.(x)
plt.plot(x, y)
plt.title("Sine function")
plt.savefig("sine.png")
# Calling R from Julia
using RCall
R"""
data <- data.frame(x = 1:10, y = rnorm(10))
plot(data$x, data$y, main="R Plot from Julia")
"""
Julia’s DifferentialEquations.jl ecosystem is widely regarded as the state-of-the-art for numerically solving differential equations:
using DifferentialEquations
using Plots
# Define the Lorenz system
function lorenz!(du, u, p, t)
σ, ρ, β = p
du[1] = σ * (u[2] - u[1])
du[2] = u[1] * (ρ - u[3]) - u[2]
du[3] = u[1] * u[2] - β * u[3]
end
# Initial conditions and parameters
u0 = [1.0, 0.0, 0.0]
tspan = (0.0, 100.0)
p = (10.0, 28.0, 8/3)
# Solve the system
prob = ODEProblem(lorenz!, u0, tspan, p)
sol = solve(prob, Tsit5())
# Visualize
plot(sol, vars=(1, 2, 3), title="Lorenz Attractor")
Julia’s machine learning ecosystem is growing rapidly, with libraries like Flux.jl providing elegant deep learning capabilities:
using Flux
using Flux.Data: DataLoader
using Flux: onehotbatch, onecold, logitcrossentropy
using Flux.Optimise: update!
using MLDatasets
# Load MNIST dataset
train_x, train_y = MLDatasets.MNIST.traindata()
test_x, test_y = MLDatasets.MNIST.testdata()
# Reshape and normalize data
train_x = reshape(Float32.(train_x), 28, 28, 1, :)
test_x = reshape(Float32.(test_x), 28, 28, 1, :)
# One-hot encode labels
train_y = onehotbatch(train_y, 0:9)
test_y = onehotbatch(test_y, 0:9)
# Create data loaders
train_data = DataLoader((train_x, train_y), batchsize=128, shuffle=true)
# Define model (a simple CNN)
model = Chain(
Conv((3, 3), 1=>16, relu),
MaxPool((2, 2)),
Conv((3, 3), 16=>32, relu),
MaxPool((2, 2)),
flatten,
Dense(32*5*5, 10)
)
# Define loss function and optimizer
loss(x, y) = logitcrossentropy(model(x), y)
opt = ADAM(0.001)
# Training loop
for epoch in 1:10
for (x, y) in train_data
grads = gradient(() -> loss(x, y), params(model))
update!(opt, params(model), grads)
end
# Evaluate on test set
test_loss = loss(test_x, test_y)
accuracy = mean(onecold(model(test_x)) .== onecold(test_y))
println("Epoch $epoch: Test Accuracy: $accuracy")
end
Julia’s JuMP.jl is a standout for mathematical optimization problems:
using JuMP
using GLPK
# Create a model
model = Model(GLPK.Optimizer)
# Variables
@variable(model, x >= 0)
@variable(model, y >= 0)
# Objective function
@objective(model, Max, 5x + 3y)
# Constraints
@constraint(model, 2x + y <= 10)
@constraint(model, x + 2y <= 8)
# Solve
optimize!(model)
# Results
println("Optimal Solution:")
println("x = ", value(x))
println("y = ", value(y))
println("Objective value = ", objective_value(model))
Julia’s DataFrames.jl and Statistics ecosystem provide powerful tools for data manipulation and analysis:
using DataFrames
using CSV
using Statistics
using StatsPlots
# Create a sample dataset
df = DataFrame(
ID = 1:100,
Age = rand(18:65, 100),
Income = rand(30000:100000, 100),
Education = rand(["High School", "Bachelor's", "Master's", "PhD"], 100)
)
# Basic statistics
describe(df)
# Group by and summarize
gdf = groupby(df, :Education)
summary_stats = combine(gdf,
:Age => mean => :MeanAge,
:Income => mean => :MeanIncome,
nrow => :Count
)
# Visualization
@df df scatter(:Age, :Income, group=:Education,
title="Income vs Age by Education",
xlabel="Age", ylabel="Income")
Julia’s built-in parallel computing features make it excellent for HPC applications:
using Distributed
using SharedArrays
# Add worker processes
addprocs(4)
# Define a parallel Monte Carlo pi estimation
function estimate_pi(n)
points_inside_circle = @distributed (+) for i in 1:n
x, y = rand(), rand()
Int(x^2 + y^2 <= 1)
end
return 4 * points_inside_circle / n
end
# Run with 100 million points
pi_estimate = estimate_pi(10^8)
println("π estimate: $pi_estimate")
The Climate Machine (CLIMA) project uses Julia to build next-generation climate models that can run efficiently on exascale supercomputers, taking advantage of Julia’s performance and parallelism.
BioJulia offers bioinformatics tools that run orders of magnitude faster than equivalent Python implementations, enabling researchers to process larger genomic datasets and simulate complex biological systems more efficiently.
Julia Computing’s JuliaFin packages provide tools for quantitative finance, risk analysis, and algorithmic trading, with the speed necessary for real-time financial calculations.
NASA and other space agencies have adopted Julia for spacecraft control systems and mission planning, where its combination of safety features and performance is crucial.
Installing Julia is straightforward:
- Download the appropriate installer for your platform from julialang.org
- Run the installer or extract the binary
- Add Julia to your system PATH
- Launch Julia from the terminal or command prompt
Julia’s built-in package manager makes it easy to install and manage dependencies:
# Enter package mode by typing ']' at the REPL
# Or programmatically:
using Pkg
# Install a package
Pkg.add("DataFrames")
# Update all packages
Pkg.update()
# Use a specific environment
Pkg.activate("my_project")
Here’s a simple example to get you started:
function analyze_dataset(filename)
# Read data
data = CSV.read(filename, DataFrame)
# Basic analysis
println("Dataset summary:")
println("Number of rows: ", nrow(data))
println("Number of columns: ", ncol(data))
# Numeric columns analysis
numeric_cols = names(data, Real)
for col in numeric_cols
println("$col: mean = $(mean(data[!, col])), std = $(std(data[!, col]))")
end
# Return the processed data
return data
end
While Julia offers tremendous advantages, it’s important to consider some challenges:
Julia’s just-in-time compilation means the first execution of code can have a noticeable delay (known as the “time-to-first-plot” problem). This is improving with each release but can be surprising to newcomers.
While Julia’s ecosystem is growing rapidly, it’s still younger than Python’s or R’s. Some specialized libraries might not be available yet, though the ability to call Python and R helps bridge this gap.
Julia introduces concepts like multiple dispatch that might be unfamiliar to programmers from other backgrounds. However, the payoff in code organization and expressiveness is substantial.
Julia is experiencing strong growth in adoption and continues to evolve rapidly. Future developments to watch include:
- Static compilation improvements to address the latency issue
- GPU acceleration becoming even more seamless
- Machine learning ecosystem maturation
- Embedded systems support for real-time applications
- Web development tools to bring Julia’s numerical prowess to web applications
Julia shines brightest when:
- You need high performance for numerical calculations
- Your problem domain involves complex mathematics
- You’re working with differential equations, optimization, or simulation
- You want to avoid the two-language problem
- You value both productivity and performance
While it may not replace general-purpose languages for every application, Julia offers a compelling solution for computational scientists, financial analysts, bioinformaticians, and others working with complex numerical problems.
As the language and its ecosystem continue to mature, Julia is positioned to become an increasingly important tool in the scientific computing landscape—one that delivers on its promise of combining the best aspects of traditional scientific programming languages into a modern, accessible package.
#JuliaLang #ScientificComputing #NumericalAnalysis #HighPerformanceComputing #DataScience #ComputationalScience #DifferentialEquations #MachineLearning #Optimization #ParallelComputing #ProgrammingLanguages #ComputationalPhysics #FinancialModeling #ScientificProgramming #PerformanceOptimization #Mathematics #Statistics #MultipleDispatch #TechnicalComputing #JIT