Pandas
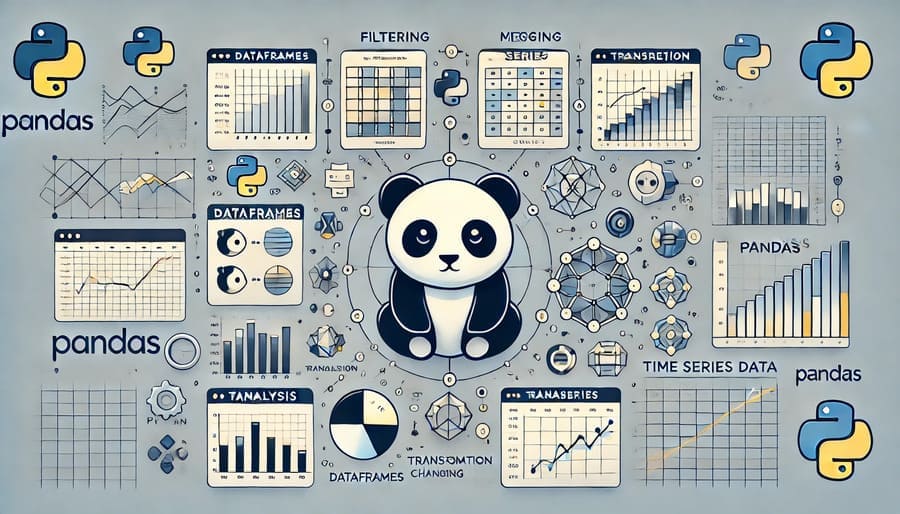
In the ever-expanding universe of data science tools, few libraries have revolutionized the way we work with data quite like Pandas. This powerful Python library has become indispensable for data scientists, analysts, and researchers worldwide, offering an intuitive and flexible framework for manipulating, analyzing, and visualizing structured data. This comprehensive guide explores Pandas’ capabilities, applications, and why it has become the backbone of data analysis in Python.
Created by Wes McKinney in 2008 while working at AQR Capital Management, Pandas (the name derived from “panel data,” an econometrics term) was born out of the need for a high-performance, flexible tool for data analysis in Python. McKinney required a tool that combined the statistical power of R with the general-purpose nature of Python, leading to the development of what would become one of the most important libraries in the data science ecosystem.
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Creating a simple DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emma'],
'Age': [25, 30, 35, 40, 45],
'Salary': [50000, 60000, 75000, 90000, 120000],
'Department': ['HR', 'IT', 'Finance', 'IT', 'Management']
}
df = pd.DataFrame(data)
print(df)
Pandas revolves around two primary data structures that make working with structured data intuitive and efficient:
The DataFrame is a two-dimensional, size-mutable, potentially heterogeneous tabular data structure with labeled axes (rows and columns). Think of it as a spreadsheet or SQL table, but with powerful manipulation capabilities.
# Different ways to create DataFrames
# From a dictionary
df1 = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c']
})
# From a list of dictionaries
df2 = pd.DataFrame([
{'A': 1, 'B': 'a'},
{'A': 2, 'B': 'b'},
{'A': 3, 'B': 'c'}
])
# From a NumPy array
df3 = pd.DataFrame(np.random.randn(3, 2), columns=['A', 'B'])
print("DataFrame info:")
print(df.info())
print("\nDataFrame statistics:")
print(df.describe())
A Series is a one-dimensional labeled array capable of holding any data type. It’s similar to a column in a DataFrame or a single-dimensional NumPy array but with an index.
# Creating Series objects
s1 = pd.Series([10, 20, 30, 40], index=['a', 'b', 'c', 'd'])
s2 = pd.Series({'a': 10, 'b': 20, 'c': 30, 'd': 40})
print("Series s1:")
print(s1)
print("\nAccessing elements:")
print(f"s1['a'] = {s1['a']}")
print(f"s1[0] = {s1[0]}")
Pandas excels at transforming and reshaping data, offering a wealth of methods for cleaning, filtering, and reorganizing datasets.
# Basic selection
print("Select a column:")
print(df['Name'])
# Multiple columns
print("\nSelect multiple columns:")
print(df[['Name', 'Salary']])
# Row selection with loc (label-based) and iloc (position-based)
print("\nSelect row by label:")
print(df.loc[2]) # Select the row with index 2
print("\nSelect row by position:")
print(df.iloc[2]) # Select the third row
# Conditional filtering
print("\nFilter by condition:")
high_salary = df[df['Salary'] > 70000]
print(high_salary)
# Complex conditions
print("\nComplex filtering:")
it_high_salary = df[(df['Department'] == 'IT') & (df['Salary'] > 60000)]
print(it_high_salary)
Missing data is a common challenge in real-world datasets, and Pandas provides robust tools for detection and treatment:
# Create data with missing values
df_missing = pd.DataFrame({
'A': [1, 2, np.nan, 4],
'B': [5, np.nan, np.nan, 8],
'C': [9, 10, 11, 12]
})
print("DataFrame with missing values:")
print(df_missing)
# Detect missing values
print("\nMissing value detection:")
print(df_missing.isna())
print(f"Total missing values: {df_missing.isna().sum().sum()}")
# Fill missing values
print("\nFill with zero:")
print(df_missing.fillna(0))
print("\nFill with forward fill:")
print(df_missing.fillna(method='ffill'))
print("\nFill with mean of each column:")
print(df_missing.fillna(df_missing.mean()))
# Drop rows with missing values
print("\nDrop rows with any missing values:")
print(df_missing.dropna())
print("\nDrop rows with all missing values:")
print(df_missing.dropna(how='all'))
Pandas provides powerful tools for transforming and reshaping data:
# Group by operations
print("Group by Department with mean aggregation:")
department_stats = df.groupby('Department').mean()
print(department_stats)
# Multiple aggregations
print("\nMultiple aggregations:")
grouped = df.groupby('Department').agg({
'Age': ['mean', 'min', 'max'],
'Salary': ['mean', 'min', 'max', 'count']
})
print(grouped)
# Pivot tables
sales_data = pd.DataFrame({
'Date': pd.date_range(start='2023-01-01', periods=10),
'Product': ['A', 'B', 'A', 'C', 'B', 'A', 'C', 'B', 'C', 'A'],
'Region': ['East', 'West', 'North', 'South', 'East', 'West', 'North', 'South', 'East', 'West'],
'Sales': [100, 200, 150, 300, 250, 180, 320, 270, 230, 190]
})
print("\nPivot table example:")
pivot = sales_data.pivot_table(
index='Region',
columns='Product',
values='Sales',
aggfunc='sum'
)
print(pivot)
# Melting (wide to long format)
wide_data = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Math': [90, 80, 70],
'Science': [95, 85, 75],
'History': [85, 90, 80]
})
print("\nMelting from wide to long format:")
long_data = pd.melt(
wide_data,
id_vars=['Name'],
value_vars=['Math', 'Science', 'History'],
var_name='Subject',
value_name='Score'
)
print(long_data)
Combining datasets is a common requirement, and Pandas offers SQL-like join operations:
# Create two DataFrames
employees = pd.DataFrame({
'ID': [1, 2, 3, 4, 5],
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emma'],
'Department_ID': [101, 102, 101, 103, 102]
})
departments = pd.DataFrame({
'Department_ID': [101, 102, 103, 104],
'Department': ['HR', 'IT', 'Finance', 'Marketing'],
'Location': ['New York', 'San Francisco', 'Chicago', 'Boston']
})
# Inner join
print("Inner join:")
inner_join = employees.merge(departments, on='Department_ID')
print(inner_join)
# Left join
print("\nLeft join:")
left_join = employees.merge(departments, on='Department_ID', how='left')
print(left_join)
# Right join
print("\nRight join:")
right_join = employees.merge(departments, on='Department_ID', how='right')
print(right_join)
# Full outer join
print("\nFull outer join:")
outer_join = employees.merge(departments, on='Department_ID', how='outer')
print(outer_join)
One of Pandas’ standout features is its robust support for time series data analysis:
# Create a time series
dates = pd.date_range(start='2023-01-01', periods=12, freq='M')
values = np.random.normal(loc=100, scale=10, size=12).cumsum()
time_series = pd.Series(values, index=dates)
print("Time series data:")
print(time_series)
# Resampling
print("\nMonthly to quarterly resampling (mean):")
quarterly = time_series.resample('Q').mean()
print(quarterly)
# Date functionality
print("\nExtract date components:")
df_dates = pd.DataFrame({
'Date': pd.date_range(start='2023-01-01', periods=12, freq='M')
})
df_dates['Year'] = df_dates['Date'].dt.year
df_dates['Month'] = df_dates['Date'].dt.month
df_dates['Day'] = df_dates['Date'].dt.day
df_dates['Weekday'] = df_dates['Date'].dt.day_name()
print(df_dates.head())
# Rolling windows
print("\nRolling average (3-month window):")
rolling_avg = time_series.rolling(window=3).mean()
print(rolling_avg)
# Plot time series
plt.figure(figsize=(12, 6))
time_series.plot(label='Original')
rolling_avg.plot(label='3-month Rolling Average')
plt.title('Time Series Analysis with Pandas')
plt.legend()
plt.savefig('time_series_analysis.png')
While not primarily a visualization library, Pandas offers convenient plotting capabilities through its integration with Matplotlib:
# Basic plotting
plt.figure(figsize=(15, 10))
# Subplot 1: Bar chart of department counts
plt.subplot(2, 2, 1)
df['Department'].value_counts().plot(kind='bar')
plt.title('Count by Department')
# Subplot 2: Scatter plot of Age vs. Salary
plt.subplot(2, 2, 2)
plt.scatter(df['Age'], df['Salary'])
plt.title('Age vs. Salary')
plt.xlabel('Age')
plt.ylabel('Salary')
# Subplot 3: Box plot of Salary by Department
plt.subplot(2, 2, 3)
df.boxplot(column='Salary', by='Department')
plt.title('Salary Distribution by Department')
# Subplot 4: Line plot of time series
plt.subplot(2, 2, 4)
time_series.plot()
plt.title('Time Series Plot')
plt.tight_layout()
plt.savefig('pandas_visualization.png')
Beyond the basics, Pandas offers advanced capabilities that can handle complex data analysis scenarios:
# Apply a function to each column
def normalize(column):
return (column - column.mean()) / column.std()
normalized_df = df[['Age', 'Salary']].apply(normalize)
print("Normalized data:")
print(normalized_df.head())
# Apply element-wise function with applymap
def format_value(x):
if isinstance(x, (int, float)):
return f"{x:.2f}"
return str(x)
formatted_df = df.applymap(format_value)
print("\nFormatted DataFrame:")
print(formatted_df.head())
# Define custom aggregation functions
def range_diff(x):
return x.max() - x.min()
def pct_change(x):
return (x.max() - x.min()) / x.min() * 100 if x.min() != 0 else 0
# Apply custom aggregations
custom_agg = df.groupby('Department').agg({
'Age': ['mean', range_diff],
'Salary': ['mean', 'median', range_diff, pct_change]
})
print("Custom aggregations:")
print(custom_agg)
# Convert to categorical for efficiency
df['Department'] = df['Department'].astype('category')
print("Memory usage with categories:")
print(df.memory_usage())
# Create an ordered category
performance = pd.Series(['Good', 'Excellent', 'Poor', 'Good', 'Excellent'])
performance = performance.astype(pd.CategoricalDtype(
categories=['Poor', 'Good', 'Excellent'],
ordered=True
))
print("\nOrdered categorical data:")
print(performance)
print(f"'Good' > 'Poor': {performance[1] > performance[2]}")
# Generate mock stock data
np.random.seed(42)
dates = pd.date_range('2022-01-01', '2022-12-31', freq='B') # Business days
stocks = ['AAPL', 'GOOG', 'MSFT', 'AMZN']
stock_data = pd.DataFrame(
np.random.normal(0, 1, (len(dates), len(stocks))).cumsum(axis=0) + 100,
index=dates,
columns=stocks
)
# Calculate daily returns
returns = stock_data.pct_change().dropna()
# Portfolio analysis
print("Stock correlation matrix:")
correlation = returns.corr()
print(correlation)
# Calculate rolling volatility (20-day window)
volatility = returns.rolling(window=20).std() * (252 ** 0.5) # Annualized
print("\nAverage annualized volatility:")
print(volatility.mean())
# Plot stock prices
plt.figure(figsize=(12, 6))
stock_data.plot()
plt.title('Stock Price Simulation')
plt.ylabel('Price')
plt.savefig('stock_analysis.png')
# Generate mock customer transaction data
np.random.seed(42)
n_customers = 1000
customer_ids = [f'CUST_{i:04d}' for i in range(n_customers)]
transaction_dates = pd.date_range('2022-01-01', '2022-12-31')
transactions = pd.DataFrame({
'customer_id': np.random.choice(customer_ids, size=5000),
'transaction_date': np.random.choice(transaction_dates, size=5000),
'amount': np.random.normal(100, 50, size=5000).round(2),
'product_category': np.random.choice(['Electronics', 'Clothing', 'Food', 'Books'], size=5000)
})
# Customer purchase frequency
purchase_frequency = transactions.groupby('customer_id').size().reset_index(name='transaction_count')
print("Purchase frequency statistics:")
print(purchase_frequency['transaction_count'].describe())
# Average purchase amount by customer
avg_purchase = transactions.groupby('customer_id')['amount'].mean().reset_index(name='avg_amount')
# Merge frequency and average amount
customer_metrics = purchase_frequency.merge(avg_purchase, on='customer_id')
# Define customer segments
def segment_customer(row):
if row['transaction_count'] >= 7 and row['avg_amount'] >= 100:
return 'High Value'
elif row['transaction_count'] >= 7:
return 'Frequent'
elif row['avg_amount'] >= 100:
return 'Big Spender'
else:
return 'Regular'
customer_metrics['segment'] = customer_metrics.apply(segment_customer, axis=1)
print("\nCustomer segments:")
print(customer_metrics['segment'].value_counts())
# Analyzing product category preferences by segment
segment_preferences = transactions.merge(customer_metrics[['customer_id', 'segment']], on='customer_id')
category_by_segment = pd.crosstab(segment_preferences['segment'], segment_preferences['product_category'], normalize='index')
print("\nProduct category preferences by segment:")
print(category_by_segment)
# Measuring performance
import time
# Method 1: Using iterrows (slow)
start_time = time.time()
result1 = []
for index, row in df.iterrows():
result1.append(row['Age'] * 2)
time1 = time.time() - start_time
# Method 2: Vectorized operation (fast)
start_time = time.time()
result2 = df['Age'] * 2
time2 = time.time() - start_time
print(f"iterrows time: {time1:.6f} seconds")
print(f"Vectorized time: {time2:.6f} seconds")
print(f"Speedup factor: {time1/time2:.1f}x")
# Use efficient data types
print("\nBefore optimizing dtypes:")
print(df.memory_usage(deep=True))
optimized_df = df.copy()
optimized_df['Department'] = optimized_df['Department'].astype('category')
optimized_df['Name'] = optimized_df['Name'].astype('category')
print("\nAfter optimizing dtypes:")
print(optimized_df.memory_usage(deep=True))
# Reading data in chunks
chunk_size = 1000
chunks = []
# Simulate reading large file in chunks
for i in range(5):
# In real applications, this would be:
# chunk = pd.read_csv('large_file.csv', chunksize=chunk_size)
chunk = pd.DataFrame(np.random.randn(chunk_size, 4), columns=list('ABCD'))
# Process each chunk
chunk['positive'] = chunk['A'] > 0
chunks.append(chunk)
# Combine processed chunks
result = pd.concat(chunks)
print(f"Processed {len(result)} rows in chunks")
Pandas works seamlessly with a variety of other data science libraries, forming a powerful ecosystem:
# Integration with NumPy
numpy_array = df[['Age', 'Salary']].values
print("Converted to NumPy array:")
print(numpy_array[:3])
# Integration with Matplotlib (already shown in examples)
# Integration with scikit-learn
from sklearn.preprocessing import StandardScaler
from sklearn.cluster import KMeans
# Scale the data
scaler = StandardScaler()
scaled_data = scaler.fit_transform(df[['Age', 'Salary']])
# Apply K-means clustering
kmeans = KMeans(n_clusters=3, random_state=42)
df['Cluster'] = kmeans.fit_predict(scaled_data)
print("\nCluster distribution:")
print(df['Cluster'].value_counts())
# Plot clusters
plt.figure(figsize=(10, 6))
colors = ['red', 'green', 'blue']
for cluster in range(3):
cluster_data = df[df['Cluster'] == cluster]
plt.scatter(
cluster_data['Age'],
cluster_data['Salary'],
color=colors[cluster],
label=f'Cluster {cluster}'
)
plt.title('Employee Clusters')
plt.xlabel('Age')
plt.ylabel('Salary')
plt.legend()
plt.savefig('employee_clusters.png')
Pandas continues to be the backbone of data analysis in Python for several compelling reasons:
- Intuitive API: The DataFrame interface is intuitive and easy to learn, yet powerful enough for complex operations.
- Flexibility: Pandas can handle a wide variety of data formats and structures, from CSV files to SQL databases.
- Comprehensive functionality: From data cleaning to advanced analytics, Pandas provides a complete toolkit for data manipulation.
- Performance: While not the fastest for all operations, Pandas offers good performance for most data analysis tasks, especially with proper optimization.
- Integration: Pandas works seamlessly with other data science libraries, forming a cohesive ecosystem for end-to-end analytics.
Whether you’re cleaning messy datasets, conducting exploratory data analysis, building financial models, or preparing data for machine learning, Pandas provides the tools you need to work effectively with structured data in Python. As data continues to grow in importance across industries, mastery of Pandas remains one of the most valuable skills for data professionals.
#Pandas #DataScience #DataAnalysis #Python #DataManipulation #DataFrames #DataVisualization #TimeSeriesAnalysis #DataCleaning #PythonDataScience #DataWrangling #FinancialAnalysis #CustomerAnalytics #DataTransformation #NumPy #Matplotlib #DataMerging #DataFiltering #DataProcessing #DataEngineering