Google Cloud Deployment Manager: Infrastructure Deployment Service
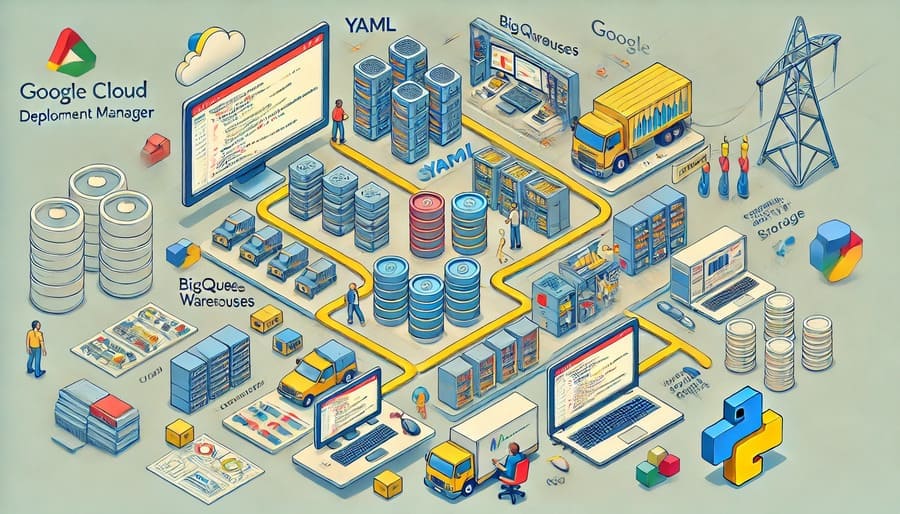
In the rapidly evolving landscape of cloud computing, efficiently managing and deploying infrastructure has become a critical challenge for organizations of all sizes. Google Cloud Platform (GCP) addresses this need with Google Cloud Deployment Manager, a powerful infrastructure-as-code (IaC) service that enables teams to automate the creation, provisioning, and management of cloud resources.
Google Cloud Deployment Manager is a native GCP service that allows you to specify all the resources needed for your applications in a declarative format using YAML or Python. This approach transforms infrastructure management from manual, error-prone processes into repeatable, version-controlled deployments that can be treated with the same rigor as application code.
Unlike other cloud providers that might require you to learn proprietary languages, Deployment Manager leverages familiar and widely-used formats:
resources:
- name: vm-instance
type: compute.v1.instance
properties:
zone: us-central1-a
machineType: zones/us-central1-a/machineTypes/n1-standard-1
disks:
- deviceName: boot
type: PERSISTENT
boot: true
autoDelete: true
initializeParams:
sourceImage: projects/debian-cloud/global/images/family/debian-10
networkInterfaces:
- network: global/networks/default
accessConfigs:
- name: External NAT
type: ONE_TO_ONE_NAT
This simple YAML configuration declares a virtual machine with specific characteristics, showcasing the declarative approach that makes Deployment Manager both powerful and approachable.
Deployment Manager embraces the declarative paradigm, where you specify what resources you want rather than how to create them. This approach offers several advantages:
- Predictability: The system handles the “how” based on your specification of “what”
- Idempotency: You can apply the same configuration multiple times without adverse effects
- Self-documenting: The configuration itself serves as documentation for your infrastructure
One of Deployment Manager’s standout features is its support for templates, which enable reusability and modularization:
imports:
- path: vm_template.jinja
resources:
- name: web-servers
type: vm_template.jinja
properties:
zone: us-central1-a
machineType: n1-standard-2
count: 3
Templates can be written in Jinja2 or Python, providing flexibility to handle complex logic while maintaining readability:
# vm_template.py
def GenerateConfig(context):
resources = []
for i in range(context.properties['count']):
vm_name = context.env['name'] + '-' + str(i)
resources.append({
'name': vm_name,
'type': 'compute.v1.instance',
'properties': {
'zone': context.properties['zone'],
'machineType': 'zones/' + context.properties['zone'] + '/machineTypes/' + context.properties['machineType'],
# Additional properties...
}
})
return {'resources': resources}
This approach allows you to create abstractions that simplify complex deployments and enforce standards across your organization.
Before making changes to your infrastructure, Deployment Manager’s preview feature lets you see exactly what would happen:
gcloud deployment-manager deployments create my-deployment --config config.yaml --preview
This capability is invaluable for understanding the impact of changes, especially in complex environments where resources have interdependencies.
As a native Google Cloud service, Deployment Manager integrates seamlessly with the broader GCP ecosystem:
- IAM Integration: Leverage Google Cloud’s identity and access management for fine-grained control
- Cloud Audit Logs: Track who made what changes to your infrastructure
- Cloud Monitoring: Monitor the health and performance of deployed resources
- Google Cloud Console: Visualize and manage deployments through the intuitive web interface
This tight integration creates a cohesive experience that’s difficult to achieve with third-party tools.
For data engineering teams, Deployment Manager offers specific advantages in managing complex data infrastructure:
resources:
- name: analytics-dataset
type: bigquery.v2.dataset
properties:
datasetReference:
datasetId: analytics
location: US
description: Analytics dataset for business intelligence
defaultTableExpirationMs: 7776000000 # 90 days
access:
- role: OWNER
userByEmail: data-engineers@example.com
- role: READER
groupByEmail: analysts@example.com
- name: events-table
type: bigquery.v2.table
properties:
datasetId: $(ref.analytics-dataset.datasetReference.datasetId)
tableReference:
tableId: events
description: User events tracking table
schema:
fields:
- name: event_id
type: STRING
mode: REQUIRED
- name: user_id
type: STRING
mode: REQUIRED
- name: event_type
type: STRING
mode: REQUIRED
- name: event_timestamp
type: TIMESTAMP
mode: REQUIRED
- name: properties
type: RECORD
mode: NULLABLE
fields:
- name: page
type: STRING
mode: NULLABLE
- name: referrer
type: STRING
mode: NULLABLE
timePartitioning:
type: DAY
field: event_timestamp
clustering:
fields:
- event_type
- user_id
This configuration creates a BigQuery dataset with specified access controls and a properly structured, partitioned, and clustered table—ensuring your data warehouse is optimized for both performance and governance from day one.
resources:
- name: data-processing-cluster
type: dataproc.v1.cluster
properties:
region: us-central1
clusterName: data-processing
config:
gceClusterConfig:
zoneUri: us-central1-a
subnetworkUri: $(ref.processing-subnet.selfLink)
serviceAccount: $(ref.processing-service-account.email)
serviceAccountScopes:
- https://www.googleapis.com/auth/cloud-platform
masterConfig:
numInstances: 1
machineTypeUri: n1-standard-4
diskConfig:
bootDiskSizeGb: 500
workerConfig:
numInstances: 4
machineTypeUri: n1-standard-4
diskConfig:
bootDiskSizeGb: 500
softwareConfig:
imageVersion: 2.0
optionalComponents:
- JUPYTER
- ZEPPELIN
- name: processing-bucket
type: storage.v1.bucket
properties:
location: US-CENTRAL1
storageClass: STANDARD
lifecycle:
rule:
- action:
type: Delete
condition:
age: 30
This example creates a Dataproc cluster for data processing along with a storage bucket for intermediate data, all properly configured with appropriate machine types, disk sizes, and lifecycle policies.
For more complex scenarios, you can combine multiple resources into a comprehensive data pipeline:
imports:
- path: data_pipeline.py
resources:
- name: analytics-pipeline
type: data_pipeline.py
properties:
region: us-central1
ingestionBucketName: data-ingestion-bucket
processingClusterMachineType: n1-standard-4
processingClusterWorkerCount: 4
warehouseDatasetLocation: US
dataRetentionDays: 90
notificationEmail: data-alerts@example.com
The corresponding Python template might create all the necessary components:
- Cloud Storage buckets for data ingestion
- Pub/Sub topics and subscriptions for event notifications
- Dataflow templates for stream processing
- Cloud Functions for data validation
- BigQuery datasets and tables for the data warehouse
- Data Studio dashboards for reporting
This modular approach allows data engineering teams to standardize infrastructure while providing flexibility where needed.
Based on real-world experience, here are some best practices for using Deployment Manager effectively:
Organize your Deployment Manager configurations to promote reuse:
deployments/
├── templates/
│ ├── network/
│ │ ├── vpc.py
│ │ └── firewall.py
│ ├── compute/
│ │ ├── instance_group.py
│ │ └── load_balancer.py
│ └── data/
│ ├── bigquery_dataset.py
│ └── dataflow_pipeline.py
├── environments/
│ ├── development/
│ │ └── config.yaml
│ ├── staging/
│ │ └── config.yaml
│ └── production/
│ └── config.yaml
└── modules/
├── analytics_platform.py
└── data_lake.py
This structure separates reusable templates from environment-specific configurations, making it easier to maintain consistency across environments.
Leverage Deployment Manager’s reference system to create dependencies between resources:
resources:
- name: analytics-vpc
type: compute.v1.network
properties:
autoCreateSubnetworks: false
- name: analytics-subnet
type: compute.v1.subnetwork
properties:
network: $(ref.analytics-vpc.selfLink)
region: us-central1
ipCidrRange: 10.0.0.0/24
- name: analytics-firewall
type: compute.v1.firewall
properties:
network: $(ref.analytics-vpc.selfLink)
sourceRanges: ["10.0.0.0/24"]
allowed:
- IPProtocol: tcp
ports: ["22", "3389"]
This approach not only creates proper dependencies but also ensures you’re referencing the actual deployed resource rather than hardcoding identifiers.
Use properties to customize deployments for different environments:
# environments/production/config.yaml
imports:
- path: ../../modules/analytics_platform.py
resources:
- name: production-analytics
type: analytics_platform.py
properties:
environment: production
highAvailability: true
machineType: n1-standard-8
replicaCount: 3
backupRetentionDays: 30
monitoringAlertEmail: prod-alerts@example.com
# environments/development/config.yaml
imports:
- path: ../../modules/analytics_platform.py
resources:
- name: development-analytics
type: analytics_platform.py
properties:
environment: development
highAvailability: false
machineType: n1-standard-2
replicaCount: 1
backupRetentionDays: 7
monitoringAlertEmail: dev-alerts@example.com
This pattern allows you to maintain a single template while accommodating the different requirements of development, staging, and production environments.
In Python templates, implement robust error handling to provide clear feedback:
def GenerateConfig(context):
"""Generates deployment configuration."""
properties = context.properties
# Validate required properties
required_properties = ['region', 'machineType', 'workerCount']
for prop in required_properties:
if prop not in properties:
raise Exception(f"Required property '{prop}' is missing")
# Validate property values
if properties['workerCount'] < 2:
raise Exception("Worker count must be at least 2 for minimal redundancy")
# Resource generation logic...
resources = [...]
return {'resources': resources}
This validation helps catch configuration errors early, before they lead to failed deployments or suboptimal infrastructure.
Comprehensive documentation makes templates easier to use and maintain:
"""BigQuery Dataset Template
This template creates a BigQuery dataset with configurable access controls
and optional default table expiration.
Required properties:
- datasetId: The ID of the dataset to create
- location: Geographic location of the dataset (e.g., 'US', 'EU')
Optional properties:
- description: Description of the dataset
- defaultTableExpirationMs: Default expiration time for tables in milliseconds
- access: List of access control entries (see examples below)
Example usage:
imports:
- path: templates/data/bigquery_dataset.py
resources:
- name: analytics-dataset
type: templates/data/bigquery_dataset.py
properties:
datasetId: analytics
location: US
description: Analytics dataset for reporting
defaultTableExpirationMs: 7776000000 # 90 days
access:
- role: OWNER
userByEmail: data-admin@example.com
- role: READER
groupByEmail: analysts@example.com
"""
def GenerateConfig(context):
# Implementation...
This documentation helps others understand how to use your templates without having to read through the implementation details.
For maximum effectiveness, integrate Deployment Manager into your broader DevOps processes:
# cloudbuild.yaml
steps:
# Test the configuration
- name: 'gcr.io/cloud-builders/gcloud'
id: 'test-config'
entrypoint: 'bash'
args:
- '-c'
- |
gcloud deployment-manager deployments validate \
--config environments/$(echo ${_ENVIRONMENT})/config.yaml
# Deploy with preview for manual approval
- name: 'gcr.io/cloud-builders/gcloud'
id: 'deploy-preview'
entrypoint: 'bash'
args:
- '-c'
- |
gcloud deployment-manager deployments $(if [ -z "$(gcloud deployment-manager deployments list --filter="name=${_DEPLOYMENT_NAME}" --format='get(name)')" ]; then echo "create"; else echo "update"; fi) ${_DEPLOYMENT_NAME} \
--config environments/$(echo ${_ENVIRONMENT})/config.yaml \
--preview \
--create-policy=CREATE_OR_ACQUIRE
# Actual deployment (requires approval)
- name: 'gcr.io/cloud-builders/gcloud'
id: 'deploy'
entrypoint: 'bash'
args:
- '-c'
- |
gcloud deployment-manager deployments $(if [ "$(gcloud deployment-manager deployments describe ${_DEPLOYMENT_NAME} --format='get(properties.deployment.operation.status)')" = "PREVIEW" ]; then echo "update"; else echo "create"; fi) ${_DEPLOYMENT_NAME} \
$(if [ "$(gcloud deployment-manager deployments describe ${_DEPLOYMENT_NAME} --format='get(properties.deployment.operation.status)')" = "PREVIEW" ]; then echo "--no-preview"; else echo "--config environments/$(echo ${_ENVIRONMENT})/config.yaml"; fi) \
--create-policy=CREATE_OR_ACQUIRE
substitutions:
_ENVIRONMENT: 'development'
_DEPLOYMENT_NAME: 'analytics-platform'
options:
dynamic_substitutions: true
This Cloud Build configuration validates your deployment configuration, creates a preview for manual review, and then completes the deployment after approval.
For critical infrastructure, implement testing to validate your deployments:
import unittest
import yaml
from deployment_validator import validate_deployment
class TestAnalyticsPlatform(unittest.TestCase):
def setUp(self):
with open('environments/production/config.yaml', 'r') as f:
self.config = yaml.safe_load(f)
def test_high_availability_enabled(self):
"""Ensure production has high availability enabled."""
resources = self.config.get('resources', [])
analytics_platform = next((r for r in resources if r['type'].endswith('analytics_platform.py')), None)
self.assertIsNotNone(analytics_platform, "Analytics platform resource not found")
self.assertTrue(
analytics_platform['properties'].get('highAvailability', False),
"High availability should be enabled in production"
)
def test_resource_validation(self):
"""Validate all resources in the deployment."""
validation_result = validate_deployment(self.config)
self.assertTrue(validation_result.valid, f"Validation failed: {validation_result.errors}")
if __name__ == '__main__':
unittest.main()
This testing approach can catch configuration issues before they reach production, ensuring your data infrastructure remains reliable.
For data engineering teams evaluating infrastructure deployment options, it’s helpful to understand how Deployment Manager compares to alternatives:
Feature | Google Cloud Deployment Manager | Terraform | AWS CloudFormation | Azure Resource Manager |
---|---|---|---|---|
Native Integration | Native to GCP | Cross-cloud | Native to AWS | Native to Azure |
Language | YAML, Python, Jinja2 | HCL | JSON, YAML | JSON, Bicep |
Learning Curve | Moderate (familiar formats) | Steeper (custom HCL) | Moderate | Moderate |
State Management | Managed by GCP | Local or remote state | Managed by AWS | Managed by Azure |
Extensibility | Python for custom logic | Provider system | Limited to CloudFormation | ARM functions |
Preview Capability | Yes | Yes (plan) | Yes (change sets) | Yes |
Adoption in Data Engineering | Common in GCP-centric teams | Very common (multi-cloud) | Common in AWS-centric teams | Common in Azure-centric teams |
For teams primarily working with Google Cloud Platform, Deployment Manager offers the tightest integration and simplest workflow. However, for multi-cloud scenarios, tools like Terraform may offer advantages despite the steeper learning curve.
As cloud infrastructure continues to evolve, several trends are shaping the future of Deployment Manager:
- Enhanced container and serverless support for modern application architectures
- Deeper integration with CI/CD pipelines for streamlined delivery
- Advanced compliance and security features for regulated industries
- Improved visualization and management tools for complex deployments
- Integration with AI-driven recommendations for optimal resource configuration
For data engineering teams, these advancements promise to make infrastructure deployment even more efficient and reliable, allowing greater focus on data processing and insights rather than infrastructure management.
Google Cloud Deployment Manager represents a powerful approach to infrastructure management for data engineering teams working with GCP. By treating infrastructure as code, it enables consistent, repeatable deployments while reducing the risk of configuration errors.
The service’s native integration with the Google Cloud ecosystem, combined with its support for familiar languages like YAML and Python, makes it an attractive option for teams seeking to automate their data infrastructure deployment. Whether you’re setting up a simple data processing pipeline or a complex analytics platform, Deployment Manager provides the tools to define, deploy, and manage your resources effectively.
As organizations continue to embrace cloud-native approaches to data engineering, tools like Deployment Manager will play an increasingly important role in ensuring that infrastructure can be deployed reliably, consistently, and at scale—ultimately enabling faster delivery of data-driven insights to the business.
Keywords: Google Cloud Deployment Manager, infrastructure as code, GCP, cloud automation, declarative configuration, YAML, Python, Jinja2, data engineering, BigQuery, Dataproc, Dataflow, templates, CI/CD integration, cloud resources, deployment automation, infrastructure testing
#GoogleCloud #DeploymentManager #InfrastructureAsCode #GCP #CloudAutomation #DataEngineering #IaC #CloudInfrastructure #YAML #Python #BigQuery #Dataproc #DevOps #CloudArchitecture #DataOps