Pulumi: Infrastructure as Code Using Programming Languages
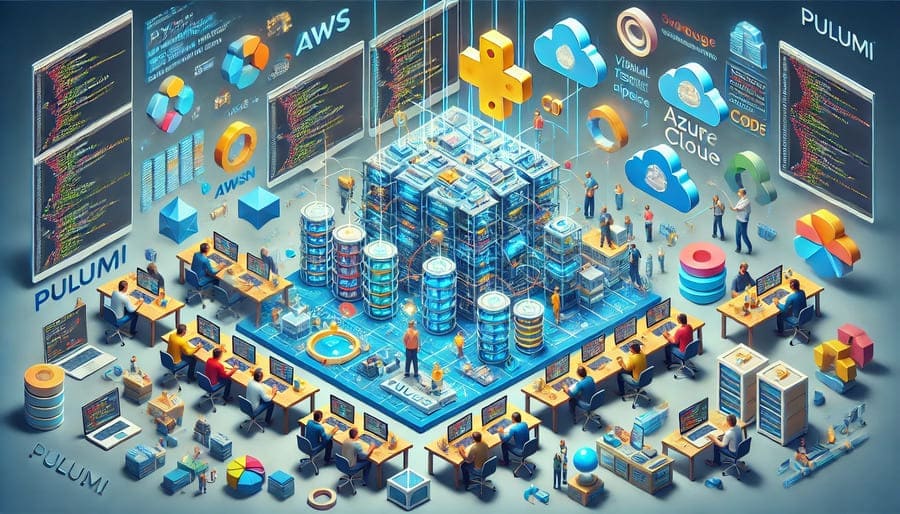
In the rapidly evolving world of cloud infrastructure, the ability to define, deploy, and manage resources programmatically has become essential. While traditional Infrastructure as Code (IaC) tools have relied on domain-specific languages and configuration files, Pulumi has pioneered a different approach: using familiar programming languages to define infrastructure. This paradigm shift has empowered developers to apply software engineering best practices to infrastructure code, bridging the gap between application development and infrastructure management.
Traditional IaC tools like Terraform, CloudFormation, and ARM Templates use declarative configuration languages or templating systems to define infrastructure. While effective, these approaches often lack the expressiveness, abstraction capabilities, and ecosystem benefits that general-purpose programming languages provide.
Pulumi’s innovation lies in allowing developers to use languages they already know—Python, TypeScript/JavaScript, Go, C#, Java, and others—to define cloud infrastructure. This approach brings several transformative advantages:
With Pulumi, infrastructure definitions benefit from the full power of programming languages:
import pulumi
import pulumi_aws as aws
# Create a reusable function for standard tags
def standard_tags(service, environment):
return {
"Service": service,
"Environment": environment,
"ManagedBy": "Pulumi"
}
# Create an S3 bucket with the standard tags
bucket = aws.s3.Bucket("data-lake",
acl="private",
tags=standard_tags("data-lake", "production")
)
# Export the bucket name
pulumi.export("bucket_name", bucket.id)
In the ever-evolving landscape of cloud infrastructure management, Pulumi has emerged as a revolutionary approach to Infrastructure as Code (IaC). Unlike traditional IaC tools that rely on domain-specific languages or templating formats, Pulumi empowers developers to define cloud infrastructure using familiar general-purpose programming languages. This paradigm shift brings the full power of software engineering practices to infrastructure deployment, bridging the gap between application development and infrastructure management.
The journey of infrastructure management has evolved significantly over the past decade:
- Manual Configuration: Initially, infrastructure was provisioned through manual processes—clicking through console interfaces or running CLI commands.
- Configuration Management: Tools like Chef and Puppet introduced automation for server configuration.
- Declarative IaC: Services like AWS CloudFormation and tools like Terraform brought declarative, template-based approaches to infrastructure definition.
- Programmatic IaC: Pulumi represents the next evolution—using actual programming languages to define infrastructure with all the capabilities they offer.
Pulumi’s approach stands out by leveraging languages developers already know, bringing software engineering principles like abstraction, modularity, and testing to infrastructure code.
What makes Pulumi’s approach transformative is the ability to use real programming languages like Python, TypeScript/JavaScript, Go, C#, Java, and YAML to define infrastructure. This unlocks several powerful capabilities:
# Python example of a reusable function to create standardized storage
def create_data_bucket(name, environment, versioning=True, encryption=True):
"""Create a standardized data storage bucket with best practices."""
bucket = storage.Bucket(
f"{name}-{environment}",
versioning_enabled=versioning,
server_side_encryption_configuration={
"rule": {
"apply_server_side_encryption_by_default": {
"sse_algorithm": "AES256" if encryption else None
}
}
},
tags={
"Environment": environment,
"Department": "Data Engineering",
"ManagedBy": "Pulumi"
}
)
return bucket
# Creating multiple buckets with consistent configuration
raw_data = create_data_bucket("raw-data", "production")
processed_data = create_data_bucket("processed-data", "production")
analytics_data = create_data_bucket("analytics", "production", versioning=False)
This approach allows for the creation of higher-level abstractions tailored to your organization’s needs, something difficult to achieve with template-based tools.
// TypeScript example of environment-specific configuration
import * as aws from "@pulumi/aws";
const config = new pulumi.Config();
const environment = config.require("environment");
const isProduction = environment === "production";
// Create a database with environment-specific settings
const database = new aws.rds.Instance("database", {
engine: "postgres",
instanceClass: isProduction ? "db.r5.2xlarge" : "db.t3.medium",
allocatedStorage: isProduction ? 100 : 20,
multiAz: isProduction,
backupRetentionPeriod: isProduction ? 30 : 7,
tags: {
Environment: environment
}
});
// Create clusters with dynamic sizing
const workerCount = isProduction ? 5 : 2;
for (let i = 0; i < workerCount; i++) {
new aws.ec2.Instance(`worker-${i}`, {
ami: "ami-0c55b159cbfafe1f0",
instanceType: isProduction ? "c5.2xlarge" : "t3.medium",
// Additional configuration...
});
}
Conditional logic, loops, and dynamic resource creation become straightforward with programming languages.
Programming languages enable robust testing of infrastructure code:
# Python unit test for infrastructure components
import unittest
import pulumi
class InfrastructureTests(unittest.TestCase):
@pulumi.runtime.test
def test_s3_bucket_has_encryption(self):
"""Test that S3 buckets have encryption enabled."""
def check_encryption(args):
sse_config = args[0].server_side_encryption_configuration
return sse_config is not None
return pulumi.Output.all(data_bucket).apply(check_encryption)
@pulumi.runtime.test
def test_rds_is_encrypted(self):
"""Test that RDS instances are encrypted."""
def check_encryption(args):
return args[0].storage_encrypted
return pulumi.Output.all(database).apply(check_encryption)
This testability brings software engineering best practices to infrastructure code, improving reliability and confidence.
Pulumi supports all major cloud providers (AWS, Azure, Google Cloud, Kubernetes) and many others, with a consistent programming model across them:
// Multi-cloud infrastructure in TypeScript
import * as aws from "@pulumi/aws";
import * as azure from "@pulumi/azure-native";
import * as gcp from "@pulumi/gcp";
// AWS resources
const awsBucket = new aws.s3.Bucket("aws-data");
// Azure resources
const azureStorageAccount = new azure.storage.StorageAccount("azdata", {
resourceGroupName: resourceGroup.name,
sku: {
name: azure.storage.SkuName.Standard_LRS,
},
kind: azure.storage.Kind.StorageV2,
});
// GCP resources
const gcpBucket = new gcp.storage.Bucket("gcp-data");
// Export the endpoints for each storage service
export const awsEndpoint = awsBucket.websiteEndpoint;
export const azureEndpoint = azureStorageAccount.primaryEndpoints.blob;
export const gcpEndpoint = gcpBucket.url;
This multi-cloud capability is increasingly important as organizations adopt best-of-breed services across providers.
For data engineering teams, Pulumi offers specific advantages:
# Python code for a comprehensive data lake on AWS
import pulumi
import pulumi_aws as aws
# Core data lake storage with partitions
data_lake_bucket = aws.s3.Bucket("data-lake",
cors_rules=[{
"allowed_headers": ["*"],
"allowed_methods": ["GET", "PUT", "POST"],
"allowed_origins": ["*"],
"max_age_seconds": 3000
}],
lifecycle_rules=[
# Archive old data to save costs
{
"enabled": True,
"id": "archive-rule",
"prefix": "raw/",
"tags": {
"archived": "false"
},
"transition": [{
"days": 90,
"storage_class": "GLACIER"
}]
}
]
)
# Create logical partitions for the data lake
zones = ["raw", "trusted", "curated", "consumption"]
for zone in zones:
aws.s3.BucketObject(
f"{zone}-zone-prefix",
bucket=data_lake_bucket.id,
key=f"{zone}/",
content_type="application/directory"
)
# Set up AWS Glue Catalog for the data
glue_database = aws.glue.CatalogDatabase("data-catalog",
description="Data lake catalog"
)
# Create crawlers for each zone
for zone in zones:
# IAM role for the crawler
crawler_role = aws.iam.Role(f"{zone}-crawler-role",
assume_role_policy=json.dumps({
"Version": "2012-10-17",
"Statement": [{
"Action": "sts:AssumeRole",
"Principal": {
"Service": "glue.amazonaws.com"
},
"Effect": "Allow"
}]
})
)
# Attach policies
aws.iam.RolePolicyAttachment(f"{zone}-glue-service",
role=crawler_role.name,
policy_arn="arn:aws:iam::aws:policy/service-role/AWSGlueServiceRole"
)
# S3 access policy
aws.iam.RolePolicy(f"{zone}-s3-access",
role=crawler_role.name,
policy=data_lake_bucket.arn.apply(lambda arn: json.dumps({
"Version": "2012-10-17",
"Statement": [{
"Effect": "Allow",
"Action": [
"s3:GetObject",
"s3:ListBucket"
],
"Resource": [
arn,
f"{arn}/{zone}/*"
]
}]
}))
)
# Create the crawler
aws.glue.Crawler(f"{zone}-crawler",
database_name=glue_database.name,
role=crawler_role.arn,
s3_targets=[{
"path": pulumi.Output.concat("s3://", data_lake_bucket.id, "/", zone, "/")
}],
schedule="cron(0 0 * * ? *)" # Run daily at midnight
)
# Export the bucket name
pulumi.export("data_lake_bucket", data_lake_bucket.id)
pulumi.export("glue_database", glue_database.name)
This example demonstrates defining a complete data lake architecture with storage, partitioning, and catalog discovery.
// TypeScript example of a Spark cluster on AWS EMR
import * as aws from "@pulumi/aws";
import * as pulumi from "@pulumi/pulumi";
const config = new pulumi.Config();
const environment = config.require("environment");
// Define scaling based on environment
const instanceCounts = {
"development": { master: 1, core: 3, task: 0 },
"staging": { master: 1, core: 5, task: 0 },
"production": { master: 1, core: 10, task: 5 }
};
const scaling = instanceCounts[environment] || instanceCounts.development;
// Create service role for EMR
const emrServiceRole = new aws.iam.Role("emr-service-role", {
assumeRolePolicy: JSON.stringify({
Version: "2008-10-17",
Statement: [{
Effect: "Allow",
Principal: {
Service: "elasticmapreduce.amazonaws.com"
},
Action: "sts:AssumeRole"
}]
})
});
new aws.iam.RolePolicyAttachment("emr-service-policy", {
role: emrServiceRole.name,
policyArn: "arn:aws:iam::aws:policy/service-role/AmazonElasticMapReduceRole"
});
// Create instance profile for EMR instances
const emrInstanceRole = new aws.iam.Role("emr-instance-role", {
assumeRolePolicy: JSON.stringify({
Version: "2008-10-17",
Statement: [{
Effect: "Allow",
Principal: {
Service: "ec2.amazonaws.com"
},
Action: "sts:AssumeRole"
}]
})
});
new aws.iam.RolePolicyAttachment("emr-instance-profile-policy", {
role: emrInstanceRole.name,
policyArn: "arn:aws:iam::aws:policy/service-role/AmazonElasticMapReduceforEC2Role"
});
const emrInstanceProfile = new aws.iam.InstanceProfile("emr-instance-profile", {
role: emrInstanceRole.name
});
// Create the EMR cluster
const cluster = new aws.emr.Cluster("data-processing-cluster", {
applications: ["Spark", "Hive", "Presto"],
releaseLabel: "emr-6.6.0",
serviceRole: emrServiceRole.name,
instances: {
masterInstanceType: "m5.xlarge",
coreInstanceType: "r5.2xlarge",
coreInstanceCount: scaling.core,
taskInstanceType: "c5.2xlarge",
taskInstanceCount: scaling.task,
ec2AttributesInstanceProfile: emrInstanceProfile.name
},
configurations: JSON.stringify([
{
Classification: "spark-defaults",
Properties: {
"spark.dynamicAllocation.enabled": "true",
"spark.executor.instances": "0"
}
}
]),
tags: {
Environment: environment,
Name: `data-processing-${environment}`,
ManagedBy: "Pulumi"
}
});
// Export the cluster details
export const clusterId = cluster.id;
export const masterPublicDns = cluster.masterPublicDns;
This Spark cluster configuration showcases environment-specific scaling and proper IAM setup.
Combining storage, processing, and orchestration:
# Python code for a complete data pipeline
import pulumi
import pulumi_aws as aws
import json
# Create a VPC for isolation
vpc = aws.ec2.Vpc("data-vpc",
cidr_block="10.0.0.0/16",
enable_dns_hostnames=True,
enable_dns_support=True,
tags={
"Name": "data-processing-vpc"
}
)
# Create subnets across AZs
private_subnets = []
public_subnets = []
for i, az in enumerate(["us-east-1a", "us-east-1b", "us-east-1c"]):
public_subnet = aws.ec2.Subnet(f"public-{i}",
vpc_id=vpc.id,
cidr_block=f"10.0.{i}.0/24",
availability_zone=az,
map_public_ip_on_launch=True,
tags={
"Name": f"data-public-{az}"
}
)
public_subnets.append(public_subnet)
private_subnet = aws.ec2.Subnet(f"private-{i}",
vpc_id=vpc.id,
cidr_block=f"10.0.{i+100}.0/24",
availability_zone=az,
tags={
"Name": f"data-private-{az}"
}
)
private_subnets.append(private_subnet)
# Create Internet Gateway for public access
igw = aws.ec2.InternetGateway("igw",
vpc_id=vpc.id,
tags={
"Name": "data-igw"
}
)
# Create NAT Gateway for private subnet outbound traffic
eip = aws.ec2.Eip("nat-eip")
nat_gateway = aws.ec2.NatGateway("nat",
allocation_id=eip.id,
subnet_id=public_subnets[0].id,
tags={
"Name": "data-nat"
}
)
# Route tables
public_rt = aws.ec2.RouteTable("public-rt",
vpc_id=vpc.id,
routes=[
{
"cidr_block": "0.0.0.0/0",
"gateway_id": igw.id
}
],
tags={
"Name": "data-public-rt"
}
)
private_rt = aws.ec2.RouteTable("private-rt",
vpc_id=vpc.id,
routes=[
{
"cidr_block": "0.0.0.0/0",
"nat_gateway_id": nat_gateway.id
}
],
tags={
"Name": "data-private-rt"
}
)
# Associate route tables with subnets
for i, subnet in enumerate(public_subnets):
aws.ec2.RouteTableAssociation(f"public-rta-{i}",
subnet_id=subnet.id,
route_table_id=public_rt.id
)
for i, subnet in enumerate(private_subnets):
aws.ec2.RouteTableAssociation(f"private-rta-{i}",
subnet_id=subnet.id,
route_table_id=private_rt.id
)
# S3 buckets for data storage
raw_bucket = aws.s3.Bucket("raw-data",
versioning={
"enabled": True
},
server_side_encryption_configuration={
"rule": {
"apply_server_side_encryption_by_default": {
"sse_algorithm": "AES256"
}
}
}
)
processed_bucket = aws.s3.Bucket("processed-data",
versioning={
"enabled": True
},
server_side_encryption_configuration={
"rule": {
"apply_server_side_encryption_by_default": {
"sse_algorithm": "AES256"
}
}
}
)
# Create an Amazon MSK (Kafka) cluster
msk_cluster = aws.msk.Cluster("data-streaming",
kafka_version="2.8.1",
number_of_broker_nodes=3,
broker_node_group_info={
"instance_type": "kafka.m5.large",
"client_subnets": [s.id for s in private_subnets],
"storage_info": {
"ebs_storage_info": {
"volume_size": 100
}
},
"security_groups": [sg.id]
},
encryption_info={
"encryption_in_transit": {
"client_broker": "TLS",
"in_cluster": True
}
}
)
# Set up Airflow for orchestration
airflow_role = aws.iam.Role("airflow-role",
assume_role_policy=json.dumps({
"Version": "2012-10-17",
"Statement": [{
"Effect": "Allow",
"Principal": {
"Service": "airflow.amazonaws.com"
},
"Action": "sts:AssumeRole"
}]
})
)
# Attach policies for Airflow
for policy in ["AmazonS3FullAccess", "AmazonMSKFullAccess"]:
aws.iam.RolePolicyAttachment(f"airflow-{policy}",
role=airflow_role.name,
policy_arn=f"arn:aws:iam::aws:policy/{policy}"
)
# Create the MWAA (Managed Workflows for Apache Airflow) environment
airflow_environment = aws.mwaa.Environment("data-orchestration",
airflow_configuration_options={
"core.default_timezone": "utc",
"scheduler.min_file_process_interval": "30"
},
dag_s3_path="dags",
execution_role_arn=airflow_role.arn,
source_bucket_arn=processed_bucket.arn,
logging_configuration={
"dag_processing_logs": {
"enabled": True,
"log_level": "INFO"
},
"scheduler_logs": {
"enabled": True,
"log_level": "INFO"
},
"task_logs": {
"enabled": True,
"log_level": "INFO"
},
"webserver_logs": {
"enabled": True,
"log_level": "INFO"
},
"worker_logs": {
"enabled": True,
"log_level": "INFO"
}
},
network_configuration={
"security_group_ids": [sg.id],
"subnet_ids": [s.id for s in private_subnets]
}
)
# Export key resources
pulumi.export("vpc_id", vpc.id)
pulumi.export("raw_bucket", raw_bucket.id)
pulumi.export("processed_bucket", processed_bucket.id)
pulumi.export("msk_bootstrap_brokers_tls", msk_cluster.bootstrap_brokers_tls)
pulumi.export("airflow_webserver", airflow_environment.webserver_url)
This comprehensive pipeline example includes networking, storage, streaming, and orchestration—all defined as code.
Understanding Pulumi’s architecture helps explain its capabilities:
- CLI: The command-line interface for creating, deploying, and managing infrastructure
- Language SDKs: Libraries for Python, TypeScript/JavaScript, Go, C#, and other languages that expose cloud resources as classes/objects
- State Management: Service that tracks which resources have been created and their current configuration (either Pulumi Service or self-hosted)
- Resource Providers: Plugins that know how to create resources in specific cloud platforms
At the core of Pulumi is its resource model, which works across languages:
# Python
bucket = aws.s3.Bucket("my-bucket", versioning=True)
// TypeScript
const bucket = new aws.s3.Bucket("my-bucket", { versioning: true });
// Go
bucket, err := s3.NewBucket(ctx, "my-bucket", &s3.BucketArgs{
Versioning: pulumi.Bool(true),
})
// C#
var bucket = new Aws.S3.Bucket("my-bucket", new Aws.S3.BucketArgs
{
Versioning = true
});
This consistent model makes Pulumi approachable regardless of your language preference.
As you scale your use of Pulumi, several advanced techniques become valuable:
Component resources enable you to create high-level abstractions:
// TypeScript example of a component resource
import * as pulumi from "@pulumi/pulumi";
import * as aws from "@pulumi/aws";
export interface DataPipelineArgs {
environment: string;
region: string;
vpcId: pulumi.Input<string>;
subnetIds: pulumi.Input<string>[];
}
export class DataPipeline extends pulumi.ComponentResource {
public readonly rawBucket: aws.s3.Bucket;
public readonly processedBucket: aws.s3.Bucket;
public readonly dataWarehouse: aws.redshift.Cluster;
constructor(name: string, args: DataPipelineArgs, opts?: pulumi.ComponentResourceOptions) {
super("custom:resource:DataPipeline", name, args, opts);
// Create raw data bucket
this.rawBucket = new aws.s3.Bucket(`${name}-raw`, {
versioning: {
enabled: true,
},
serverSideEncryptionConfiguration: {
rule: {
applyServerSideEncryptionByDefault: {
sseAlgorithm: "AES256",
},
},
},
tags: {
Environment: args.environment,
Component: "DataPipeline",
},
}, { parent: this });
// Create processed data bucket
this.processedBucket = new aws.s3.Bucket(`${name}-processed`, {
versioning: {
enabled: true,
},
serverSideEncryptionConfiguration: {
rule: {
applyServerSideEncryptionByDefault: {
sseAlgorithm: "AES256",
},
},
},
tags: {
Environment: args.environment,
Component: "DataPipeline",
},
}, { parent: this });
// Create security group for Redshift
const redshiftSg = new aws.ec2.SecurityGroup(`${name}-redshift-sg`, {
vpcId: args.vpcId,
description: "Security group for Redshift cluster",
ingress: [
{
protocol: "tcp",
fromPort: 5439,
toPort: 5439,
cidrBlocks: ["10.0.0.0/16"],
},
],
egress: [
{
protocol: "-1",
fromPort: 0,
toPort: 0,
cidrBlocks: ["0.0.0.0/0"],
},
],
tags: {
Environment: args.environment,
Component: "DataPipeline",
},
}, { parent: this });
// Create subnet group for Redshift
const subnetGroup = new aws.redshift.SubnetGroup(`${name}-subnet-group`, {
subnetIds: args.subnetIds,
tags: {
Environment: args.environment,
Component: "DataPipeline",
},
}, { parent: this });
// Create Redshift cluster for data warehousing
this.dataWarehouse = new aws.redshift.Cluster(`${name}-warehouse`, {
clusterType: args.environment === "production" ? "multi-node" : "single-node",
numberOfNodes: args.environment === "production" ? 3 : 1,
nodeType: args.environment === "production" ? "ra3.xlplus" : "dc2.large",
masterUsername: "admin",
masterPassword: "YourSecurePassword123!", // In production, use secrets management
databaseName: "warehouse",
vpcSecurityGroupIds: [redshiftSg.id],
clusterSubnetGroupName: subnetGroup.name,
encrypted: true,
skipFinalSnapshot: args.environment !== "production",
tags: {
Environment: args.environment,
Component: "DataPipeline",
},
}, { parent: this });
// Register outputs
this.registerOutputs({
rawBucketName: this.rawBucket.id,
processedBucketName: this.processedBucket.id,
warehouseEndpoint: this.dataWarehouse.endpoint,
});
}
}
This pattern enables creating reusable, composable infrastructure components.
The CrossGuard feature enables policy enforcement:
// Policy that ensures all S3 buckets are encrypted
import { PolicyPack, validateResourceOfType } from "@pulumi/policy";
import { Bucket } from "@pulumi/aws/s3";
new PolicyPack("aws-s3", {
policies: [{
name: "s3-encryption-required",
description: "Ensures that all S3 buckets have encryption enabled",
enforcementLevel: "mandatory",
validateResource: validateResourceOfType(Bucket, (bucket, args, reportViolation) => {
if (!bucket.serverSideEncryptionConfiguration) {
reportViolation("All S3 buckets must have server-side encryption configured");
}
}),
}],
});
This approach enforces security and compliance standards automatically during deployments.
For truly custom resources, dynamic providers enable integration with any API:
// Dynamic provider example for a custom API
import * as pulumi from "@pulumi/pulumi";
// Define the shape of inputs and outputs
interface MyCustomResourceInputs {
name: string;
property1: string;
property2: number;
}
interface MyCustomResourceOutputs {
id: string;
endpoint: string;
status: string;
}
// Create a dynamic provider
class MyCustomResourceProvider implements pulumi.dynamic.ResourceProvider {
async create(inputs: MyCustomResourceInputs): Promise<pulumi.dynamic.CreateResult> {
// Call an external API to create the resource
const response = await fetch("https://api.example.com/resources", {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({
name: inputs.name,
prop1: inputs.property1,
prop2: inputs.property2,
}),
});
const result = await response.json();
// Return the resource ID and properties
return {
id: result.id,
outs: {
id: result.id,
endpoint: result.endpoint,
status: result.status,
},
};
}
async delete(id: string, props: MyCustomResourceOutputs): Promise<void> {
// Delete the resource when the stack is destroyed
await fetch(`https://api.example.com/resources/${id}`, {
method: "DELETE",
});
}
}
// Create a dynamic resource class
export class MyCustomResource extends pulumi.dynamic.Resource {
public readonly endpoint: pulumi.Output<string>;
public readonly status: pulumi.Output<string>;
constructor(name: string, args: MyCustomResourceInputs, opts?: pulumi.CustomResourceOptions) {
super(
new MyCustomResourceProvider(),
name,
{
...args,
endpoint: undefined,
status: undefined,
},
opts
);
}
}
This capability allows Pulumi to work with services that don’t have official providers.
Based on industry experience, here are key practices for effective Pulumi usage:
Organize your projects for maintainability:
project/
├── Pulumi.yaml # Project definition
├── Pulumi.dev.yaml # Dev environment configuration
├── Pulumi.prod.yaml # Production environment configuration
├── index.ts # Main entry point
├── tsconfig.json # TypeScript configuration
├── package.json # Dependencies
├── components/ # Reusable components
│ ├── networking.ts
│ ├── storage.ts
│ └── compute.ts
├── resources/ # Resource definitions
│ ├── database.ts
│ ├── pipelines.ts
│ └── monitoring.ts
└── config/ # Configuration utilities
└── helpers.ts
This structure promotes modularity and reuse across deployments.
Handle sensitive information securely:
// Using Pulumi's built-in secrets
const config = new pulumi.Config();
const dbPassword = config.requireSecret("dbPassword");
const database = new aws.rds.Instance("database", {
// Other properties...
password: dbPassword,
});
// Using external secret management
const secretsManager = new aws.secretsmanager.Secret("db-credentials");
const secretVersion = new aws.secretsmanager.SecretVersion("db-credentials-version", {
secretId: secretsManager.id,
secretString: JSON.stringify({
username: "admin",
password: config.requireSecret("dbPassword"),
}),
});
const database = new aws.rds.Instance("database", {
// Other properties...
username: "admin",
password: secretVersion.secretString.apply(s => JSON.parse(s).password),
});
This ensures sensitive data is handled securely throughout the deployment lifecycle.
Implement testing for your infrastructure:
// Example of testing infrastructure code with Mocha
import * as pulumi from "@pulumi/pulumi";
import * as aws from "@pulumi/aws";
import { expect } from "chai";
import * as infra from "../index";
pulumi.runtime.setMocks({
newResource: function(type, name, inputs) {
return {
id: `${name}-id`,
state: inputs,
};
},
call: function(token, args, provider) {
return args;
},
});
describe("Infrastructure", function() {
it("creates a properly configured S3 bucket", function(done) {
const dataBucket = infra.dataBucket;
pulumi.all([
dataBucket.versioning,
dataBucket.serverSideEncryptionConfiguration,
]).apply(([versioning, encryption]) => {
try {
expect(versioning.enabled).to.equal(true);
expect(encryption.rule.applyServerSideEncryptionByDefault.sseAlgorithm).to.equal("AES256");
done();
} catch (e) {
done(e);
}
});
});
it("configures the data warehouse with encryption", function(done) {
pulumi.all([infra.dataWarehouse.encrypted]).apply(([encrypted]) => {
try {
expect(encrypted).to.equal(true);
done();
} catch (e) {
done(e);
}
});
});
it("configures the data warehouse with encryption", function(done) {
pulumi.all([infra.dataWarehouse.encrypted]).apply(([encrypted]) => {
try {
expect(encrypted).to.equal(true);
done();
} catch (e) {
done(e);
}
});
});
});
Testing improves reliability and gives you confidence in your infrastructure changes.
Integrate Pulumi into your CI/CD pipelines:
# GitHub Actions workflow for Pulumi
name: Infrastructure CI/CD
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
preview:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: 16.x
- name: Install dependencies
run: npm install
- uses: pulumi/actions@v3
with:
command: preview
stack-name: dev
comment-on-pr: true
env:
PULUMI_ACCESS_TOKEN: ${{ secrets.PULUMI_ACCESS_TOKEN }}
update:
runs-on: ubuntu-latest
if: github.event_name == 'push' && github.ref == 'refs/heads/main'
needs: preview
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: 16.x
- name: Install dependencies
run: npm install
- uses: pulumi/actions@v3
with:
command: up
stack-name: dev
env:
PULUMI_ACCESS_TOKEN: ${{ secrets.PULUMI_ACCESS_TOKEN }}
This approach automates infrastructure updates while maintaining safety through previews.
For complex infrastructures, use a strategic organization:
organization/
├── platform/ # Core shared infrastructure
│ ├── networking/ # VPCs, subnets, etc.
│ ├── security/ # IAM, security groups, etc.
│ └── monitoring/ # Logging, monitoring, alerts
├── data-platform/ # Data infrastructure
│ ├── storage/ # Data lake, warehouses
│ ├── processing/ # Spark, EMR, etc.
│ └── analytics/ # BI tools, dashboards
└── applications/ # Application infrastructure
├── service-a/
├── service-b/
└── service-c/
This structure allows different teams to manage their areas while sharing core components.
Understanding how Pulumi compares to alternatives helps determine when to use it:
Feature | Pulumi | Terraform | AWS CloudFormation | Serverless Framework |
---|---|---|---|---|
Language | Multiple general purpose (TS, Python, Go, C#, etc.) | HCL (domain-specific) | JSON/YAML (declarative) | YAML + JavaScript |
Programming Model | Imperative and declarative | Primarily declarative | Declarative | Declarative with hooks |
State Management | Service or self-hosted backend | State files (local or remote) | Managed by cloud provider | CloudFormation or proprietary |
Extensibility | Custom resources with any API | Provider plugins | Limited custom resources | Plugins and hooks |
Multi-cloud | Native, unified API | Multiple providers | Provider-specific | Limited via plugins |
Learning Curve | Depends on language familiarity | Moderate (new DSL) | Moderate (JSON/YAML) | Low for JS developers |
Testing | Standard language testing tools | Custom testing tools | Limited | Limited |
Pulumi’s key advantages include using familiar languages, strong typing, and software engineering patterns. It’s particularly well-suited for teams that already have software development expertise.
For organizations looking to adopt Pulumi, a phased approach works best:
Start with a single, non-critical component:
- Choose a well-understood service (e.g., S3 buckets, EC2 instances)
- Define it in Pulumi using your preferred language
- Deploy to a development or staging environment
- Validate the deployment works as expected
- Practice updates and rollbacks
Gradually expand to more complex infrastructure:
- Define networking components (VPCs, subnets, security groups)
- Add data services (databases, data lakes, processing frameworks)
- Implement deployment pipelines
- Create reusable components for common patterns
Scale to full infrastructure management:
- Develop standardized modules and components
- Implement governance through policy as code
- Integrate with existing CI/CD systems
- Train teams on infrastructure as code principles
- Migrate legacy infrastructure incrementally
As infrastructure as code continues to evolve, several trends are shaping Pulumi’s future:
Pulumi continues to expand support for cloud services and platforms, with same-day support for new AWS, Azure, and GCP services.
The integration of AI capabilities is beginning to transform how infrastructure is defined:
Pulumi AI> Create an S3 bucket with versioning enabled and a lifecycle policy to archive objects after 90 days
# Generated Python code:
import pulumi
import pulumi_aws as aws
bucket = aws.s3.Bucket("my-bucket",
versioning=aws.s3.BucketVersioningArgs(
enabled=True,
),
lifecycle_rules=[aws.s3.BucketLifecycleRuleArgs(
enabled=True,
transitions=[aws.s3.BucketLifecycleRuleTransitionArgs(
days=90,
storage_class="GLACIER",
)],
)],
)
pulumi.export("bucket_name", bucket.id)
These AI capabilities promise to make infrastructure definition more accessible and efficient.
As security concerns grow, Pulumi’s policy as code features continue to expand, with enhanced scanning, drift detection, and automated remediation.
The Pulumi Registry is growing rapidly, offering pre-built components for common infrastructure patterns and specialized use cases.
Pulumi represents a significant evolution in infrastructure as code, bringing the full power of programming languages to cloud infrastructure management. By treating infrastructure as software, it enables teams to apply established software engineering practices to infrastructure deployment—improving reliability, maintainability, and deployment velocity.
For data engineering teams in particular, Pulumi offers compelling advantages in defining and managing complex data platforms. The ability to use familiar languages, create abstractions, implement testing, and integrate with existing toolchains makes it an increasingly popular choice for modern data infrastructure.
Whether you’re building a data lake on AWS, a processing pipeline on Google Cloud, or an analytics platform spanning multiple clouds, Pulumi provides the flexibility and power to define your infrastructure with confidence and precision. As cloud architectures continue to grow in complexity, tools like Pulumi that enable a software engineering approach to infrastructure will only become more valuable.
Keywords: Pulumi, Infrastructure as Code, IaC, programming languages, cloud infrastructure, Python, TypeScript, Go, C#, cloud automation, multi-cloud, AWS, Azure, Google Cloud, configuration management, DevOps, DataOps, cloud native, software engineering
#Pulumi #InfrastructureAsCode #IaC #CloudAutomation #DevOps #DataEngineering #ProgrammaticInfrastructure #MultiCloud #AWS #Azure #GoogleCloud #Python #TypeScript #CloudNative #DataOps #ComponentResources #SoftwareEngineering