AWS CloudFormation: Amazon’s Infrastructure as Code Service
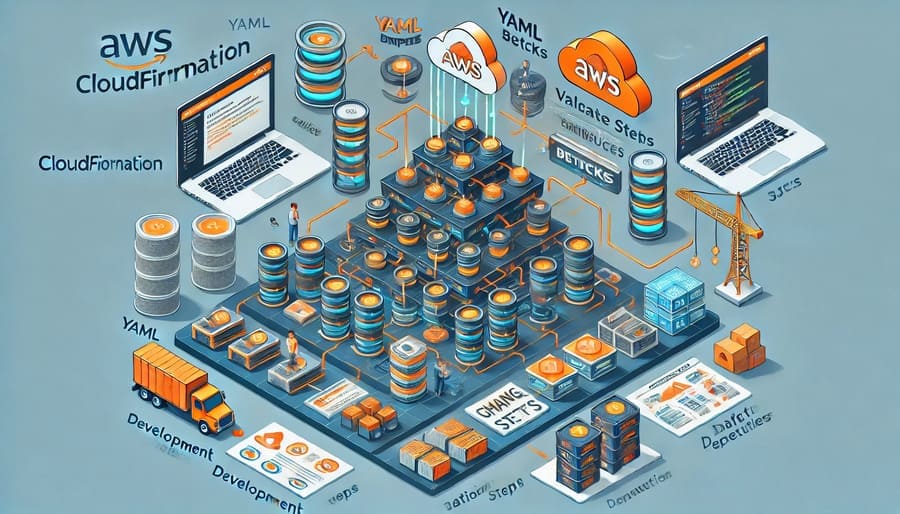
In the ever-evolving landscape of cloud computing, managing infrastructure efficiently has become paramount for organizations of all sizes. AWS CloudFormation stands as Amazon’s powerful answer to this challenge—a comprehensive Infrastructure as Code (IaC) service that enables developers and DevOps teams to define, deploy, and manage AWS resources programmatically and at scale.
At its core, CloudFormation enables you to describe your entire infrastructure using template files. Rather than navigating through the AWS Management Console to provision resources individually, you can define everything—from basic compute instances to complex multi-tier applications—in code. This declarative approach dramatically transforms how cloud infrastructure is managed.
AWSTemplateFormatVersion: '2010-09-09'
Description: 'Basic S3 bucket configuration'
Resources:
DataBucket:
Type: 'AWS::S3::Bucket'
Properties:
BucketName: !Sub 'data-lake-${AWS::AccountId}'
VersioningConfiguration:
Status: Enabled
BucketEncryption:
ServerSideEncryptionConfiguration:
- ServerSideEncryptionByDefault:
SSEAlgorithm: AES256
Tags:
- Key: Environment
Value: Production
- Key: Purpose
Value: DataLake
This simple YAML template demonstrates CloudFormation’s declarative syntax, defining an S3 bucket with specific configurations and tags. Once deployed, CloudFormation creates and configures this resource exactly as specified.
CloudFormation brings several transformative capabilities to infrastructure management:
You specify what resources you want and their configurations, not how to create them. CloudFormation determines the optimal order for provisioning resources and handles all the complex orchestration for you.
CloudFormation organizes resources into logical groupings called “stacks.” These stacks can be created, updated, or deleted as a single unit, ensuring that resources remain in sync. For multi-region or multi-account deployments, Stack Sets extend this capability across organizational boundaries.
# Example of a CloudFormation Stack Set deployment configuration
StackSetName: global-networking-infrastructure
Description: Core networking components deployed across regions
TemplateURL: https://s3.amazonaws.com/templates/network-template.yaml
Parameters:
- ParameterKey: VpcCidr
ParameterValue: 10.0.0.0/16
OperationPreferences:
RegionConcurrencyType: PARALLEL
MaxConcurrentPercentage: 100
Regions:
- us-east-1
- us-west-2
- eu-central-1
- ap-southeast-1
Before implementing changes, CloudFormation can generate a preview through Change Sets, showing exactly what modifications will occur to your infrastructure. This preview capability helps prevent unexpected changes and provides a safety check before implementation.
Infrastructure can change over time as teams make manual adjustments. CloudFormation’s drift detection identifies when resources have been modified outside the template, helping maintain consistency and prevent configuration drift.
# Detect drift in a CloudFormation stack using AWS CLI
aws cloudformation detect-stack-drift --stack-name ProductionDataPipeline
# Get detailed drift information
aws cloudformation describe-stack-resource-drifts --stack-name ProductionDataPipeline
When you need to incorporate resources beyond AWS or perform custom provisioning logic, CloudFormation Custom Resources let you extend its capabilities through Lambda functions.
For data engineering teams, CloudFormation offers powerful capabilities for creating and managing data infrastructure:
Resources:
DataLakeBucket:
Type: AWS::S3::Bucket
Properties:
BucketName: !Sub 'enterprise-data-lake-${AWS::AccountId}'
LifecycleConfiguration:
Rules:
- Id: ArchiveRule
Status: Enabled
Transitions:
- TransitionInDays: 90
StorageClass: GLACIER
ExpirationInDays: 2555 # 7 years
GlueDatabase:
Type: AWS::Glue::Database
Properties:
CatalogId: !Ref AWS::AccountId
DatabaseInput:
Name: data_lake_catalog
Description: Catalog for our enterprise data lake
RawDataCrawler:
Type: AWS::Glue::Crawler
Properties:
Name: raw-data-crawler
Role: !GetAtt GlueServiceRole.Arn
DatabaseName: !Ref GlueDatabase
Targets:
S3Targets:
- Path: !Sub 's3://${DataLakeBucket}/raw/'
Schedule:
ScheduleExpression: 'cron(0 0 * * ? *)'
This CloudFormation snippet defines a data lake architecture with S3 storage, AWS Glue database for metadata, and an automated crawler to discover schema information.
Resources:
ProcessingBucket:
Type: AWS::S3::Bucket
Properties:
BucketName: !Sub 'data-processing-${AWS::AccountId}'
DataProcessingCluster:
Type: AWS::EMR::Cluster
Properties:
Name: DataProcessingCluster
Applications:
- Name: Spark
- Name: Hive
- Name: Presto
Instances:
MasterInstanceGroup:
InstanceCount: 1
InstanceType: m5.xlarge
CoreInstanceGroup:
InstanceCount: 4
InstanceType: r5.2xlarge
TerminationProtected: true
BootstrapActions:
- Name: InstallCustomLibraries
ScriptBootstrapAction:
Path: !Sub 's3://${ProcessingBucket}/bootstrap/install_libraries.sh'
Configurations:
- Classification: spark-defaults
ConfigurationProperties:
spark.executor.memory: 5g
spark.driver.memory: 10g
This example provisions an EMR cluster for data processing with Spark, Hive, and Presto, configured with appropriate instance types and performance settings.
Resources:
DataWarehouse:
Type: AWS::Redshift::Cluster
Properties:
ClusterIdentifier: analytics-warehouse
NodeType: ra3.4xlarge
NumberOfNodes: 3
MasterUsername: !Ref MasterUsername
MasterUserPassword: !Ref MasterUserPassword
DatabaseName: analytics
Encrypted: true
IamRoles:
- !GetAtt RedshiftServiceRole.Arn
VpcSecurityGroupIds:
- !Ref WarehouseSecurityGroup
Tags:
- Key: Environment
Value: Production
WarehouseSecurityGroup:
Type: AWS::EC2::SecurityGroup
Properties:
GroupDescription: Security group for data warehouse
VpcId: !Ref VpcId
SecurityGroupIngress:
- IpProtocol: tcp
FromPort: 5439
ToPort: 5439
CidrIp: !Ref AllowedCidr
This snippet creates a Redshift data warehouse cluster with encryption enabled, appropriate security groups, and IAM roles for data access.
For more sophisticated deployments, CloudFormation offers several advanced capabilities:
Large infrastructures can be modularized using nested stacks, where one CloudFormation template references and incorporates others:
Resources:
NetworkingStack:
Type: AWS::CloudFormation::Stack
Properties:
TemplateURL: https://s3.amazonaws.com/templates/networking.yaml
Parameters:
VPCCidrBlock: 10.0.0.0/16
DatabaseStack:
Type: AWS::CloudFormation::Stack
Properties:
TemplateURL: https://s3.amazonaws.com/templates/database.yaml
Parameters:
VPCId: !GetAtt NetworkingStack.Outputs.VPCId
SubnetIds: !GetAtt NetworkingStack.Outputs.PrivateSubnetIds
ApplicationStack:
Type: AWS::CloudFormation::Stack
Properties:
TemplateURL: https://s3.amazonaws.com/templates/application.yaml
Parameters:
VPCId: !GetAtt NetworkingStack.Outputs.VPCId
SubnetIds: !GetAtt NetworkingStack.Outputs.PublicSubnetIds
DatabaseEndpoint: !GetAtt DatabaseStack.Outputs.DatabaseEndpoint
This approach improves organization and allows specialized teams to own different parts of the infrastructure.
CloudFormation’s transformation capabilities let you extend its functionality and simplify complex template patterns:
Transform: 'AWS::Serverless-2021-07-20'
Resources:
DataProcessingFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
CodeUri: s3://lambda-artifacts/data-processor.zip
Events:
DataUpload:
Type: S3
Properties:
Bucket: !Ref DataBucket
Events: s3:ObjectCreated:*
Filter:
S3Key:
Suffix: .json
This example uses the AWS Serverless Application Model (SAM) transform to simplify Lambda function configuration with S3 event triggers.
For sensitive configuration values, CloudFormation integrates with AWS Systems Manager Parameter Store and Secrets Manager:
Resources:
DatabaseInstance:
Type: AWS::RDS::DBInstance
Properties:
Engine: postgres
DBInstanceClass: db.r5.large
MasterUsername: admin
MasterUserPassword: '{{resolve:ssm-secure:/database/production/password:1}}'
AllocatedStorage: 100
StorageType: gp2
MultiAZ: true
This feature ensures sensitive information like passwords remains secure and isn’t stored in plaintext within templates.
Based on industry experience, here are key best practices for effective CloudFormation usage:
Structure your templates for maintainability:
infrastructure/
├── templates/
│ ├── networking/
│ │ ├── vpc.yaml
│ │ └── security-groups.yaml
│ ├── data/
│ │ ├── s3-data-lake.yaml
│ │ ├── glue-catalog.yaml
│ │ └── redshift-warehouse.yaml
│ └── applications/
│ ├── emr-processing.yaml
│ └── analytics-dashboard.yaml
├── parameters/
│ ├── dev/
│ ├── staging/
│ └── production/
└── scripts/
└── deploy.sh
This organization separates concerns and makes navigation intuitive.
Use parameter files to manage environment-specific values:
# parameters/production/data-warehouse.json
[
{
"ParameterKey": "EnvironmentName",
"ParameterValue": "Production"
},
{
"ParameterKey": "NodeType",
"ParameterValue": "ra3.4xlarge"
},
{
"ParameterKey": "NodeCount",
"ParameterValue": "4"
},
{
"ParameterKey": "VpcId",
"ParameterValue": "vpc-0a1b2c3d4e5f6g7h8"
}
]
This approach keeps templates environment-agnostic and simplifies deployment to different environments.
Tags are crucial for resource organization, cost allocation, and governance:
Resources:
AnalyticsDataBucket:
Type: AWS::S3::Bucket
Properties:
# Bucket properties...
Tags:
- Key: Project
Value: !Ref ProjectName
- Key: Environment
Value: !Ref EnvironmentName
- Key: Department
Value: "Data Engineering"
- Key: CostCenter
Value: !Ref CostCenter
- Key: ManagedBy
Value: "CloudFormation"
Consistent tagging improves resource management and cost visibility.
Structure your stacks in logical layers:
- Foundation Layer: VPC, subnets, security groups, IAM roles
- Data Layer: Storage, databases, data catalogs
- Processing Layer: EMR clusters, Glue jobs, Lambda functions
- Application Layer: Analytics tools, dashboards, APIs
This layering creates a natural dependency flow and separation of concerns.
Automate CloudFormation deployments using CI/CD pipelines:
# Example AWS CodePipeline configuration
version: 0.2
phases:
install:
runtime-versions:
python: 3.9
pre_build:
commands:
- pip install cfn-lint
- pip install aws-sam-cli
build:
commands:
- cfn-lint templates/**/*.yaml
- aws cloudformation validate-template --template-body file://templates/main.yaml
- aws cloudformation deploy --template-file templates/main.yaml --stack-name data-platform --parameter-overrides file://parameters/production/parameters.json --capabilities CAPABILITY_NAMED_IAM
This approach applies software engineering best practices to infrastructure code.
When evaluating CloudFormation against other Infrastructure as Code tools, consider these key comparisons:
Feature | AWS CloudFormation | Terraform | Pulumi | AWS CDK |
---|---|---|---|---|
Language | YAML/JSON | HCL | Various (Python, TypeScript, etc.) | TypeScript, Python, Java, .NET |
AWS Integration | Native, deep integration | Good, via provider | Good, via provider | Native, built on CloudFormation |
Multi-cloud Support | AWS only | Strong multi-cloud | Strong multi-cloud | AWS-focused with limited multi-cloud |
Learning Curve | Moderate | Moderate | Varies by language | Depends on language familiarity |
State Management | Managed by AWS | External state file | External state file | Managed by AWS |
Provisioning Logic | Limited (Macros) | Limited (expression syntax) | Full programming language | Full programming language |
Maturity | Very mature | Mature | Newer | Newer |
CloudFormation’s key advantages include native AWS integration, managed state, and deep service support. For teams primarily working with AWS, these benefits often outweigh the more flexible programming model offered by alternatives.
CloudFormation continues to evolve with the cloud computing landscape:
The AWS CDK represents the future of CloudFormation, allowing infrastructure definition in familiar programming languages while retaining CloudFormation’s deployment engine:
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
import * as glue from 'aws-cdk-lib/aws-glue';
export class DataLakeStack extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// Create a data lake bucket with lifecycle rules
const dataLakeBucket = new s3.Bucket(this, 'DataLakeBucket', {
bucketName: `data-lake-${this.account}`,
versioned: true,
encryption: s3.BucketEncryption.S3_MANAGED,
lifecycleRules: [
{
id: 'ArchiveRule',
transitions: [
{
storageClass: s3.StorageClass.GLACIER,
transitionAfter: cdk.Duration.days(90),
},
],
expiration: cdk.Duration.days(2555),
},
],
});
// Create a Glue database and crawler
const glueDatabase = new glue.CfnDatabase(this, 'GlueDatabase', {
catalogId: this.account,
databaseInput: {
name: 'data_lake_catalog',
description: 'Catalog for our enterprise data lake',
},
});
// Add more resources as needed...
}
}
This CDK code generates CloudFormation templates but offers the power of a full programming language for infrastructure definition.
CloudFormation’s growing support for AI/ML services is transforming how data science infrastructure is deployed:
Resources:
ModelTrainingJob:
Type: AWS::SageMaker::TrainingJob
Properties:
AlgorithmSpecification:
TrainingImage: 763104351884.dkr.ecr.us-east-1.amazonaws.com/tensorflow-training:2.6-cpu-py38
TrainingInputMode: File
RoleArn: !GetAtt SageMakerExecutionRole.Arn
InputDataConfig:
- ChannelName: training
DataSource:
S3DataSource:
S3Uri: !Sub 's3://${DataBucket}/training-data/'
S3DataType: S3Prefix
OutputDataConfig:
S3OutputPath: !Sub 's3://${ModelBucket}/output/'
ResourceConfig:
InstanceCount: 1
InstanceType: ml.c5.2xlarge
VolumeSizeInGB: 50
StoppingCondition:
MaxRuntimeInSeconds: 86400
These capabilities enable complete ML workflows defined as infrastructure.
CloudFormation’s security capabilities continue to expand, with features like:
- Drift detection for compliance monitoring
- Integration with AWS Security Hub
- Policy as Code through AWS CloudFormation Guard
# CloudFormation Guard rule file (rules.guard)
AWS::S3::Bucket {
BucketEncryption.ServerSideEncryptionConfiguration[*].ServerSideEncryptionByDefault.SSEAlgorithm == "AES256" || "aws:kms"
VersioningConfiguration.Status == "Enabled"
# Ensure all buckets have access logging
LoggingConfiguration exists
}
AWS::RDS::DBInstance {
# Ensure all databases are encrypted
StorageEncrypted == true
# Ensure multi-AZ for production
when Environment == "Production" {
MultiAZ == true
}
}
These enhancements help organizations maintain security and compliance as infrastructure scales.
AWS CloudFormation stands as a cornerstone of infrastructure automation on the AWS platform. Its declarative approach to resource definition, combined with powerful orchestration capabilities, enables organizations to manage complex cloud environments with precision and consistency.
For data engineering teams in particular, CloudFormation provides the foundation for building robust, scalable data platforms—from data lakes and processing engines to analytics systems and machine learning infrastructure. By treating infrastructure as code, teams can apply software engineering best practices to infrastructure management, improving reliability, security, and development velocity.
As cloud adoption continues to accelerate and infrastructure grows increasingly complex, tools like CloudFormation will remain essential for organizations seeking to harness the full power of the cloud while maintaining control, consistency, and governance across their environments.
Keywords: AWS CloudFormation, Infrastructure as Code, IaC, AWS, cloud automation, stacks, templates, nested stacks, CloudFormation macros, drift detection, change sets, YAML, JSON, AWS CDK, data engineering, data lake, data warehouse, DevOps
#AWSCloudFormation #InfrastructureAsCode #AWS #CloudAutomation #IaC #DevOps #CloudComputing #DataEngineering #AWSCDK #CloudDeployment #DataLake #DataWarehouse #Serverless #CloudNative #DataOps