Terraform: Infrastructure as Code Software Tool
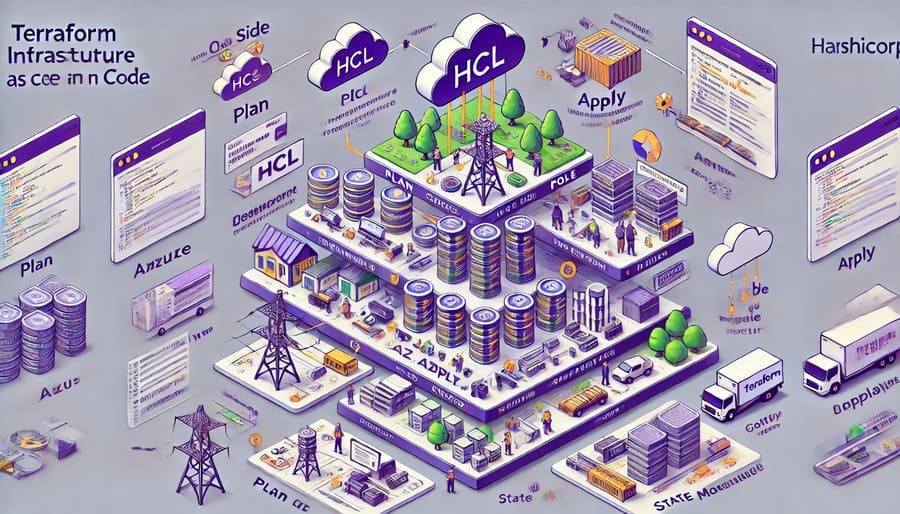
In the rapidly evolving landscape of cloud computing and infrastructure management, few tools have transformed the way organizations build and maintain their technology foundations as profoundly as Terraform. Created by HashiCorp, Terraform has established itself as the leading infrastructure as code (IaC) solution, enabling engineers to define, provision, and manage complex infrastructure using simple, declarative configuration files.
Before we dive into Terraform’s capabilities, it’s worth understanding the evolution that led to its creation. Traditionally, infrastructure was managed manually—system administrators would click through console interfaces or run commands to set up servers, networks, and other resources. This approach was error-prone, difficult to scale, and nearly impossible to reproduce consistently.
As cloud computing emerged, the number of infrastructure components grew exponentially, making manual management increasingly impractical. This challenge gave birth to the “Infrastructure as Code” movement, where infrastructure configurations are defined in code, version-controlled, and automatically deployed—much like application code.
Terraform, launched in 2014, quickly became a frontrunner in this space by offering a provider-agnostic approach to infrastructure provisioning. Rather than being tied to a specific cloud platform, Terraform allowed engineers to use a consistent workflow across multiple providers and services.
At its heart, Terraform operates on a few fundamental principles that drive its functionality:
Terraform uses HashiCorp Configuration Language (HCL), a declarative language designed for describing infrastructure:
resource "aws_s3_bucket" "data_lake" {
bucket = "enterprise-data-lake"
acl = "private"
versioning {
enabled = true
}
server_side_encryption_configuration {
rule {
apply_server_side_encryption_by_default {
sse_algorithm = "AES256"
}
}
}
tags = {
Environment = "Production"
Department = "Data Engineering"
}
}
This code snippet defines an AWS S3 bucket with specific properties. The declarative approach means you specify the desired end state rather than the steps to achieve it.
Before making any changes, Terraform creates an execution plan that outlines exactly what will happen:
Terraform will perform the following actions:
# aws_s3_bucket.data_lake will be created
+ resource "aws_s3_bucket" "data_lake" {
+ acceleration_status = (known after apply)
+ acl = "private"
+ arn = (known after apply)
+ bucket = "enterprise-data-lake"
+ bucket_domain_name = (known after apply)
# ... other properties ...
}
Plan: 1 to add, 0 to change, 0 to destroy.
This preview capability allows engineers to validate changes before implementation, significantly reducing the risk of unexpected outcomes.
Terraform builds a dependency graph of all resources, enabling it to create or modify resources in the correct order:
digraph {
compound = "true"
newrank = "true"
subgraph "root" {
"[root] aws_s3_bucket.data_lake" [label = "aws_s3_bucket.data_lake", shape = "box"]
"[root] aws_iam_role.data_processing" [label = "aws_iam_role.data_processing", shape = "box"]
"[root] aws_iam_policy_attachment.s3_access" [label = "aws_iam_policy_attachment.s3_access", shape = "box"]
"[root] aws_iam_policy_attachment.s3_access" -> "[root] aws_s3_bucket.data_lake"
"[root] aws_iam_policy_attachment.s3_access" -> "[root] aws_iam_role.data_processing"
}
}
This graph-based approach ensures that interdependent resources are created in the proper sequence.
Terraform tracks the state of resources it manages, allowing it to understand what exists and what needs to change:
{
"version": 4,
"terraform_version": "1.0.0",
"serial": 3,
"lineage": "3f6b0918-627d-9c2a-5f9e-94f7723212c5",
"outputs": {},
"resources": [
{
"mode": "managed",
"type": "aws_s3_bucket",
"name": "data_lake",
"provider": "provider[\"registry.terraform.io/hashicorp/aws\"]",
"instances": [
{
"schema_version": 0,
"attributes": {
"acl": "private",
"bucket": "enterprise-data-lake",
# ... other attributes ...
}
}
]
}
]
}
This state file is crucial for Terraform’s operation, as it maps real-world resources to your configuration.
For data engineering teams, Terraform offers powerful capabilities for managing complex data infrastructure:
# Define a VPC for data platform resources
resource "aws_vpc" "data_platform" {
cidr_block = "10.0.0.0/16"
tags = {
Name = "data-platform-vpc"
}
}
# Create subnets for different tiers
resource "aws_subnet" "private" {
count = 3
vpc_id = aws_vpc.data_platform.id
cidr_block = "10.0.${count.index + 1}.0/24"
availability_zone = data.aws_availability_zones.available.names[count.index]
tags = {
Name = "private-subnet-${count.index + 1}"
}
}
# Set up a data warehouse
resource "aws_redshift_cluster" "analytics" {
cluster_identifier = "data-warehouse"
database_name = "analytics"
master_username = var.redshift_username
master_password = var.redshift_password
node_type = "ra3.4xlarge"
cluster_type = "multi-node"
number_of_nodes = 4
vpc_security_group_ids = [aws_security_group.redshift.id]
cluster_subnet_group_name = aws_redshift_subnet_group.analytics.name
encrypted = true
tags = {
Environment = "Production"
Department = "Data Engineering"
}
}
# Configure a data processing EMR cluster
resource "aws_emr_cluster" "processing" {
name = "data-processing-cluster"
release_label = "emr-6.5.0"
applications = ["Spark", "Hive", "Presto"]
ec2_attributes {
subnet_id = aws_subnet.private[0].id
instance_profile = aws_iam_instance_profile.emr.name
emr_managed_master_security_group = aws_security_group.emr_master.id
emr_managed_slave_security_group = aws_security_group.emr_slave.id
service_access_security_group = aws_security_group.emr_service.id
}
master_instance_group {
instance_type = "m5.xlarge"
}
core_instance_group {
instance_type = "r5.2xlarge"
instance_count = 4
ebs_config {
size = "100"
type = "gp3"
volumes_per_instance = 1
}
}
# ... additional configuration ...
}
# Set up streaming data ingestion
resource "aws_kinesis_firehose_delivery_stream" "events" {
name = "events-ingestion-stream"
destination = "s3"
s3_configuration {
role_arn = aws_iam_role.firehose_role.arn
bucket_arn = aws_s3_bucket.data_lake.arn
prefix = "raw/events/"
buffer_size = 5
buffer_interval = 60
compression_format = "GZIP"
}
tags = {
Environment = "Production"
}
}
This configuration demonstrates how Terraform can provision a complete data platform with networking, data warehousing, processing, and ingestion components.
Terraform’s module system allows for creating reusable infrastructure components:
module "data_lake" {
source = "./modules/data-lake"
bucket_name = "enterprise-data-lake"
environment = "production"
enable_versioning = true
lifecycle_rules = var.data_retention_policies
}
module "data_warehouse" {
source = "./modules/redshift"
cluster_name = "analytics-warehouse"
database_name = "analytics"
node_type = "ra3.4xlarge"
number_of_nodes = 4
subnet_ids = module.vpc.private_subnet_ids
vpc_id = module.vpc.vpc_id
master_username = var.redshift_username
master_password = var.redshift_password
}
module "spark_processing" {
source = "./modules/emr"
cluster_name = "data-processing"
release_label = "emr-6.5.0"
applications = ["Spark", "Hive", "Presto"]
instance_groups = var.processing_instance_groups
subnet_id = module.vpc.private_subnet_ids[0]
vpc_id = module.vpc.vpc_id
}
This modular approach promotes code reuse, maintainability, and consistency across environments.
Terraform excels at managing multiple environments with minimal code duplication:
# Define provider configuration
provider "aws" {
region = var.aws_region
# Use different AWS profiles for different environments
profile = terraform.workspace == "prod" ? "production" : "development"
}
locals {
# Environment-specific settings
env = {
dev = {
instance_type = "r5.large"
instance_count = 2
retention_days = 30
}
staging = {
instance_type = "r5.xlarge"
instance_count = 2
retention_days = 60
}
prod = {
instance_type = "r5.2xlarge"
instance_count = 4
retention_days = 90
}
}
# Use current workspace for environment selection
environment = terraform.workspace
settings = local.env[local.environment]
}
# Resources use the environment-specific settings
resource "aws_emr_cluster" "processing" {
name = "${local.environment}-data-processing"
# ... other settings ...
master_instance_group {
instance_type = local.settings.instance_type
}
core_instance_group {
instance_type = local.settings.instance_type
instance_count = local.settings.instance_count
}
}
This approach allows the same configuration to be deployed with environment-specific settings using Terraform workspaces.
As data infrastructure scales, several advanced Terraform techniques become valuable:
For teams collaborating on infrastructure, remote state storage is essential:
terraform {
backend "s3" {
bucket = "terraform-state-bucket"
key = "data-platform/terraform.tfstate"
region = "us-east-1"
dynamodb_table = "terraform-locks"
encrypt = true
}
}
This configuration stores state in S3 with locking via DynamoDB, enabling safe collaboration.
For organizations working across multiple clouds or regions:
provider "aws" {
alias = "us_east"
region = "us-east-1"
}
provider "aws" {
alias = "us_west"
region = "us-west-2"
}
module "east_data_warehouse" {
source = "./modules/redshift"
providers = {
aws = aws.us_east
}
# ... configuration ...
}
module "west_data_warehouse" {
source = "./modules/redshift"
providers = {
aws = aws.us_west
}
# ... configuration ...
}
This approach enables consistent deployments across regions or cloud providers.
For sophisticated configurations, Terraform’s built-in functions provide powerful capabilities:
locals {
# Generate list of CIDR blocks for subnets
subnet_cidrs = [
for index in range(var.subnet_count) :
cidrsubnet(var.vpc_cidr, 8, index)
]
# Create map of tags common across all resources
common_tags = merge(
var.default_tags,
{
Environment = var.environment
ManagedBy = "Terraform"
Project = var.project_name
}
)
# Calculate appropriate cluster size based on data volume
processing_nodes = var.data_volume_gb > 1000 ? 8 : (
var.data_volume_gb > 500 ? 4 : 2
)
}
These functions enable dynamic calculations, transformations, and conditional logic within your configurations.
For maximum effectiveness, Terraform should be integrated into your broader DevOps processes:
# Example GitLab CI configuration for Terraform
stages:
- validate
- plan
- apply
validate:
stage: validate
script:
- terraform init -backend=false
- terraform validate
- terraform fmt -check
plan:
stage: plan
script:
- terraform init
- terraform plan -out=tfplan
artifacts:
paths:
- tfplan
apply:
stage: apply
script:
- terraform init
- terraform apply -auto-approve tfplan
dependencies:
- plan
only:
- main
when: manual
This pipeline demonstrates how Terraform can be integrated into CI/CD processes for automated infrastructure deployment.
Infrastructure testing can be implemented using tools like Terratest:
package test
import (
"testing"
"github.com/gruntwork-io/terratest/modules/terraform"
"github.com/stretchr/testify/assert"
)
func TestDataLakeModule(t *testing.T) {
terraformOptions := &terraform.Options{
TerraformDir: "../modules/data-lake",
Vars: map[string]interface{}{
"bucket_name": "test-data-lake",
"environment": "test",
},
}
defer terraform.Destroy(t, terraformOptions)
terraform.InitAndApply(t, terraformOptions)
// Test outputs
bucketId := terraform.Output(t, terraformOptions, "bucket_id")
assert.Equal(t, "test-data-lake", bucketId)
// Additional assertions...
}
This approach allows you to verify infrastructure configurations behave as expected.
Regular drift detection can identify unauthorized changes:
#!/bin/bash
# Script to detect and report infrastructure drift
terraform plan -detailed-exitcode
EXITCODE=$?
if [ $EXITCODE -eq 0 ]; then
echo "No changes detected"
elif [ $EXITCODE -eq 2 ]; then
echo "Drift detected!"
terraform show -json tfplan | jq '.resource_changes[] | select(.change.actions[0] != "no-op")'
# Optionally, send alerts or trigger remediation
if [ "$AUTO_REMEDIATE" = "true" ]; then
terraform apply -auto-approve
else
# Send alert to operations team
curl -X POST $ALERT_WEBHOOK -d "Infrastructure drift detected in $(pwd)"
fi
else
echo "Error running terraform plan"
exit 1
fi
This script can run as a scheduled job to identify and optionally remediate configuration drift.
Based on real-world experience, here are key best practices for effective Terraform usage:
terraform/
├── environments/
│ ├── dev/
│ │ ├── main.tf
│ │ ├── variables.tf
│ │ └── terraform.tfvars
│ ├── staging/
│ └── production/
├── modules/
│ ├── networking/
│ ├── data-storage/
│ ├── data-processing/
│ └── monitoring/
└── scripts/
├── apply.sh
└── plan-all.sh
This structure separates modules, environment-specific configurations, and utility scripts for better maintainability.
Always specify version constraints for providers and modules:
terraform {
required_version = ">= 1.0.0, < 2.0.0"
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 4.0"
}
snowflake = {
source = "Snowflake-Labs/snowflake"
version = "~> 0.40"
}
}
}
These constraints prevent unexpected changes when new versions are released.
Implement thorough variable definitions with validation:
variable "environment" {
description = "Deployment environment (dev, staging, prod)"
type = string
validation {
condition = contains(["dev", "staging", "prod"], var.environment)
error_message = "Environment must be one of: dev, staging, prod."
}
}
variable "redshift_node_count" {
description = "Number of nodes in Redshift cluster"
type = number
default = 2
validation {
condition = var.redshift_node_count >= 1
error_message = "Redshift node count must be at least 1."
}
}
This approach prevents configuration errors and improves self-documentation.
Document outputs thoroughly for better usability:
output "data_lake_bucket_name" {
description = "Name of the S3 bucket used for the data lake"
value = aws_s3_bucket.data_lake.id
}
output "redshift_connection_string" {
description = "JDBC connection string for the Redshift cluster"
value = "jdbc:redshift://${aws_redshift_cluster.analytics.endpoint}:5439/${aws_redshift_cluster.analytics.database_name}"
sensitive = false
}
output "database_password" {
description = "Password for the database (sensitive)"
value = var.database_password
sensitive = true
}
Well-documented outputs make your modules more useful to others.
For complex, multi-environment deployments, Terragrunt adds valuable capabilities:
# terragrunt.hcl
include {
path = find_in_parent_folders()
}
terraform {
source = "git::git@github.com:company/terraform-modules.git//data-platform?ref=v1.0.0"
}
inputs = {
environment = "production"
region = "us-east-1"
vpc_cidr = "10.0.0.0/16"
instance_type = "r5.2xlarge"
retention_days = 90
}
# Remote state configuration
remote_state {
backend = "s3"
config = {
bucket = "company-terraform-states"
key = "${path_relative_to_include()}/terraform.tfstate"
region = "us-east-1"
encrypt = true
dynamodb_table = "terraform-locks"
}
}
Terragrunt provides DRY configurations, improved workflow automation, and additional features for managing complex deployments.
When evaluating Terraform against other infrastructure as code tools, several distinctions emerge:
Feature | Terraform | CloudFormation | Pulumi | Ansible |
---|---|---|---|---|
Language | HCL (declarative) | YAML/JSON (declarative) | Programming languages (TypeScript, Python, etc.) | YAML (procedural) |
State Management | External state file | Managed by AWS | External state file | Stateless (with limitations) |
Providers | Multi-cloud, 1000+ integrations | AWS-specific | Multi-cloud | Agentless, broad support |
Learning Curve | Moderate | Moderate | Varies by language | Gentle |
Execution | Push-based | Push-based | Push-based | Push-based (agentless) |
Maturity | Very mature | Mature (AWS only) | Growing | Mature |
Community | Very large | Large (AWS-centric) | Growing | Very large |
Terraform’s key advantages include its multi-cloud support, extensive provider ecosystem, and declarative approach. For data engineering teams working across multiple clouds or with diverse infrastructure, these benefits often make Terraform the preferred choice.
As infrastructure continues to evolve, several trends are shaping Terraform’s future:
For teams preferring programming languages over HCL, CDKTF enables Terraform configurations using TypeScript, Python, Java, and others:
import { Construct } from 'constructs';
import { App, TerraformStack, TerraformOutput } from 'cdktf';
import { AwsProvider } from '@cdktf/provider-aws/lib/provider';
import { S3Bucket } from '@cdktf/provider-aws/lib/s3-bucket';
import { GlueDatabase } from '@cdktf/provider-aws/lib/glue-database';
class DataLakeStack extends TerraformStack {
constructor(scope: Construct, name: string) {
super(scope, name);
// Define AWS provider
new AwsProvider(this, 'aws', {
region: 'us-east-1'
});
// Create data lake bucket
const dataLakeBucket = new S3Bucket(this, 'dataLake', {
bucket: 'enterprise-data-lake',
versioning: {
enabled: true
},
serverSideEncryptionConfiguration: {
rule: {
applyServerSideEncryptionByDefault: {
sseAlgorithm: 'AES256'
}
}
}
});
// Create Glue database for metadata
const glueDatabase = new GlueDatabase(this, 'glueCatalog', {
name: 'data_lake_catalog',
catalogId: '${aws_account_id}'
});
// Define outputs
new TerraformOutput(this, 'bucketName', {
value: dataLakeBucket.bucket
});
}
}
const app = new App();
new DataLakeStack(app, 'data-lake-infrastructure');
app.synth();
This approach brings the power of programming languages to Terraform while maintaining its provider ecosystem and execution model.
Terraform’s security capabilities continue to expand, with tools like Checkov for policy as code:
# Example Checkov policy
from checkov.terraform.checks.resource.base_resource_check import BaseResourceCheck
from checkov.common.models.enums import CheckResult
class S3BucketEncryption(BaseResourceCheck):
def __init__(self):
name = "Ensure S3 bucket has encryption enabled"
id = "CKV_AWS_19"
supported_resources = ['aws_s3_bucket']
categories = ['encryption']
super().__init__(name=name, id=id, categories=categories, supported_resources=supported_resources)
def scan_resource_conf(self, conf):
if 'server_side_encryption_configuration' in conf:
return CheckResult.PASSED
return CheckResult.FAILED
These capabilities help organizations enforce security and compliance requirements across their infrastructure.
HashiCorp continues to enhance Terraform Cloud and Enterprise with features like:
- No-code provisioning through ServiceNow integration
- Cost estimation for infrastructure changes
- Policy as code with Sentinel and OPA
- Run tasks for integration with security scanning and custom workflows
- Dynamic provider credentials for improved security
These enterprise features make Terraform even more powerful for large organizations with complex governance requirements.
Terraform has fundamentally transformed how organizations manage infrastructure, bringing software engineering practices to infrastructure deployment and operations. Its declarative approach, provider-agnostic design, and powerful ecosystem make it an invaluable tool for modern data engineering teams.
By treating infrastructure as code, Terraform enables consistent, repeatable deployments across environments and cloud providers. Its ability to preview changes, track state, and integrate with CI/CD pipelines brings confidence and reliability to infrastructure management—qualities that are particularly valuable in data engineering contexts where infrastructure often underpins critical business operations.
Whether you’re building a data lake on AWS, a processing pipeline on Google Cloud, or a multi-cloud analytics platform, Terraform provides the foundation for defining, deploying, and evolving your infrastructure in a controlled, secure, and efficient manner. As cloud infrastructure continues to grow in complexity and importance, tools like Terraform will remain essential for organizations seeking to harness the full power of the cloud while maintaining governance, control, and agility.
Keywords: Terraform, HashiCorp, Infrastructure as Code, IaC, HCL, cloud automation, state management, multi-cloud, provider, modules, data engineering, DevOps, configuration management, CloudFormation, Pulumi, Terragrunt, CDKTF
#Terraform #InfrastructureAsCode #IaC #DevOps #CloudAutomation #DataEngineering #MultiCloud #HashiCorp #HCL #CloudComputing #DataOps #TerraformModules #CDKTF #ConfigurationManagement #CloudInfrastructure