Dask
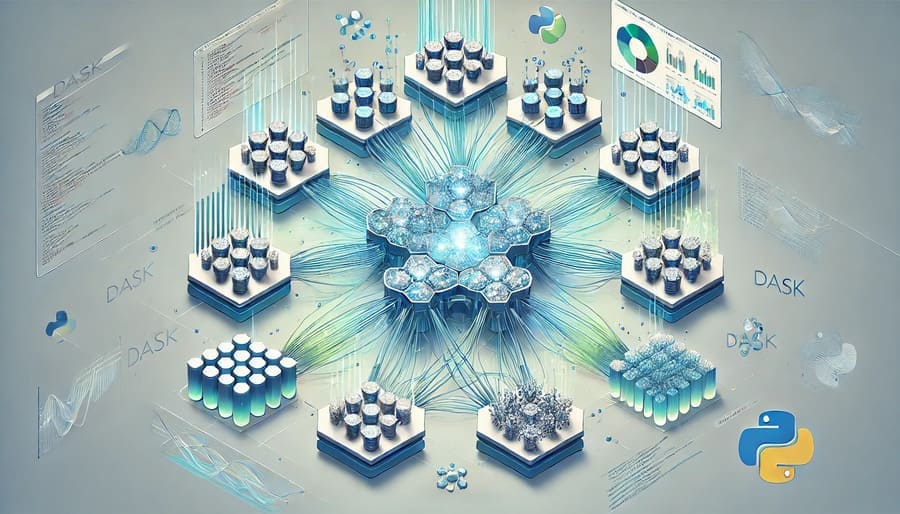
In an era where data science projects routinely push against the limits of single-machine computing, Dask has emerged as a game-changing solution that scales Python’s most beloved data science tools to handle massive datasets across multiple cores and machines. This comprehensive guide explores how Dask is revolutionizing parallel computing while maintaining the familiar Python ecosystem that data professionals love.
Dask is an open-source parallel computing library that scales Python’s data science ecosystem – particularly NumPy, Pandas, and Scikit-learn – to distributed computing environments. Created to bridge the gap between small-scale and big data processing, Dask allows data scientists to continue using familiar tools while seamlessly scaling to larger-than-memory datasets and multi-core or multi-node processing.
import dask.dataframe as dd
import dask.array as da
# Create a Dask DataFrame from CSV files that won't fit in memory
df = dd.read_csv('s3://my-bucket/huge-dataset-*.csv')
# Perform familiar pandas-like operations
result = df.groupby('category').agg({'value': ['mean', 'sum']})
# Trigger computation only when needed
result.compute()
Dask’s architecture consists of three main layers, each serving a specific purpose in the parallel computing ecosystem:
Dask provides parallel versions of Python’s most popular data structures:
- Dask Array: Parallel NumPy arrays for numerical computing
- Dask DataFrame: Parallel Pandas DataFrames for tabular data
- Dask Bag: Parallel Python lists for semi-structured data
- Dask Delayed: A simple interface for parallelizing custom algorithms
Each collection implements a familiar API, making the transition from single-machine code to parallel processing remarkably smooth.
# Dask Array example - Working with larger-than-memory arrays
import dask.array as da
# Create a 10TB array that won't fit in memory
x = da.random.random((1000000, 1000000, 10), chunks=(10000, 10000, 5))
# Perform NumPy-like computations
y = x.mean(axis=0)
z = y[::100, ::100]
# Compute only what's needed
result = z.compute()
Under the hood, Dask operations create task graphs—directed acyclic graphs (DAGs) where nodes represent tasks and edges represent dependencies. These graphs are then executed by Dask’s schedulers:
- Single-machine schedulers: For local parallel processing
- Distributed scheduler: For multi-machine clusters
import dask
from dask.distributed import Client
# Connect to a Dask cluster (or create a local one)
client = Client('scheduler-address:8786') # For existing cluster
# client = Client() # For local cluster
# Visualize the task graph
dask.visualize(result) # Creates a task graph visualization
Dask’s schedulers dynamically execute task graphs, adapting to:
- Available resources (memory, CPU, network)
- Data locality
- Task dependencies
This adaptive approach minimizes unnecessary computation and data movement, optimizing performance even for complex workflows.
What sets Dask apart from other big data tools is its seamless integration with the existing Python ecosystem:
# Pandas code
import pandas as pd
df = pd.read_csv('data.csv')
result = df.groupby('column').mean()
# Equivalent Dask code for large datasets
import dask.dataframe as dd
df = dd.read_csv('huge-data-*.csv')
result = df.groupby('column').mean().compute()
This familiar interface dramatically reduces the learning curve for data scientists transitioning to distributed computing.
Dask offers multiple deployment options to match your resource needs:
# Local scaling on a single machine
from dask.distributed import Client
client = Client(n_workers=8, threads_per_worker=4) # Utilize 8 cores with 4 threads each
# Cluster scaling with existing job schedulers
from dask_jobqueue import SLURMCluster
cluster = SLURMCluster(cores=8, memory="16GB")
cluster.scale(jobs=10) # Scale to 10 nodes
client = Client(cluster)
# Cloud scaling
from dask_kubernetes import KubeCluster
cluster = KubeCluster()
cluster.adapt(minimum=2, maximum=20) # Auto-scale between 2-20 workers
client = Client(cluster)
Dask uses lazy evaluation to optimize performance:
- Operations are recorded but not executed immediately
- Task graphs are built representing the full computation
- Optimizations are applied to the entire graph
- Computation is triggered only when results are needed
# Building a computation without executing
df = dd.read_csv('large-dataset-*.csv')
high_value = df[df['value'] > 100]
result = high_value.groupby('category').mean()
# The computation only happens when we call compute()
final_result = result.compute()
This approach allows Dask to:
- Minimize unnecessary computation
- Optimize memory usage
- Reduce data movement
- Execute tasks in parallel when possible
Financial institutions and IoT applications regularly work with time-series datasets that exceed single-machine capabilities:
import dask.dataframe as dd
# Load several years of minute-level stock data
stocks = dd.read_parquet('s3://bucket/stocks/year=*/month=*/*.parquet')
# Resample to daily frequency and calculate metrics
daily = stocks.groupby('symbol').resample('1D').agg({
'open': 'first',
'high': 'max',
'low': 'min',
'close': 'last',
'volume': 'sum'
})
# Calculate 30-day rolling average for each symbol
rolling_avg = daily['close'].rolling(window=30).mean()
# Trigger computation and save results
rolling_avg.to_frame().compute().to_csv('rolling_averages.csv')
Data scientists can leverage Dask to scale machine learning workflows:
import dask.array as da
import dask.dataframe as dd
from dask_ml.model_selection import train_test_split
from dask_ml.linear_model import LogisticRegression
# Load and preprocess data
df = dd.read_parquet('s3://bucket/large-dataset/*.parquet')
X = df.drop('target', axis=1)
y = df['target']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Train model (distributed training)
model = LogisticRegression()
model.fit(X_train, y_train)
# Evaluate model
score = model.score(X_test, y_test)
predictions = model.predict(X_test)
Dask arrays excel at processing large multi-dimensional datasets like satellite imagery:
import dask.array as da
import rioxarray
# Open a collection of satellite images
satellite_data = rioxarray.open_mfdataset(
'sentinel-2/*.tif',
chunks={'x': 1000, 'y': 1000, 'band': 1}
)
# Calculate vegetation index (NDVI)
red = satellite_data.sel(band='B4')
nir = satellite_data.sel(band='B8')
ndvi = (nir - red) / (nir + red)
# Apply threshold and calculate statistics
vegetation_mask = ndvi > 0.4
vegetation_coverage = vegetation_mask.sum() / vegetation_mask.size
# Compute result
result = vegetation_coverage.compute()
print(f"Vegetation coverage: {result * 100:.2f}%")
Dask simplifies complex data pipelines with its ability to handle diverse data formats and operations:
import dask.dataframe as dd
import dask.bag as db
from dask.distributed import Client
client = Client() # Start local cluster
# Extract: Load data from multiple sources
orders = dd.read_csv('s3://bucket/orders/*.csv')
customers = dd.read_json('s3://bucket/customers/*.json')
logs = db.read_text('s3://bucket/logs/*.log').map(parse_log)
# Transform: Join and process data
enriched_orders = orders.merge(
customers[['customer_id', 'segment']],
on='customer_id'
)
daily_metrics = enriched_orders.groupby(['date', 'segment']).agg({
'order_id': 'count',
'value': 'sum'
})
# Additional transformations with logs
error_rates = logs.filter(lambda x: x['level'] == 'ERROR') \
.map(lambda x: (x['date'], 1)) \
.reduction(perkey=True, chunk=lambda s: s.sum(),
aggregate=lambda s: s.sum())
# Load: Store results
daily_metrics.to_parquet('s3://bucket/analytics/daily-metrics/',
partition_on=['date'])
client.compute(error_rates.to_textfiles('s3://bucket/analytics/errors-*.json'))
For complex algorithms not covered by high-level collections, dask.delayed
provides a way to parallelize arbitrary code:
import dask
import dask.array as da
from scipy import signal
@dask.delayed
def process_chunk(chunk, kernel):
# CPU-intensive image processing on a single chunk
return signal.convolve2d(chunk, kernel)
# Create a large dask array
large_image = da.random.random((10000, 10000), chunks=(1000, 1000))
kernel = np.random.random((5, 5))
# Process each chunk in parallel
chunks = []
for i in range(10):
for j in range(10):
chunk = large_image.blocks[i, j]
# Convert dask array chunk to numpy array for processing
processed = process_chunk(chunk.compute(), kernel)
chunks.append(processed)
# Combine results
results = dask.compute(*chunks)
final_image = np.block([[results[i*10 + j] for j in range(10)] for i in range(10)])
Dask’s distributed scheduler supports advanced task management:
from dask.distributed import Client, worker_client
client = Client()
# Set custom task priorities
future1 = client.submit(slow_function, priority=10) # Higher priority
future2 = client.submit(slow_function, priority=5) # Lower priority
# Specify resource requirements
@dask.delayed
def gpu_task(data):
# Task that requires GPU
return process_on_gpu(data)
future3 = client.submit(gpu_task, data, resources={'GPU': 1})
# Dynamic adaptation to worker capabilities
def process_data_adaptively(df):
with worker_client() as client:
# Get worker's available resources
worker_info = client.scheduler_info()['workers']
this_worker = list(worker_info.values())[0]
available_memory = this_worker['memory']['available']
# Adjust processing based on available resources
if available_memory > 4e9: # More than 4GB available
return process_in_memory(df)
else:
return process_with_less_memory(df)
result = df.map_partitions(process_data_adaptively)
Fine-tuning Dask for optimal performance:
from dask.distributed import Client, performance_report
# Start client with tuned settings
client = Client(
n_workers=4,
threads_per_worker=8,
memory_limit='16GB',
dashboard_address=':8787'
)
# Generate performance report
with performance_report(filename="dask-report.html"):
# Adjust partition size for better performance
df = dd.read_csv('large-data-*.csv', blocksize='64MB')
# Repartition for more even distribution
df = df.repartition(npartitions=100)
# Persist frequently used data in memory
df = client.persist(df)
# Run computation
result = df.groupby('key').mean().compute()
Dask works seamlessly with the broader Python data science ecosystem:
import dask.array as da
import dask.dataframe as dd
import xarray as xr
import matplotlib.pyplot as plt
from sklearn.ensemble import RandomForestRegressor
from dask_ml.wrappers import ParallelPostFit
# Load climate data with xarray and dask
ds = xr.open_mfdataset('climate-data/temp_*.nc', chunks={'time': 10})
temps = ds['temperature'].values # Dask array
# Visualize with matplotlib (computes only what's needed for the plot)
plt.figure(figsize=(12, 6))
da.mean(temps, axis=(1, 2)).plot()
plt.title('Global Temperature Trends')
plt.savefig('temperature_trends.png')
# Integrate with scikit-learn
X = dd.read_parquet('features/*.parquet')
y = dd.read_parquet('targets/*.parquet')
# Use scikit-learn with Dask for parallel prediction
rf = RandomForestRegressor(n_estimators=100)
parallel_rf = ParallelPostFit(rf)
parallel_rf.fit(X.compute(), y.compute()) # Train on in-memory data
# Parallel prediction on larger dataset
predictions = parallel_rf.predict(X)
Dask provides optimized connectors to popular data formats and storage systems:
import dask.dataframe as dd
import s3fs
import gcsfs
# Read optimized formats
parquet_df = dd.read_parquet(
's3://bucket/data.parquet',
engine='pyarrow',
storage_options={'anon': False}
)
# Use cloud storage directly
s3 = s3fs.S3FileSystem(anon=False)
files = s3.glob('bucket/data/year=2023/month=*/*.parquet')
df = dd.read_parquet(files)
# Write with partitioning
df.to_parquet(
'gs://bucket/processed/',
partition_on=['date'],
engine='pyarrow',
storage_options={'token': 'google-credentials.json'}
)
Integrate Dask with workflow orchestrators and streaming systems:
# Airflow DAG using Dask
from airflow import DAG
from airflow.operators.python import PythonOperator
from datetime import datetime
def process_with_dask(ds, **kwargs):
from dask.distributed import Client
client = Client('dask-scheduler:8786')
# Process data for the current date
df = dd.read_parquet(f's3://bucket/data/date={ds}/')
result = df.groupby('category').mean().compute()
# Save results
result.to_csv(f's3://bucket/results/date={ds}/metrics.csv')
with DAG('dask_processing', start_date=datetime(2023, 1, 1), schedule_interval='@daily') as dag:
process_task = PythonOperator(
task_id='process_with_dask',
python_callable=process_with_dask
)
Optimize your Dask deployment for different environments:
- Single Machine Scaling
- Start with a local cluster for development
- Use processes for CPU-bound work, threads for IO-bound work
- Set memory limits appropriate for your machine
from dask.distributed import Client, LocalCluster
# For CPU-bound work
cluster = LocalCluster(
n_workers=8, # Use 8 separate processes
threads_per_worker=1, # Single thread per worker
memory_limit='4GB' # 4GB per worker
)
# For IO-bound work
cluster = LocalCluster(
n_workers=1, # Single process
threads_per_worker=16, # Multiple threads
memory_limit='16GB' # All memory in one process
)
client = Client(cluster)
- Cluster Scaling
- Adapt to existing infrastructure (SLURM, PBS, SGE)
- Implement auto-scaling based on workload
- Balance worker size vs. number of workers
# Kubernetes scaling
from dask_kubernetes import KubeCluster
cluster = KubeCluster(
pod_template='/path/to/pod-template.yaml'
)
cluster.adapt(minimum=5, maximum=100)
client = Client(cluster)
# Scale based on current workload
from distributed.diagnostics import progress
future = client.submit(big_computation)
progress(future) # Monitor progress
cluster.scale(20) # Manually scale to 20 workers when needed
Effective memory management is crucial for Dask performance:
import dask
import dask.dataframe as dd
from dask.distributed import Client, performance_report
# Configure global settings
dask.config.set({
'distributed.worker.memory.target': 0.6, # Target 60% memory usage
'distributed.worker.memory.spill': 0.7, # Spill to disk at 70%
'distributed.worker.memory.pause': 0.8, # Pause work at 80%
'distributed.worker.memory.terminate': 0.95 # Restart worker at 95%
})
client = Client()
# Minimize memory usage with smaller partitions for large datasets
df = dd.read_csv('huge-file.csv', blocksize='32MB')
# Use repartition to balance memory usage
df = df.repartition(partition_size='64MB')
# Explicitly clean up to manage memory
result = df.some_big_computation().compute()
del df
client.run(gc.collect) # Force garbage collection on all workers
Set up comprehensive monitoring for your Dask workflows:
from dask.distributed import Client
import time
client = Client('scheduler-address:8786')
# Access the dashboard
print(f"Dashboard available at: {client.dashboard_link}")
# Monitor cluster status
client.scheduler_info()
# Track specific computation
future = client.submit(slow_function, 100)
future.status # 'pending', 'running', 'finished', or 'error'
# Stream logs from workers
client.get_worker_logs()
# Debugging worker issues
def debug_worker_state():
import psutil
import os
return {
'memory': psutil.virtual_memory()._asdict(),
'cpu': psutil.cpu_percent(interval=0.1),
'open_files': len(psutil.Process().open_files())
}
diagnostics = client.run(debug_worker_state)
for worker, state in diagnostics.items():
print(f"Worker {worker}: {state}")
Dask has fundamentally transformed how data scientists approach large-scale computing problems by bringing the power of distributed computing to the familiar Python ecosystem. Its unique combination of flexible scaling, intuitive APIs, and integration with existing tools makes it an indispensable tool for modern data work.
As data continues to grow in volume and complexity, Dask’s importance will only increase. Recent developments point to an exciting future:
- Enhanced GPU support for accelerated computing
- Improved integration with cloud-native technologies
- Expanded machine learning capabilities
- Optimized performance for specific workloads
- Growing ecosystem of compatible tools and libraries
Whether you’re processing terabytes of financial data, training machine learning models on massive datasets, or analyzing complex scientific simulations, Dask provides the scalable foundation needed to tackle tomorrow’s data challenges while using the Python tools you already know and love today.
#Dask #ParallelComputing #DataScience #BigData #PythonScaling #DistributedComputing #DataEngineering #NumPy #Pandas #ScikitLearn #DataProcessing #ScalableComputing #PythonDataStack #LargeScaleData #ParallelProcessing #DataAnalysis #CloudComputing #MachineLearning #OpenSource #HighPerformanceComputing