Go
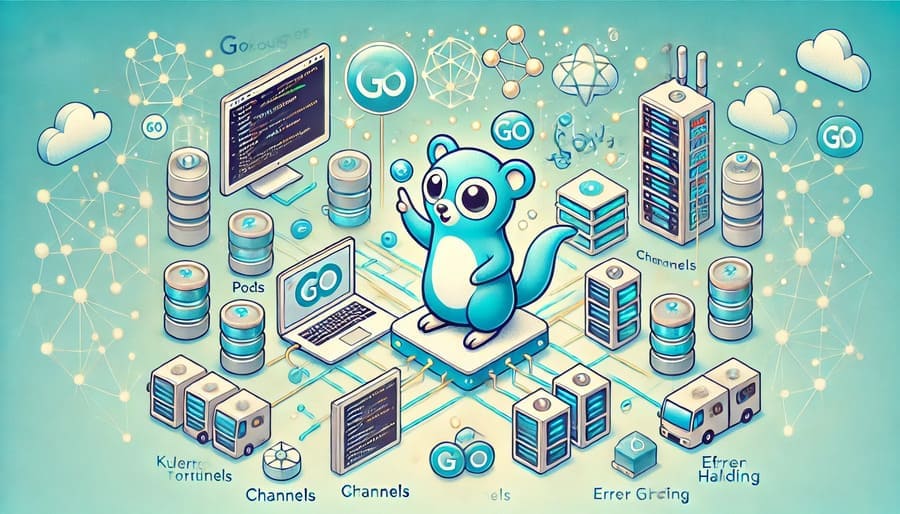
In today’s cloud-native world, building systems that can scale reliably across multiple machines is a fundamental challenge. Enter Go (or Golang)—a programming language specifically designed to excel in distributed environments. Created by Google engineers Robert Griesemer, Rob Pike, and Ken Thompson in 2009, Go has risen to prominence as the language of choice for cloud infrastructure, microservices, and distributed systems. This comprehensive guide explores why Go has become indispensable for modern distributed computing and how you can leverage its strengths in your projects.
Go emerged from Google’s need to solve real-world problems with existing programming languages. The creators identified several pain points in developing large-scale distributed systems:
- Long compilation times slowing down development cycles
- Complex dependency management creating “dependency hell”
- Lack of built-in concurrency making distributed programming difficult
- Verbose syntax reducing readability and increasing bugs
- Runtime inefficiencies causing performance bottlenecks
Go was designed specifically to address these challenges while maintaining simplicity, efficiency, and reliability—the perfect combination for distributed systems.
package main
import (
"fmt"
"net/http"
"time"
)
func main() {
// A simple web server
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, distributed world! The time is %s", time.Now())
})
fmt.Println("Server starting on port 8080...")
http.ListenAndServe(":8080", nil)
}
Go’s most revolutionary feature is its approach to concurrency through goroutines. Unlike traditional threads, goroutines are extremely lightweight (starting at just 2KB of memory) and managed by the Go runtime rather than the operating system. This allows Go programs to spawn thousands or even millions of concurrent operations efficiently.
func main() {
// Launch 100,000 concurrent operations
for i := 0; i < 100000; i++ {
go func(id int) {
// This function runs concurrently
processWork(id)
}(i)
}
// Wait for all to complete (simplified)
time.Sleep(2 * time.Second)
}
func processWork(id int) {
// Simulate work
time.Sleep(time.Millisecond * time.Duration(rand.Intn(100)))
fmt.Printf("Work unit %d completed\n", id)
}
This lightweight concurrency model is perfect for distributed systems where each connection, request, or data processing task can run independently without exhausting system resources.
Rather than relying on shared memory and locks, Go encourages communication through channels—type-safe conduits that allow goroutines to send and receive values. This approach, summarized by the Go proverb “Don’t communicate by sharing memory; share memory by communicating,” leads to safer concurrent code.
func main() {
// Create a channel
results := make(chan int)
// Launch 5 workers
for i := 0; i < 5; i++ {
go worker(i, results)
}
// Collect results
sum := 0
for i := 0; i < 5; i++ {
result := <-results
sum += result
}
fmt.Printf("Final result: %d\n", sum)
}
func worker(id int, results chan int) {
// Simulate work
time.Sleep(time.Millisecond * time.Duration(rand.Intn(1000)))
// Send result back through channel
results <- id * 100
}
Channels provide synchronization without explicit locks, reducing the risk of deadlocks and race conditions that plague distributed systems.
Go ships with a powerful standard library that includes comprehensive networking capabilities—essential for distributed systems that communicate over the network.
func startServer() {
http.HandleFunc("/api/data", func(w http.ResponseWriter, r *http.Request) {
data := map[string]interface{}{
"message": "Success",
"timestamp": time.Now().Unix(),
"status": "active",
}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(data)
})
// Start server with graceful shutdown
srv := &http.Server{
Addr: ":8080",
Handler: nil, // Use default router
}
go func() {
if err := srv.ListenAndServe(); err != nil && err != http.ErrServerClosed {
log.Fatalf("Server error: %v", err)
}
}()
// Set up graceful shutdown
quit := make(chan os.Signal, 1)
signal.Notify(quit, syscall.SIGINT, syscall.SIGTERM)
<-quit
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
srv.Shutdown(ctx)
log.Println("Server gracefully stopped")
}
The standard library’s net
and net/http
packages make it straightforward to create servers, handle connections, and implement various protocols without third-party dependencies.
Go’s compilation speed and ability to produce standalone static binaries make it ideal for distributed systems that need frequent updates and simple deployment:
- Quick feedback cycle: Fast compilation means developers can iterate quickly
- Single binary deployment: No dependencies to install on target machines
- Cross-compilation: Build for any platform from any platform
- Small container images: Ideal for microservices in containers
# Build a static binary for Linux from any OS
GOOS=linux GOARCH=amd64 go build -o myservice main.go
# Create a minimal Docker container
FROM scratch
COPY myservice /myservice
ENTRYPOINT ["/myservice"]
This simplicity dramatically reduces operational complexity in distributed environments where services might be deployed across many machines.
Go includes testing, benchmarking, and profiling tools in its standard library, essential for ensuring reliability in distributed systems:
// Simple test for a service handler
func TestDataHandler(t *testing.T) {
req := httptest.NewRequest("GET", "/api/data", nil)
w := httptest.NewRecorder()
dataHandler(w, req)
resp := w.Result()
if resp.StatusCode != http.StatusOK {
t.Errorf("Expected status OK; got %v", resp.StatusCode)
}
var data map[string]interface{}
if err := json.NewDecoder(resp.Body).Decode(&data); err != nil {
t.Fatal(err)
}
if data["message"] != "Success" {
t.Errorf("Expected message 'Success'; got %v", data["message"])
}
}
// Benchmark function
func BenchmarkDataProcessing(b *testing.B) {
for i := 0; i < b.N; i++ {
processData(testPayload)
}
}
The ability to thoroughly test and profile code leads to higher reliability—a critical requirement for distributed systems.
Kubernetes, the de facto standard for container orchestration, is written in Go. The language’s efficiency, reliability, and concurrency model were crucial factors in its selection. Kubernetes components run as distributed services, coordinating to manage containerized applications across clusters of machines.
// Simplified example inspired by Kubernetes controller pattern
func reconcileLoop(client *kubernetes.Clientset, namespace string) {
for {
// Get current state
pods, err := client.CoreV1().Pods(namespace).List(context.TODO(), metav1.ListOptions{})
if err != nil {
log.Printf("Error listing pods: %v", err)
time.Sleep(5 * time.Second)
continue
}
// Check desired state and reconcile
for _, pod := range pods.Items {
if needsReconciliation(pod) {
go reconcilePod(client, pod)
}
}
time.Sleep(10 * time.Second)
}
}
func reconcilePod(client *kubernetes.Clientset, pod v1.Pod) {
// Apply changes to reconcile the pod with desired state
// ...
}
Many modern distributed databases like CockroachDB, InfluxDB, and Dgraph are implemented in Go. The language’s performance characteristics and concurrency model make it well-suited for implementing consensus algorithms, data replication, and distributed query processing.
// Simplified example of a distributed consensus implementation
type ConsensusNode struct {
id string
state *State
peers []string
proposals chan Proposal
votes map[string]map[string]bool
mu sync.RWMutex
}
func (n *ConsensusNode) Start() {
// Start accepting proposals
go func() {
for proposal := range n.proposals {
go n.handleProposal(proposal)
}
}()
// Start heartbeat to peers
go n.heartbeatLoop()
}
func (n *ConsensusNode) handleProposal(p Proposal) {
// Send to all peers and collect votes
votes := make(chan Vote)
for _, peer := range n.peers {
go func(peerId string) {
vote := requestVote(peerId, p)
votes <- vote
}(peer)
}
// Collect votes with timeout
accepted := 1 // Count self vote
timer := time.NewTimer(5 * time.Second)
for i := 0; i < len(n.peers); i++ {
select {
case vote := <-votes:
if vote.Accepted {
accepted++
}
case <-timer.C:
break
}
}
// Check if we have majority
if accepted > (len(n.peers)+1)/2 {
n.commitProposal(p)
}
}
Go’s small memory footprint, fast startup time, and efficient HTTP handling make it ideal for microservices:
// A typical microservice in Go
package main
import (
"context"
"encoding/json"
"log"
"net/http"
"os"
"os/signal"
"syscall"
"time"
"github.com/gorilla/mux"
"github.com/prometheus/client_golang/prometheus/promhttp"
)
func main() {
// Create router
r := mux.NewRouter()
// API routes
r.HandleFunc("/api/users", getUsers).Methods("GET")
r.HandleFunc("/api/users/{id}", getUser).Methods("GET")
r.HandleFunc("/api/users", createUser).Methods("POST")
// Metrics endpoint
r.Handle("/metrics", promhttp.Handler())
// Health check
r.HandleFunc("/health", func(w http.ResponseWriter, r *http.Request) {
w.WriteHeader(http.StatusOK)
})
// Create server
srv := &http.Server{
Addr: ":8080",
Handler: r,
ReadTimeout: 15 * time.Second,
WriteTimeout: 15 * time.Second,
IdleTimeout: 60 * time.Second,
}
// Start server
go func() {
log.Println("Starting service on port 8080")
if err := srv.ListenAndServe(); err != nil && err != http.ErrServerClosed {
log.Fatalf("Server error: %v", err)
}
}()
// Graceful shutdown
c := make(chan os.Signal, 1)
signal.Notify(c, os.Interrupt, syscall.SIGTERM)
<-c
ctx, cancel := context.WithTimeout(context.Background(), 15*time.Second)
defer cancel()
log.Println("Shutting down server...")
srv.Shutdown(ctx)
log.Println("Server gracefully stopped")
}
func getUsers(w http.ResponseWriter, r *http.Request) {
// Fetch users from database or other service
users := []User{
{ID: "1", Name: "Alice", Email: "alice@example.com"},
{ID: "2", Name: "Bob", Email: "bob@example.com"},
}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(users)
}
// Other handler implementations...
Each microservice can be packaged as a small container, deployed independently, and scaled according to demand.
Message brokers play a critical role in distributed systems by decoupling services and ensuring reliable message delivery. NATS, a high-performance message broker, is written in Go and demonstrates how the language can be used to build efficient event distribution systems.
// Example of a simple message broker node in Go
type BrokerNode struct {
topics map[string][]*Subscription
topicsMu sync.RWMutex
clusterPeers []*PeerConnection
peersMu sync.RWMutex
}
func (b *BrokerNode) Subscribe(topic string, client *Client) *Subscription {
b.topicsMu.Lock()
defer b.topicsMu.Unlock()
sub := &Subscription{
Topic: topic,
Client: client,
ID: uuid.New().String(),
}
b.topics[topic] = append(b.topics[topic], sub)
return sub
}
func (b *BrokerNode) Publish(topic string, message []byte) {
// Local delivery
b.topicsMu.RLock()
subs, exists := b.topics[topic]
b.topicsMu.RUnlock()
if exists {
for _, sub := range subs {
go func(s *Subscription, msg []byte) {
s.Client.Send(s.Topic, msg)
}(sub, message)
}
}
// Propagate to peers
b.peersMu.RLock()
defer b.peersMu.RUnlock()
for _, peer := range b.clusterPeers {
if !peer.HasTopic(topic) {
continue
}
go peer.ForwardMessage(topic, message)
}
}
Circuit breakers prevent cascading failures in distributed systems by failing fast when a service is unavailable:
type CircuitBreaker struct {
name string
maxFailures int
resetTimeout time.Duration
failures int
lastFailure time.Time
state string // "closed", "open", "half-open"
mu sync.Mutex
}
func (cb *CircuitBreaker) Execute(command func() (interface{}, error)) (interface{}, error) {
cb.mu.Lock()
if cb.state == "open" {
// Check if reset timeout has elapsed
if time.Since(cb.lastFailure) > cb.resetTimeout {
cb.state = "half-open"
} else {
cb.mu.Unlock()
return nil, fmt.Errorf("circuit breaker %s is open", cb.name)
}
}
cb.mu.Unlock()
// Execute the command
result, err := command()
cb.mu.Lock()
defer cb.mu.Unlock()
if err != nil {
// Command failed
cb.failures++
cb.lastFailure = time.Now()
if cb.state == "half-open" || cb.failures >= cb.maxFailures {
cb.state = "open"
}
return nil, err
}
// Command succeeded
if cb.state == "half-open" {
// Reset on successful half-open call
cb.state = "closed"
cb.failures = 0
}
return result, nil
}
For observability in distributed systems, Go services can integrate with distributed tracing systems like Jaeger or Zipkin:
func userServiceHandler(w http.ResponseWriter, r *http.Request) {
// Extract tracing information from the request
spanCtx, _ := opentracing.GlobalTracer().Extract(
opentracing.HTTPHeaders,
opentracing.HTTPHeadersCarrier(r.Header),
)
// Create a span for this handler
span := opentracing.StartSpan(
"user_service.get_user",
ext.RPCServerOption(spanCtx),
)
defer span.Finish()
// Add useful tags
span.SetTag("http.method", r.Method)
span.SetTag("http.url", r.URL.Path)
// Create context with span
ctx := opentracing.ContextWithSpan(r.Context(), span)
// Call a dependency with the tracing context
user, err := getUserFromDatabase(ctx, r.URL.Query().Get("id"))
if err != nil {
span.SetTag("error", true)
span.LogFields(
log.String("event", "error"),
log.String("message", err.Error()),
)
http.Error(w, "User not found", http.StatusNotFound)
return
}
// Respond with the user data
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(user)
}
func getUserFromDatabase(ctx context.Context, userID string) (User, error) {
// Extract the parent span from context
span, ctx := opentracing.StartSpanFromContext(
ctx,
"database.get_user",
)
defer span.Finish()
span.SetTag("user.id", userID)
// Actual database call...
// ...
return user, nil
}
Go’s concurrency primitives make it easy to implement rate limiting to protect services from overload:
type RateLimiter struct {
rate int // Requests per second
capacity int // Burst capacity
tokens int
lastRefill time.Time
mu sync.Mutex
}
func NewRateLimiter(rate, capacity int) *RateLimiter {
return &RateLimiter{
rate: rate,
capacity: capacity,
tokens: capacity,
lastRefill: time.Now(),
}
}
func (l *RateLimiter) Allow() bool {
l.mu.Lock()
defer l.mu.Unlock()
// Refill tokens based on elapsed time
now := time.Now()
elapsed := now.Sub(l.lastRefill).Seconds()
newTokens := int(elapsed * float64(l.rate))
if newTokens > 0 {
l.tokens = min(l.capacity, l.tokens + newTokens)
l.lastRefill = now
}
// Check if we have tokens available
if l.tokens > 0 {
l.tokens--
return true
}
return false
}
// Use in an HTTP middleware
func rateLimitMiddleware(limiter *RateLimiter) func(http.Handler) http.Handler {
return func(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
if !limiter.Allow() {
http.Error(w, "Rate limit exceeded", http.StatusTooManyRequests)
return
}
next.ServeHTTP(w, r)
})
}
}
When parts of a distributed system fail, Go services can gracefully degrade functionality:
func getProductDetails(ctx context.Context, productID string) (Product, error) {
var product Product
var wg sync.WaitGroup
var mu sync.Mutex
// Get core product data - essential
wg.Add(1)
go func() {
defer wg.Done()
core, err := getProductCore(ctx, productID)
if err != nil {
log.Printf("Failed to get core product data: %v", err)
return
}
mu.Lock()
product.ID = core.ID
product.Name = core.Name
product.Price = core.Price
product.Available = core.Available
mu.Unlock()
}()
// Get product reviews - non-essential
wg.Add(1)
go func() {
defer wg.Done()
// Create context with shorter timeout for non-essential data
reviewCtx, cancel := context.WithTimeout(ctx, 300*time.Millisecond)
defer cancel()
reviews, err := getProductReviews(reviewCtx, productID)
if err != nil {
log.Printf("Failed to get product reviews: %v", err)
return
}
mu.Lock()
product.Reviews = reviews
mu.Unlock()
}()
// Get related products - non-essential
wg.Add(1)
go func() {
defer wg.Done()
// Create context with shorter timeout for non-essential data
relatedCtx, cancel := context.WithTimeout(ctx, 300*time.Millisecond)
defer cancel()
related, err := getRelatedProducts(relatedCtx, productID)
if err != nil {
log.Printf("Failed to get related products: %v", err)
return
}
mu.Lock()
product.RelatedProducts = related
mu.Unlock()
}()
// Wait for all goroutines to complete or timeout
done := make(chan struct{})
go func() {
wg.Wait()
close(done)
}()
select {
case <-done:
// All goroutines completed
case <-ctx.Done():
// Context timed out
return product, ctx.Err()
case <-time.After(1 * time.Second):
// Hard timeout
log.Printf("Timed out while getting full product details")
}
// Return whatever data we have, even if incomplete
if product.ID == "" {
return product, errors.New("failed to get core product data")
}
return product, nil
}
Error handling is critical in distributed systems where failures are expected:
func processOrder(ctx context.Context, orderID string) error {
// Validate the order
order, err := getOrder(ctx, orderID)
if err != nil {
// Add context to the error
return fmt.Errorf("failed to get order %s: %w", orderID, err)
}
// Process payment
err = processPayment(ctx, order)
if err != nil {
// Check for specific error types
if errors.Is(err, ErrInsufficientFunds) {
// Handle specific error case
notifyCustomerAboutPaymentIssue(order.CustomerID)
return fmt.Errorf("insufficient funds for order %s: %w", orderID, err)
}
return fmt.Errorf("payment processing failed for order %s: %w", orderID, err)
}
// Update inventory
err = updateInventory(ctx, order.Items)
if err != nil {
// Attempt to rollback payment
rollbackErr := rollbackPayment(ctx, order.PaymentID)
if rollbackErr != nil {
// Now we have two errors to report
return fmt.Errorf("inventory update failed for order %s and payment rollback also failed: %v, original error: %w",
orderID, rollbackErr, err)
}
return fmt.Errorf("inventory update failed for order %s (payment rolled back): %w", orderID, err)
}
return nil
}
The context
package is essential for managing request lifecycles, cancellation, and deadlines across service boundaries:
func handleRequest(w http.ResponseWriter, r *http.Request) {
// Create a timeout context
ctx, cancel := context.WithTimeout(r.Context(), 5*time.Second)
defer cancel()
// Add request ID to context for tracing
requestID := r.Header.Get("X-Request-ID")
if requestID == "" {
requestID = uuid.New().String()
}
ctx = context.WithValue(ctx, requestIDKey, requestID)
// Process the request with context
result, err := processWithContext(ctx)
if err != nil {
if errors.Is(err, context.DeadlineExceeded) {
w.WriteHeader(http.StatusGatewayTimeout)
fmt.Fprintf(w, "Request timed out")
return
}
// Handle other errors
w.WriteHeader(http.StatusInternalServerError)
fmt.Fprintf(w, "Error: %v", err)
return
}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(result)
}
func processWithContext(ctx context.Context) (Result, error) {
// Check for cancellation before expensive operation
if ctx.Err() != nil {
return Result{}, ctx.Err()
}
// Get request ID from context
requestID := ctx.Value(requestIDKey).(string)
// Call downstream service with context
return callService(ctx, requestID)
}
func callService(ctx context.Context, requestID string) (Result, error) {
req, err := http.NewRequestWithContext(ctx, "GET", "http://api.example.com/data", nil)
if err != nil {
return Result{}, err
}
// Propagate request ID
req.Header.Set("X-Request-ID", requestID)
resp, err := http.DefaultClient.Do(req)
if err != nil {
return Result{}, err
}
defer resp.Body.Close()
// Process response
// ...
return result, nil
}
For high-performance services, reducing garbage collection pauses is crucial:
// Inefficient: creates a new slice for each request
func processRequestsInefficient(requests []Request) []Response {
responses := make([]Response, 0, len(requests))
for _, req := range requests {
// Process each request
resp := processRequest(req)
responses = append(responses, resp)
}
return responses
}
// Efficient: uses a pre-allocated slice and object pool
var responsePool = sync.Pool{
New: func() interface{} {
return &Response{}
},
}
func processRequestsEfficient(requests []Request, responses []Response) []Response {
// Ensure slice capacity
if cap(responses) < len(requests) {
responses = make([]Response, len(requests))
} else {
responses = responses[:len(requests)]
}
for i, req := range requests {
// Get a response object from the pool
resp := responsePool.Get().(*Response)
// Reset it
*resp = Response{}
// Process the request
processRequestInto(req, resp)
// Store in our result slice
responses[i] = *resp
// Return to pool
responsePool.Put(resp)
}
return responses
}
Go’s ecosystem has evolved with numerous libraries and tools specifically designed for distributed systems:
- Consul: For service discovery, configuration, and health checking
- etcd: Distributed key-value store for configuration and service discovery
- Prometheus: For metrics collection and alerting
- OpenTelemetry: For distributed tracing and metrics
- gRPC: High-performance RPC framework
- NATS: Lightweight messaging system
- Protocol Buffers: Efficient serialization
- Docker: For containerization
- Kubernetes: For container orchestration
- Istio: Service mesh for distributed systems
Go’s intentional design choices have positioned it as the go-to language for building reliable, efficient distributed systems. Its combination of simplicity, performance, and built-in concurrency makes it uniquely suited for the challenges of distributed computing.
For developers and organizations building distributed systems, Go offers:
- Productivity: Fast compilation, simple syntax, and clear error handling
- Performance: Efficient execution with minimal resource utilization
- Reliability: Strong type system and comprehensive standard library
- Scalability: Lightweight concurrency model for handling massive parallelism
- Maintainability: Readable code and straightforward dependency management
As distributed systems continue to grow in importance with the rise of cloud computing, microservices, and edge computing, Go’s role as the language of choice for this domain is likely to strengthen further. Whether you’re building a new microservice, a distributed database, or a container orchestration system, Go provides the ideal foundation for your distributed systems journey.
#Golang #DistributedSystems #Concurrency #Microservices #CloudNative #SoftwareEngineering #Goroutines #Kubernetes #DevOps #SystemsDesign #ScalableArchitecture #BackendDevelopment #CloudComputing #GoProgramming #Containers #Docker #RESTfulAPI #gRPC #HighPerformance #Reliability #ServerSideDevelopment