Scala
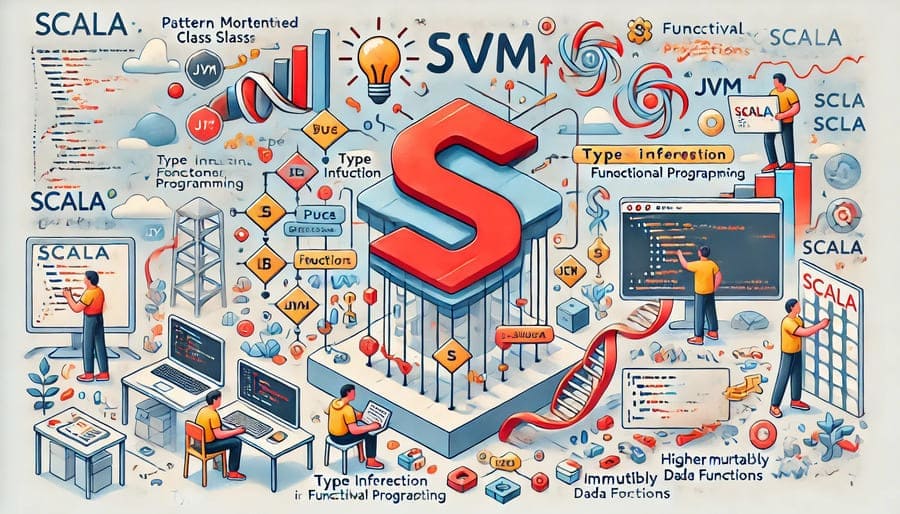
In the ever-evolving landscape of programming languages, Scala stands out as a unique and powerful fusion of object-oriented and functional programming paradigms. Built to run on the Java Virtual Machine (JVM), Scala offers developers the perfect blend of familiarity and innovation, enabling everything from web services and data analytics to distributed computing. This comprehensive guide explores what makes Scala special, its key features, and why it might be the ideal language for your next project.
Scala, whose name is a portmanteau of “Scalable Language,” was created by Martin Odersky and released in 2004. Odersky, who had previously worked on Java generics, sought to address several limitations he perceived in Java. His vision was to create a language that:
- Combined object-oriented and functional programming seamlessly
- Ran on the JVM for compatibility with existing systems
- Featured a more concise, expressive syntax than Java
- Embraced immutability and type safety by default
- Could scale from small scripts to massive enterprise applications
The result was Scala—a statically typed language that feels dynamic thanks to its powerful type inference, while providing the safety and optimization opportunities that static typing offers.
// A simple Scala program demonstrating its concise syntax
object HelloWorld {
def main(args: Array[String]): Unit = {
val names = List("Alice", "Bob", "Charlie", "David")
// Functional approach to transforming data
val greetings = names.map(name => s"Hello, $name!")
// Print each greeting
greetings.foreach(println)
// One-liner alternative
names.map(name => s"Hello, $name!").foreach(println)
}
}
Scala’s most distinctive feature is how it marries object-oriented programming (OOP) with functional programming (FP). Unlike languages that favor one paradigm over the other, Scala treats both approaches as first-class citizens:
// Object-oriented features
class Person(val name: String, val age: Int) {
def greet(): String = s"Hello, my name is $name and I am $age years old."
// Method overloading
def greet(timeOfDay: String): String =
s"$timeOfDay! My name is $name and I am $age years old."
}
// Functional features
object PersonOperations {
// Higher-order function taking a function as parameter
def processPersons(persons: List[Person],
transform: Person => String): List[String] = {
persons.map(transform)
}
// Pattern matching - another functional feature
def categorize(person: Person): String = person.age match {
case age if age < 18 => "Minor"
case age if age < 65 => "Adult"
case _ => "Senior"
}
}
// Using both paradigms together
val people = List(new Person("Alice", 25), new Person("Bob", 70))
val categories = PersonOperations.processPersons(people, PersonOperations.categorize)
Scala’s type system is sophisticated yet practical, offering features like:
- Type inference: Reducing boilerplate without sacrificing type safety
- Generics: With variance annotations for more precise type relationships
- Traits: Similar to interfaces but with the ability to include implementation
- Case classes: Immutable data containers with built-in equality and pattern matching support
- Type classes: Enabling ad-hoc polymorphism
// Type inference
val number = 42 // Scala infers this as Int
val text = "Hello" // Scala infers this as String
// Generics with variance
trait Reader[+A] {
def read(): A
}
trait Writer[-A] {
def write(value: A): Unit
}
// Case classes
case class Point(x: Int, y: Int) {
def distanceFromOrigin: Double = math.sqrt(x*x + y*y)
}
// Pattern matching with case classes
def describePoint(point: Point): String = point match {
case Point(0, 0) => "Origin"
case Point(x, 0) => s"On X-axis at $x"
case Point(0, y) => s"On Y-axis at $y"
case Point(x, y) if x == y => s"On diagonal at $x"
case Point(x, y) => s"At coordinates ($x, $y)"
}
Scala encourages immutable data structures and pure functions, which leads to code that is:
- Easier to reason about
- Safer in concurrent environments
- More testable
- Less prone to certain classes of bugs
// Immutable collections
val numbers = Vector(1, 2, 3, 4, 5)
val doubled = numbers.map(_ * 2) // Creates a new vector, doesn't modify original
// Pure function
def sum(numbers: List[Int]): Int = numbers.foldLeft(0)(_ + _)
// Pattern matching for immutable transformations
def increment(values: List[Int]): List[Int] = values match {
case Nil => Nil
case head :: tail => (head + 1) :: increment(tail)
}
Scala’s pattern matching goes beyond simple switch statements, providing a powerful mechanism for decomposing and handling data:
// Complex pattern matching example
def processInput(input: Any): String = input match {
// Match specific values
case 0 => "Zero"
case "" => "Empty string"
// Match types with conditions
case n: Int if n > 0 => s"Positive number: $n"
case s: String if s.nonEmpty => s"Non-empty string: $s"
// Destructuring case classes
case Person(name, age) if age >= 18 => s"Adult named $name"
// Matching collections
case head :: tail => s"List with head: $head and ${tail.length} more elements"
case List(a, b, _*) => s"List starting with $a and $b"
// Wildcard
case _ => "Something else"
}
As a JVM language, Scala offers exceptional interoperability with Java:
// Using Java libraries in Scala
import java.util.{Date, Calendar}
import java.text.SimpleDateFormat
def formattedCurrentDate(): String = {
val now = Calendar.getInstance()
val dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss")
dateFormat.format(now.getTime())
}
// Creating Scala collections from Java collections
import scala.jdk.CollectionConverters._
val javaList = new java.util.ArrayList[String]()
javaList.add("one")
javaList.add("two")
val scalaList = javaList.asScala.toList
Scala has become a dominant language in big data ecosystems, largely due to Apache Spark, which is written in Scala:
// Simple Spark data processing in Scala
import org.apache.spark.sql.{SparkSession, functions as F}
val spark = SparkSession.builder()
.appName("User Analysis")
.master("local[*]")
.getOrCreate()
// Read data
val users = spark.read.parquet("s3://data/users")
// Perform analysis
val activeUsersByCountry = users
.filter(F.col("active") === true)
.groupBy("country")
.agg(
F.count("id").as("active_users"),
F.avg("age").as("average_age")
)
.orderBy(F.desc("active_users"))
// Show results
activeUsersByCountry.show()
Scala offers several frameworks for building web applications, with Play Framework being among the most popular:
// Simple Play Framework controller
package controllers
import javax.inject._
import play.api.mvc._
@Singleton
class UserController @Inject()(cc: ControllerComponents,
userService: UserService) extends AbstractController(cc) {
def list() = Action { implicit request =>
val users = userService.getAllUsers()
Ok(views.html.users.list(users))
}
def show(id: Long) = Action { implicit request =>
userService.findById(id) match {
case Some(user) => Ok(views.html.users.detail(user))
case None => NotFound("User not found")
}
}
def create() = Action(parse.json) { request =>
request.body.validate[UserCreateRequest].fold(
errors => BadRequest(errors.toString),
userRequest => {
val newUser = userService.createUser(userRequest)
Created(views.html.users.detail(newUser))
}
)
}
}
Scala provides excellent tools for building concurrent and distributed systems:
// Actor-based concurrency with Akka
import akka.actor.{Actor, ActorSystem, Props}
// Define message types
case class ProcessTask(data: String)
case class TaskResult(result: String)
// Define an actor
class WorkerActor extends Actor {
def receive: Receive = {
case ProcessTask(data) =>
// Simulate processing
val result = data.toUpperCase
sender() ! TaskResult(result)
case _ => println("Unknown message")
}
}
// Using the actor system
val system = ActorSystem("TaskProcessingSystem")
val worker = system.actorOf(Props[WorkerActor], "worker")
// Send message to actor
implicit val timeout: akka.util.Timeout = akka.util.Timeout(5.seconds)
import akka.pattern.ask
import scala.concurrent.ExecutionContext.Implicits.global
val future = (worker ? ProcessTask("Hello, Akka!")).mapTo[TaskResult]
future.onComplete {
case Success(TaskResult(result)) => println(s"Task completed: $result")
case Failure(exception) => println(s"Task failed: ${exception.getMessage}")
}
Type classes provide a flexible way to add behavior to types without modifying their source code:
// Type class for conversion to JSON
trait JsonEncoder[T] {
def toJson(value: T): String
}
// Implementations for specific types
implicit val stringEncoder: JsonEncoder[String] = new JsonEncoder[String] {
def toJson(value: String): String = s"\"$value\""
}
implicit val intEncoder: JsonEncoder[Int] = new JsonEncoder[Int] {
def toJson(value: Int): String = value.toString
}
// Type class for lists, using other type class instances
implicit def listEncoder[T](implicit encoder: JsonEncoder[T]): JsonEncoder[List[T]] =
new JsonEncoder[List[T]] {
def toJson(values: List[T]): String =
values.map(encoder.toJson).mkString("[", ",", "]")
}
// Method using the type class
def convertToJson[T](value: T)(implicit encoder: JsonEncoder[T]): String =
encoder.toJson(value)
// Usage
convertToJson("hello") // Returns: "hello"
convertToJson(42) // Returns: 42
convertToJson(List(1, 2, 3)) // Returns: [1,2,3]
Scala supports higher-kinded types, which allow for extremely generic abstractions:
// Higher-kinded type parameter (F[_])
trait Functor[F[_]] {
def map[A, B](fa: F[A])(f: A => B): F[B]
}
// Implementation for Option
implicit val optionFunctor: Functor[Option] = new Functor[Option] {
def map[A, B](fa: Option[A])(f: A => B): Option[B] = fa.map(f)
}
// Implementation for List
implicit val listFunctor: Functor[List] = new Functor[List] {
def map[A, B](fa: List[A])(f: A => B): List[B] = fa.map(f)
}
// Generic function using functors
def increment[F[_]](container: F[Int])(implicit functor: Functor[F]): F[Int] =
functor.map(container)(_ + 1)
// Usage
increment(Option(5)) // Returns: Some(6)
increment(List(1, 2, 3)) // Returns: List(2, 3, 4)
Scala’s for comprehensions provide a clean syntax for working with monadic structures:
// Simple for comprehension with Option
def getUserInfo(id: String): Option[UserInfo] = {
for {
user <- findUser(id)
address <- findAddress(user.addressId)
company <- findCompany(user.companyId)
} yield UserInfo(user.name, address.city, company.name)
}
// For comprehension with error handling using Either
def processOrder(orderId: String): Either[Error, OrderConfirmation] = {
for {
order <- findOrder(orderId).toRight(OrderNotFound(orderId))
customer <- findCustomer(order.customerId).toRight(CustomerNotFound(order.customerId))
inventory <- checkInventory(order.items).toRight(InventoryError("Insufficient inventory"))
payment <- processPayment(customer, order.total).toRight(PaymentError("Payment failed"))
shipment <- createShipment(order, customer.address).toRight(ShippingError("Shipping failed"))
} yield OrderConfirmation(order.id, shipment.trackingNumber, payment.transactionId)
}
Scala benefits from a rich ecosystem of libraries and frameworks:
- Akka: Actor-based concurrency and distributed systems
- Play Framework: Web application framework
- Apache Spark: Big data processing
- Cats & Scalaz: Functional programming abstractions
- ZIO: Type-safe, composable asynchronous and concurrent programming
- http4s: Typeful, functional HTTP server and client
- Slick: Database access layer
- ScalaTest & Specs2: Testing frameworks
If you’re interested in learning Scala, consider this progressive approach:
- Start with the basics: Learn Scala syntax, collections, and pattern matching
- Understand functional programming: Explore immutability, higher-order functions, and pure functions
- Dive into object-oriented features: Classes, traits, and inheritance in Scala
- Explore advanced type system features: Generics, type classes, and type-level programming
- Learn concurrency models: Future/Promise, Akka actors, and reactive streams
- Specialize in an application area: Web development, big data, or backend systems
- Expressiveness: Accomplish more with less code
- Type Safety: Catch errors at compile time
- Functional Programming: Immutability and composition
- JVM Compatibility: Access to the vast Java ecosystem
- Concurrency Support: Tools for building robust concurrent systems
- Big Data Ecosystem: First-class language for Apache Spark
- Learning Curve: Steeper than some languages due to powerful features
- Compilation Speed: Can be slower than Java (though improving)
- Community Size: Smaller than Java or Python
- Job Market: More specialized positions, though often higher-paying
Scala represents a thoughtful evolution of programming language design, bringing together the best aspects of object-oriented and functional paradigms. Its combination of type safety, expressiveness, and JVM compatibility makes it an excellent choice for building complex, scalable systems.
Whether you’re developing data processing pipelines, web services, or distributed systems, Scala offers a powerful toolkit that grows with your needs. As functional programming concepts continue to influence mainstream development, Scala’s ahead-of-the-curve adoption of these ideas positions it well for the future.
For developers looking to expand their programming horizons, Scala offers a rewarding journey into advanced concepts while remaining practical and production-ready. Its influence extends beyond its direct usage, as many of its innovations have inspired features in other languages.
As the programming world continues to evolve, Scala’s balance of pragmatism and innovation ensures it will remain a valuable tool in the modern developer’s arsenal.
#Scala #FunctionalProgramming #JVM #ScalaProgramming #BigData #ApacheSpark #SoftwareDevelopment #TypeSafety #ProgrammingLanguages #WebDevelopment #Akka #PlayFramework #PatternMatching #ObjectOrientedProgramming #JavaInteroperability #Concurrency #DistributedSystems #TypeSystem #DataEngineering #Immutability